Optional ifPresent() in Java
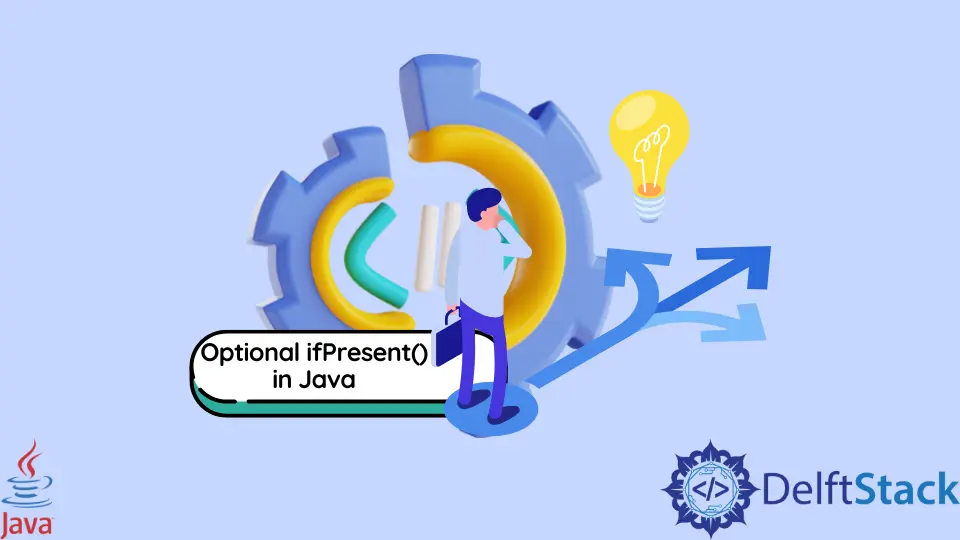
This tutorial will discuss the Optional class method named ifPresent()
in Java.
Optional Class Method ifPresent()
in Java
The Optional
class named ifPresent()
is an instance method mainly used to perform an action if the instance of the Class
contains a value. It is an implementation of the Consumer
interface.
To import this method, we need to import its package, java.util
, one of the most commonly used packages in every java program. The name of the class is Optional
.
Let’s now look at several examples of this method.
Use the ifPresent()
Method in Java
To understand how the ifPresent()
method works, we first put an empty Optional
and then we will put an Optional
with a value so that we can track the changes in the result.
The following snippet will show an example of the ifPresent()
method. In this example, we will set our Consumer
to empty.
Code Example:
// Importing necessary packages
import java.util.Optional;
import java.util.function.Consumer;
class Main {
public static void main(String args[]) {
Consumer<String> MyConsumer =
value -> System.out.println("\tConsumer Called: [" + value + "]"); // Declaring a Consumer.
Optional<String> MyOptional = Optional.empty(); // Declaring a Optional
MyOptional.ifPresent(MyConsumer); // Checking whether the Optional is empty or not.
}
}
We first declare a Consumer
, and then we create an Optional
and set it to empty by Optional.empty()
. After executing the above code, you will not get any output as the Optional
is empty.
Now we are going to put some value on the Optional
.
Code Example:
// Importing necessary packages
import java.util.Optional;
import java.util.function.Consumer;
public class Main {
public static void main(String args[]) {
Consumer<String> MyConsumer =
value -> System.out.println("\tConsumer Called: [" + value + "]"); // Declaring a Consumer.
Optional<String> MyOptional = Optional.of("This is a value."); // Declaring a Optional
MyOptional.ifPresent(MyConsumer); // Checking whether the Optional is empty or not.
}
}
Here we set a string value to the Optional
. If you run the updated code, you will get the below output.
Output:
Consumer Called: [This is a value.]
Aminul Is an Expert Technical Writer and Full-Stack Developer. He has hands-on working experience on numerous Developer Platforms and SAAS startups. He is highly skilled in numerous Programming languages and Frameworks. He can write professional technical articles like Reviews, Programming, Documentation, SOP, User manual, Whitepaper, etc.
LinkedIn