How to Replace Character in String at Index in Java
-
Replace a Character in a String at Index in Java Using
substring()
-
Replace a Character in a String at Index in Java Using
StringBuilder()
-
Convert the String to an
Array
ofchars
to Replace a Character in a String at Index in Java
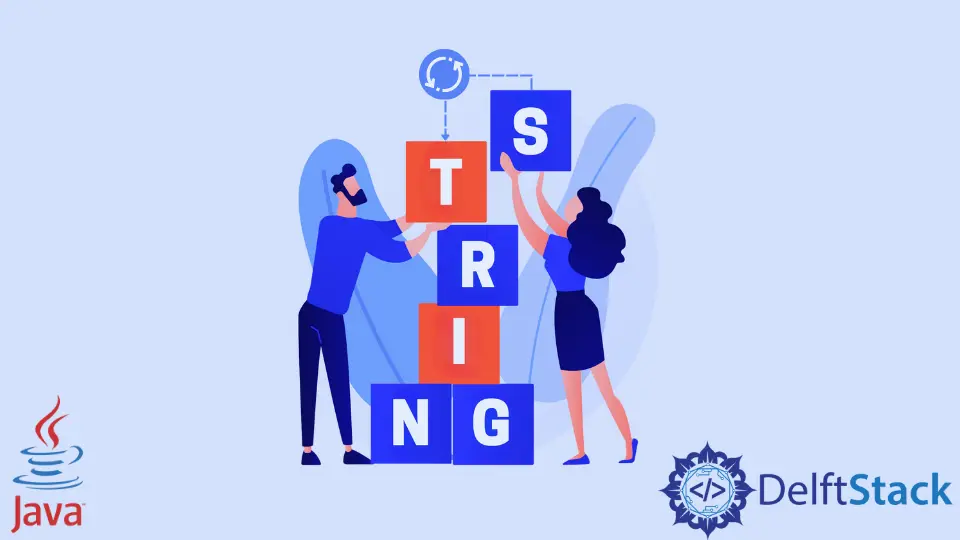
This article will introduce how we can replace a character in a string at a specific index in Java. We can use various ways to achieve our goal, which are mentioned in the following examples.
Replace a Character in a String at Index in Java Using substring()
In our first example, we have a string - ab
that has the character A
, which is an uppercase letter that does not fit the sentence, and we want to replace it with a lowercase character a
.
To replace it, we use the substring()
function of the String
class that takes a range or the string’s beginning index as an argument. Our target character is located at the position of index 8.
ab.substring(0, index)
returns the part of the string from 0 to 8th position. We concatenate this part of the string with our new character a
, and join the remaining string using ab.substring(index + 1)
.
public class ReplaceCharAtIndex {
public static void main(String[] args) {
String ab = "This is A String";
int index = 8;
String newString = ab.substring(0, index) + 'a' + ab.substring(index + 1);
System.out.println(newString);
}
}
Output:
This is a String
Replace a Character in a String at Index in Java Using StringBuilder()
We have the same string that we use in our previous example but will use StringBuilder()
to create a new string that is mutable because a normal string in Java is immutable. As the newString
is now modifiable, we can use its setChartAt()
method to set a new char
to a position or index.
newString.setCharAt(8, 'a')
sets the character a
at the 8th position.
public class ReplaceCharAtIndex {
public static void main(String[] args) {
String ab = "This is A String";
StringBuilder newString = new StringBuilder(ab);
newString.setCharAt(8, 'a');
System.out.println(newString);
}
}
Output:
This is a String
Convert the String to an Array
of chars
to Replace a Character in a String at Index in Java
The last method converts the string oldString
to an array of char
using toCharArray()
. We can replace any value in an array by specifying its position.
As we can see that there is a typo in oldString
where the word is misspelled, and we need to replace the character ( m
) with the character ( n
). We can replace our character at an index using charArray[index] = 'n'
.
At last, we have to convert the char[]
to a string
by using String.valueOf()
. The output shows that the character has been replaced.
public class ReplaceCharAtIndex {
public static void main(String[] args) {
String oldString = "This is an example strimg";
int index = 23;
char[] charArray = oldString.toCharArray();
charArray[index] = 'n';
String newString = String.valueOf(charArray);
System.out.println(newString);
}
}
Output:
This is an example string
Rupam Saini is an android developer, who also works sometimes as a web developer., He likes to read books and write about various things.
LinkedInRelated Article - Java Char
- How to Convert Int to Char in Java
- Char vs String in Java
- How to Initialize Char in Java
- How to Represent Empty Char in Java
- How to Convert Char to Uppercase/Lowercase in Java
- How to Check if a Character Is Alphanumeric in Java