How to Round Up a Number in Java
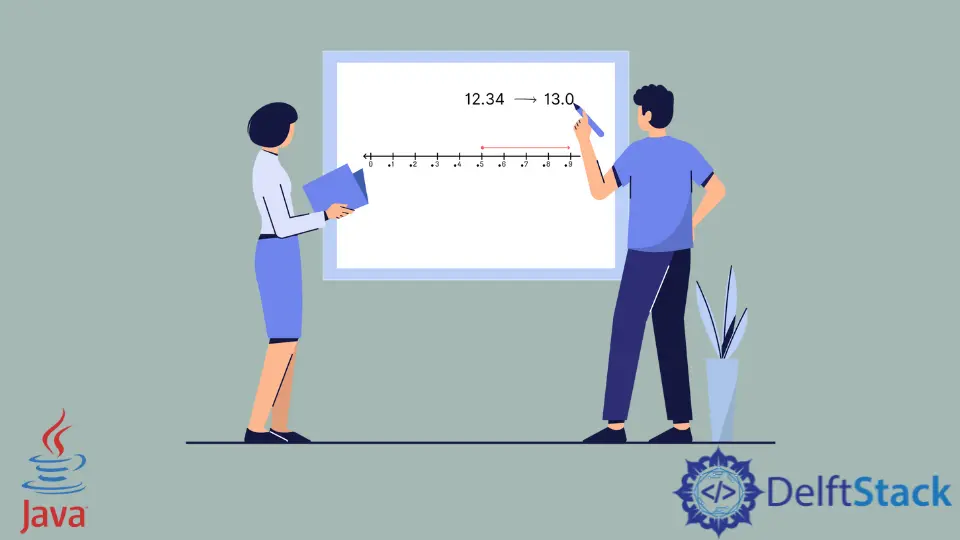
This article will introduce how to round up any number by using native classes in Java. We will use the ceil()
method of the Math
class in Java.
Math
has a few methods like Math.floor()
and Math.ceil()
to round numbers. Math.ceil()
is used to round up numbers; this is why we will use it.
Our goal is the round up the given number. Let’s take an example; if we have a number 0.2, then the rounded up number will be 1.
Math.ceil()
to Round Up Any Number to int
Math.ceil()
takes a double value, which it rounds up. In the below example, a
has the value 0.06 that rounds up to 1.0.
We want the result to be an int
, but when we use Math.ceil()
, we get the result as a double
; this is why we will cast the result to int
.
Example:
public class Main {
public static void main(String[] args) {
double a = 0.06;
int roundedNumA = (int) Math.ceil(a / 100);
System.out.println("Rounding up " + a + " we get " + roundedNumA);
}
}
Output:
Rounding up 0.6 we get 1
Math.ceil()
to Round Up a float
Number
We can round up float numbers using the Math.ceil()
method.
Example:
public class Main {
public static void main(String[] args) {
float a = 12.34f;
System.out.println("Rounding up " + a + " we get " + Math.ceil(a));
}
}
Output:
Rounding up 12.34 we get 13.0
Rupam Saini is an android developer, who also works sometimes as a web developer., He likes to read books and write about various things.
LinkedIn