Java or/and Logic
- Understanding Logical Operators in Java
- Using Logical Operators in Conditional Statements
- Combining Multiple Conditions with OR Operator
- Best Practices When Using Logical Operators
- Conclusion
- FAQ
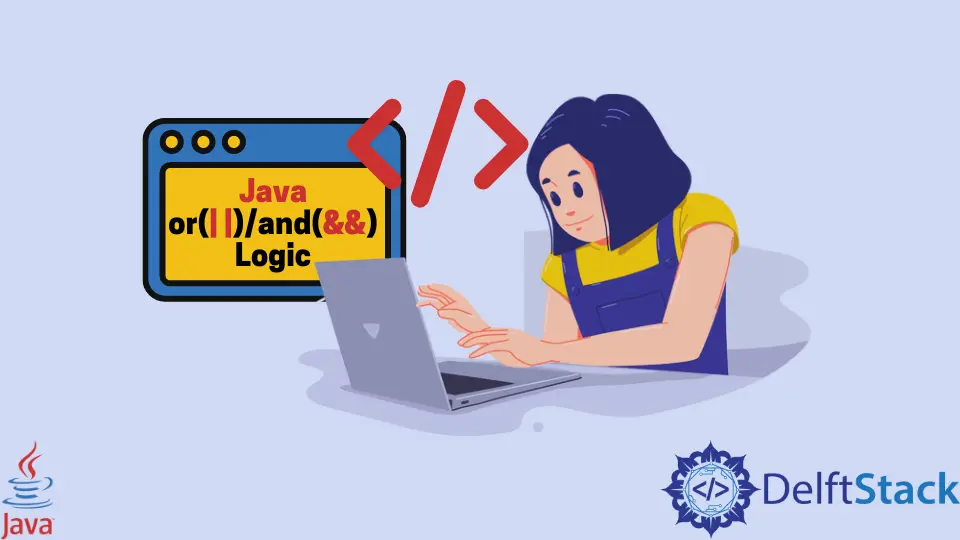
In the world of programming, logical operators play a crucial role in decision-making processes. When working with Java, understanding how to effectively utilize logical operators such as AND (&&) and OR (||) is essential for creating robust applications.
This article delves into the intricacies of Java’s logical operators, providing clear examples and explanations to help both beginners and seasoned developers grasp their functionality. Whether you’re developing complex algorithms or simple conditional statements, mastering these logical constructs will enhance your coding skills and improve your application’s performance. Let’s dive in and explore how to work with Java and its logical operators!
Understanding Logical Operators in Java
Logical operators are fundamental in programming, allowing you to combine multiple boolean expressions. In Java, the two primary logical operators are AND (&&) and OR (||). These operators evaluate expressions and return a boolean value based on the conditions specified.
The AND operator (&&) returns true only if both operands are true. On the other hand, the OR operator (||) returns true if at least one of the operands is true. Understanding these operators is vital for controlling the flow of your Java applications.
Here’s a simple example to illustrate their use:
boolean condition1 = true;
boolean condition2 = false;
boolean resultAND = condition1 && condition2;
boolean resultOR = condition1 || condition2;
The resultAND
variable will be false because one of the conditions is false. Conversely, resultOR
will be true since at least one condition is true.
Output:
resultAND: false
resultOR: true
This example highlights how logical operators can help in making decisions in your code. By using these operators, you can create more complex conditions and streamline your programming logic.
Using Logical Operators in Conditional Statements
One of the most common applications of logical operators is in conditional statements, such as if
statements. These operators allow you to combine multiple conditions to determine the flow of your program.
Consider the following example where we check user input against multiple criteria:
int age = 25;
boolean hasLicense = true;
if (age >= 18 && hasLicense) {
System.out.println("You are eligible to drive.");
} else {
System.out.println("You are not eligible to drive.");
}
In this code, we check if the user is at least 18 years old and has a valid driver’s license. The if
statement uses the AND operator (&&) to ensure both conditions are true before allowing the user to drive.
Output:
You are eligible to drive.
If either condition were false, the output would indicate that the user is not eligible. This demonstrates how logical operators can be utilized to enforce rules and validations effectively within your Java applications.
Combining Multiple Conditions with OR Operator
The OR operator (||) is particularly useful when you want to check if at least one condition is true. This can simplify your logic significantly. Let’s look at an example where we check if a user can access a restricted area based on their role or age.
String role = "guest";
int age = 17;
if (role.equals("admin") || age >= 18) {
System.out.println("Access granted.");
} else {
System.out.println("Access denied.");
}
In this scenario, the user is a guest and is underage. The if
statement checks if the user is either an admin or over 18. Since neither condition is met, access is denied.
Output:
Access denied.
This example illustrates how the OR operator can help create flexible conditions, enabling users with different roles or statuses to access certain features of your application.
Best Practices When Using Logical Operators
When working with logical operators in Java, it’s essential to follow best practices to ensure your code remains readable and maintainable. Here are some tips:
-
Keep Conditions Simple: Complex conditions can make your code hard to read. Break them down into simpler expressions if necessary.
-
Use Parentheses for Clarity: When combining multiple logical operators, use parentheses to make the order of evaluation clear. This can prevent logical errors.
-
Short-Circuit Evaluation: Java employs short-circuit evaluation with logical operators. For example, in an AND operation, if the first condition is false, the second condition is not evaluated. This can enhance performance and prevent unnecessary computations.
-
Testing and Validation: Always test your logical conditions thoroughly. Use different scenarios to ensure your logic holds under various inputs.
By adhering to these best practices, you can write cleaner, more efficient Java code that leverages logical operators effectively.
Conclusion
In conclusion, mastering logical operators such as AND (&&) and OR (||) in Java is essential for any programmer looking to enhance their coding skills. These operators enable you to create complex conditions and control the flow of your applications seamlessly. By understanding how to implement these logical constructs in conditional statements, you can build more robust and efficient programs. Remember to follow best practices to ensure your code remains clear and maintainable. With these tools at your disposal, you’re well on your way to becoming a proficient Java developer!
FAQ
-
What are logical operators in Java?
Logical operators are symbols used to combine boolean expressions, allowing for complex decision-making in programming. -
How does the AND operator work in Java?
The AND operator (&&) returns true only if both operands are true; otherwise, it returns false. -
When should I use the OR operator in my code?
Use the OR operator (||) when you want to check if at least one of multiple conditions is true. -
Can I combine AND and OR operators in Java?
Yes, you can combine AND and OR operators, but it’s important to use parentheses to clarify the order of evaluation. -
What are some best practices for using logical operators?
Keep conditions simple, use parentheses for clarity, and test your logic thoroughly to avoid errors.
Rashmi is a professional Software Developer with hands on over varied tech stack. She has been working on Java, Springboot, Microservices, Typescript, MySQL, Graphql and more. She loves to spread knowledge via her writings. She is keen taking up new things and adopt in her career.
LinkedIn