Java Not InstanceOf
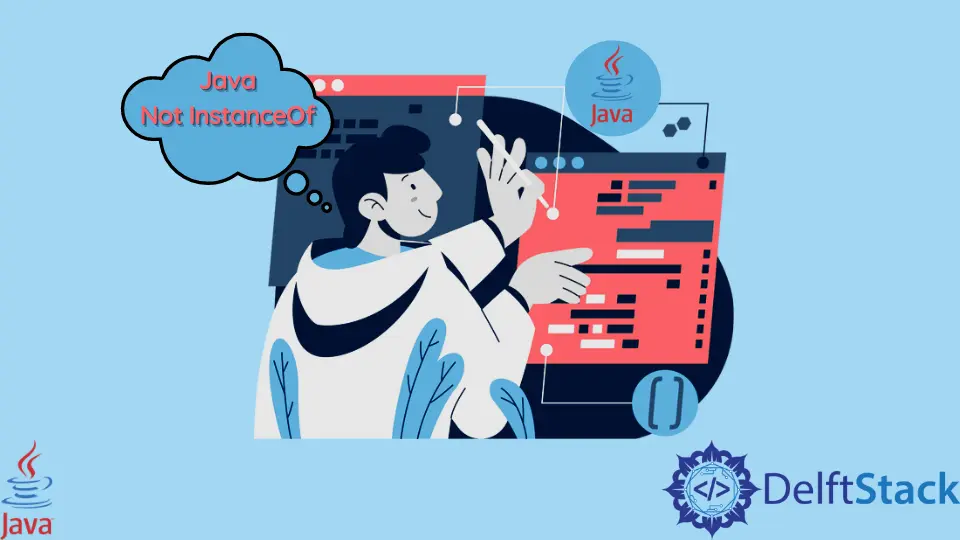
The InstanceOf
keyword checks if a reference variable contains a given object reference type. It returns Boolean types, so we can also negate them.
This tutorial demonstrates how to negate InstanceOf
or make Not InstanceOf
in Java.
Using the Not InstanceOf
in Java
The instanceof
returns a Boolean value, so negating it will return the false
value. Negating the InstanceOf
is done similarly to other negating in Java.
For example:
if (!(str instanceof String)) { /* ... */
}
or:
if (str instanceof String == false) { /* ... */
}
Let’s try a complete Java example to show the use of Not InstanceOf
in Java.
package delftstack;
class Delftstack_One {}
class Delftstack_Two extends Delftstack_One {}
public class Negate_InstanceOf {
public static void main(String[] args) {
Delftstack_Two Demo = new Delftstack_Two();
// A simple not instanceof using !
if (!(Demo instanceof Delftstack_Two)) {
System.out.println("Demo is Not the instance of Delftstack_Two");
} else {
System.out.println("Demo is the instance of Delftstack_Two");
}
// not instanceof using !
if (!(Demo instanceof Delftstack_One)) {
System.out.println("Demo is Not the instance of Delftstack_One");
} else {
System.out.println("Demo is the instance of Delftstack_One");
}
// instanceof returns true for all ancestors, and the object is the ancestor of all classes in
// Java
// not instance using == false
if ((Demo instanceof Object) == false) {
System.out.println("Demo is Not the instance of Object");
} else {
System.out.println("Demo is the instance of Object");
}
System.out.println(Demo instanceof Delftstack_One);
System.out.println(Demo instanceof Delftstack_Two);
System.out.println(Demo instanceof Object);
}
}
The code above creates two parent-child classes to show the usage of Not InstanceOf
. We used both methods described above.
Output:
Demo is the instance of Delftstack_Two
Demo is Not the instance of Delftstack_One
Demo is the instance of Object
false
true
true
Sheeraz is a Doctorate fellow in Computer Science at Northwestern Polytechnical University, Xian, China. He has 7 years of Software Development experience in AI, Web, Database, and Desktop technologies. He writes tutorials in Java, PHP, Python, GoLang, R, etc., to help beginners learn the field of Computer Science.
LinkedIn Facebook