The >> Operator in Java
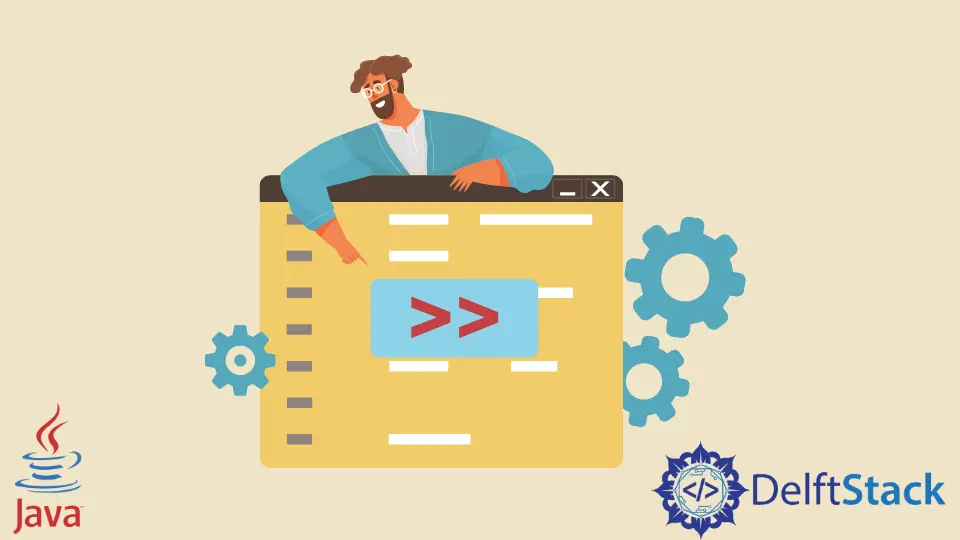
This guide will teach about the >>
operator in Java. To understand this concept, you need to be familiar with some lower-level computing concepts. For instance, bits, bytes, and whatnot. Let’s dive in.
the >>
Operator in Java
In Java, the >>
operator is the right shift operator. It shifts the given bit pattern to the right. For instance, if you are familiar with the bits, you know that shifters shift bits patterns.
Take a look at the following example.
Let
X=0110101;
X>>1
Shift the bytes by 1, and the result will be
0110101
0011010
Let
Y = 00111011
So when you do, x >> 2,
result in x = 00001110
If you look at the example, you will notice the shift of one bit. After the shift, the value 0110101
is changed to 0011010
.
You can visit this link to learn more about the shifters in bits.
The >>
operator works the same in Java. We’ll see how it operates and how you write a code for such a purpose. Take a look.
public static void main(String[] args) {
byte val = 100;
// binary of 100 is 1100100
val = (byte) (val >> 2); // shifting by two bits
System.out.println(val);
// after running the above code, the bits in binary will shift and it will look
// like this, 0011001 which is equal to number 25 in decimals.
}
The above code is self-explanatory. We are giving a byte value of 100
. The machine will work in binary numbers and read 100
as 1100100
.
Output:
25
After shifting it right by two bits, it will look like this 0011001
, equal to 25 in decimals. That’s the functionality of the >>
operator in Java.
Haider specializes in technical writing. He has a solid background in computer science that allows him to create engaging, original, and compelling technical tutorials. In his free time, he enjoys adding new skills to his repertoire and watching Netflix.
LinkedIn