How to Fix java.net.MalformedURLException: No Protocol Error in Java
-
the
java.net.MalformedURLException: no protocol
Error in Java -
Fix the
java.net.MalformedURLException: no protocol
Error
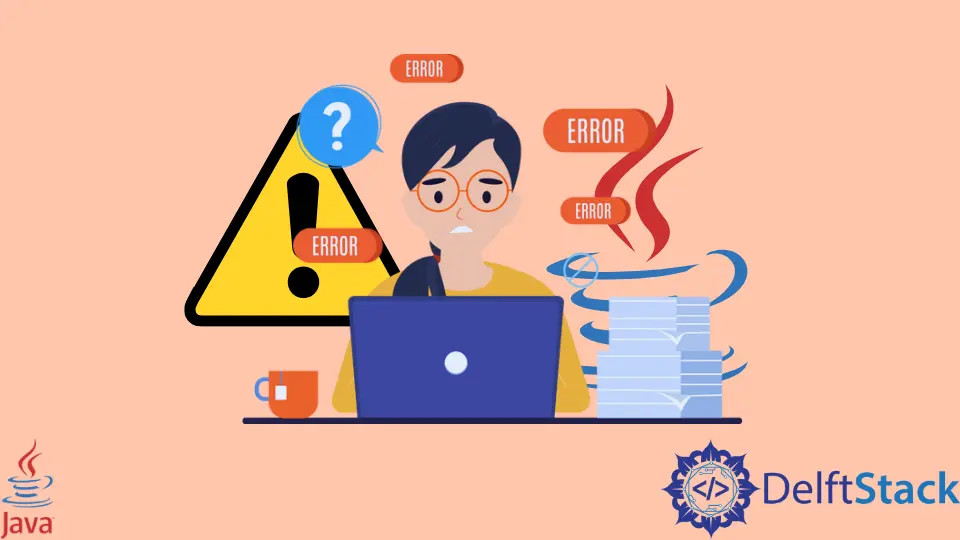
We are going to look into the error java.net.MalformedURLException: no protocol
in Java. Why does it occur, and how we can solve the error.
the java.net.MalformedURLException: no protocol
Error in Java
This java.net.MalformedURLException: no protocol
occurs when there’s an issue with our URL. This is client-server programming; by giving a request, we receive the response.
The request would be the URL that we will use to establish the connection between the client and the server. If the URL used is not working properly or not correctly used in the string, then we will receive the java.net.MalformedURLException: no protocol
error.
Fix the java.net.MalformedURLException: no protocol
Error
Sometimes, the programmer does not understand how to implement this client-server relation. Hence, this error is shown.
This error occurs only in two cases. The first case is when the user uses the string directly to parse by declaring it, and the second case is when the URL used is not correct.
In the second case, the incorrect URL can be easily resolved by correcting it. In the first case, we have to use the source of the string text and use it as a URL or use a method like StringReader
.
We will only use the StringReader
to properly implement and establish the connection. The StringReader
is a Reader
that reads the string as it is.
Let’s have a code example that will return the java.net.MalformedURLException: no protocol
error.
Code:
DocumentBuilderFactory DbuilderFac = DocumentBuilderFactory.newInstance();
DocumentBuilder Dbuilder = DbuilderFac.newDocumentBuilder();
String xml =
"/paste your XML here" Dbuilder.parse(xml); // we can's pass a direct XML string in it, because
// this particular function takes URL as input
As mentioned above, we must use the StringReader()
to fix the error. Take a look at the following code.
Code:
DocumentBuilderFactory DbuilderFac = DocumentBuilderFactory.newInstance();
DocumentBuilder Dbuilder = DbuilderFac.newDocumentBuilder();
String xml = "/paste your XML here" Dbuilder.parse(
new InputSource(new StringReader(xml))); // StringReader() is being used here
All you need to do is to use the InputSource
and the StringReader
to resolve the java.net.MalformedURLException: no protocol
error.
Haider specializes in technical writing. He has a solid background in computer science that allows him to create engaging, original, and compelling technical tutorials. In his free time, he enjoys adding new skills to his repertoire and watching Netflix.
LinkedInRelated Article - Java Error
- How to Fix the Error: Failed to Create the Java Virtual Machine
- How to Fix the Missing Server JVM Error in Java
- How to Fix the 'No Java Virtual Machine Was Found' Error in Eclipse
- How to Fix Javax.Net.SSL.SSLHandShakeException: Remote Host Closed Connection During Handshake
- How to Fix the Error: Failed to Create the Java Virtual Machine
- How to Fix Java.Lang.VerifyError: Bad Type on Operand Stack