Difference Between X++ and ++X in Java
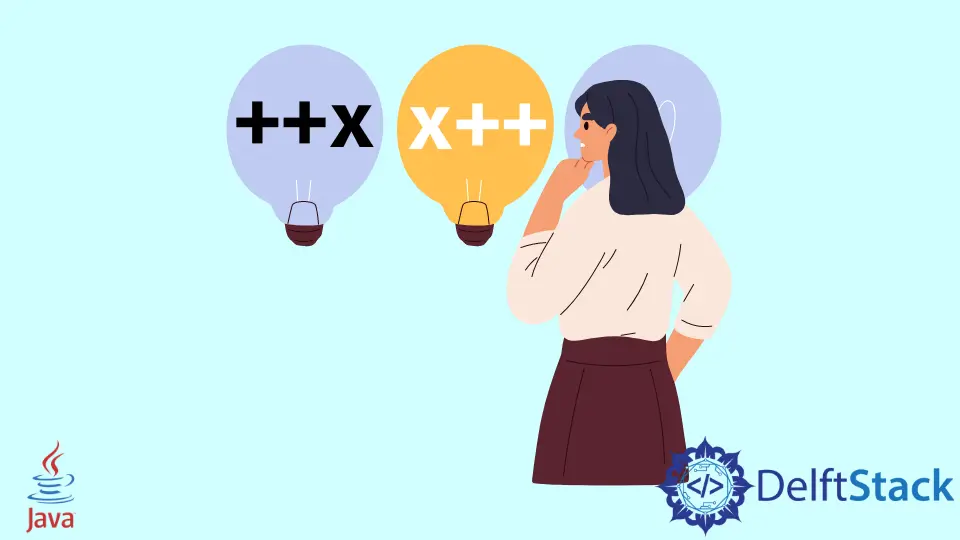
Many programmers face issues while understanding the difference between x++
and ++x
in Java. So, we are going to briefly the difference between these two expressions.
Prefix vs Postfix
Prefix = ++x
Postfix = x++
Both are used to increment value by one but in different manners. If the variable starts with ++
, then it is called pre-increment. If it comes after the variable, then it is called the post-increment operator.
Process to Increment in Java
In the post-increment method, we first use the current value and then increase the value by 1.
In the pre-increment method, the value is first incremented by 1 and then used in the Java statement.
Example
int x = 3;
int a = x++; // a = 3, x = 4
int b = ++a // b = 4, a = 4
In the first line, the x value is 3, which users assign. In second-line x post-increment method is used to give value to a. So, the first 3 is assigned to a and then incremented by 1, and the x value becomes 4. In the third line, b is assigned with a pre-increment value of a. It will increment a value by 1 and increase a value from 3 to 4. So, both a and b value becomes 4.
Java example:
import java.io.*;
class ABC {
public static void main(String[] args) {
// initialize i
int i = 0;
System.out.println("Post Increment");
System.out.println(i++);
int j = 0;
System.out.println("Pre Increment");
System.out.println(++j);
}
}
Output:
Post Increment
0
Pre Increment
1