How to Read File From Resources in Java
- Using ClassLoader to Read Files
- Reading Files Using getResourceAsStream
- Reading JSON Files from Resources
- Conclusion
- FAQ
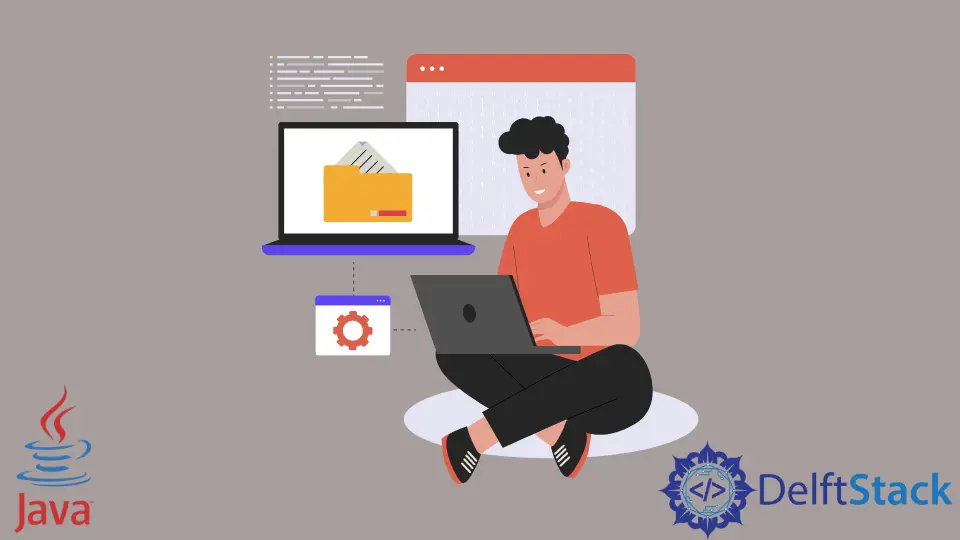
Reading files from resources in Java is a common task that developers often encounter, especially when working with properties files, configuration files, or any other static resources. Understanding how to effectively read these files can streamline your application development process and enhance your project’s organization.
In this article, we will explore various methods to read files from resources in Java, including using the ClassLoader and the getResourceAsStream method. Whether you are a beginner or an experienced developer, this guide will provide you with practical examples and insights to help you master file reading in Java.
Using ClassLoader to Read Files
One of the most straightforward methods to read files from resources in Java is by utilizing the ClassLoader. This method is particularly useful when you want to access files packaged within your JAR files or located in the classpath. The ClassLoader provides a way to load resources as streams, allowing you to read them easily.
Here’s a simple example of how to read a text file from resources using ClassLoader:
import java.io.BufferedReader;
import java.io.IOException;
import java.io.InputStream;
import java.io.InputStreamReader;
public class ResourceReader {
public static void main(String[] args) {
ClassLoader classLoader = ResourceReader.class.getClassLoader();
try (InputStream inputStream = classLoader.getResourceAsStream("example.txt");
BufferedReader reader = new BufferedReader(new InputStreamReader(inputStream))) {
String line;
while ((line = reader.readLine()) != null) {
System.out.println(line);
}
} catch (IOException e) {
e.printStackTrace();
}
}
}
Output:
This is an example line from the file.
Another line from the resource file.
In this example, we first obtain the ClassLoader instance using ResourceReader.class.getClassLoader()
. We then use getResourceAsStream("example.txt")
to acquire an InputStream for the specified resource file. By wrapping this InputStream in a BufferedReader, we can read the file line by line. This method is efficient and handles the resource cleanup automatically using the try-with-resources statement.
Reading Files Using getResourceAsStream
Another effective way to read files from resources in Java is to use the getResourceAsStream
method directly from the ClassLoader. This method is particularly helpful when you need to read files that are part of your project’s resources folder.
Here’s how you can implement this method:
import java.io.InputStream;
import java.nio.file.Files;
import java.nio.file.Paths;
public class ResourceReader {
public static void main(String[] args) {
try (InputStream inputStream = ResourceReader.class.getResourceAsStream("/example.txt")) {
String content = new String(inputStream.readAllBytes());
System.out.println(content);
} catch (IOException e) {
e.printStackTrace();
}
}
}
Output:
This is an example line from the file.
Another line from the resource file.
In this code snippet, we use getResourceAsStream("/example.txt")
to directly access the resource file. The leading slash indicates that the path is absolute, starting from the root of the classpath. After obtaining the InputStream, we read all bytes from it and convert them into a string. This approach is straightforward and ideal for reading smaller files where you want to load the entire content at once.
Reading JSON Files from Resources
If your project involves working with JSON files, you can easily read them from resources using similar methods. Reading JSON is essential for many applications, especially those that interact with APIs or require configuration data in a structured format.
Here’s an example of how to read a JSON file from resources:
import java.io.InputStream;
import java.nio.charset.StandardCharsets;
import com.fasterxml.jackson.databind.ObjectMapper;
public class JsonReader {
public static void main(String[] args) {
ObjectMapper objectMapper = new ObjectMapper();
try (InputStream inputStream = JsonReader.class.getResourceAsStream("/data.json")) {
MyData data = objectMapper.readValue(inputStream, MyData.class);
System.out.println(data);
} catch (IOException e) {
e.printStackTrace();
}
}
}
Output:
MyData{field1='value1', field2='value2'}
In this example, we utilize the Jackson library to parse the JSON file. We first create an instance of ObjectMapper
, then read the JSON file from resources using getResourceAsStream
. Finally, we map the JSON content to a custom Java class, MyData
, which represents the structure of the JSON data. This method is efficient and leverages powerful libraries for parsing complex data formats.
Conclusion
Reading files from resources in Java is a fundamental skill that every developer should master. By utilizing methods such as ClassLoader and getResourceAsStream
, you can efficiently access and manipulate files within your application. Whether you’re dealing with text files, configuration files, or JSON data, understanding these techniques will enhance your coding practices and improve your project organization. As you become more familiar with these methods, you’ll find it easier to integrate various resources into your Java applications.
FAQ
-
What is the difference between ClassLoader and getResourceAsStream?
ClassLoader is a Java class that loads classes and resources, while getResourceAsStream is a method used to retrieve a file as an InputStream from the classpath. -
Can I read files from outside the resources folder?
Yes, but you would need to provide the full file path instead of using the ClassLoader.
-
What types of files can I read from resources?
You can read any type of file, including text, JSON, XML, and properties files, as long as they are accessible from the classpath. -
How do I handle exceptions when reading files?
Use try-catch blocks to handle IOException and other potential exceptions that may arise during file operations. -
Is it necessary to close InputStreams?
Yes, it’s essential to close InputStreams to free up system resources. Using try-with-resources is a good practice for automatic resource management.
Haider specializes in technical writing. He has a solid background in computer science that allows him to create engaging, original, and compelling technical tutorials. In his free time, he enjoys adding new skills to his repertoire and watching Netflix.
LinkedIn