How to Resolve Missing Method Body or Declare Abstract in Java
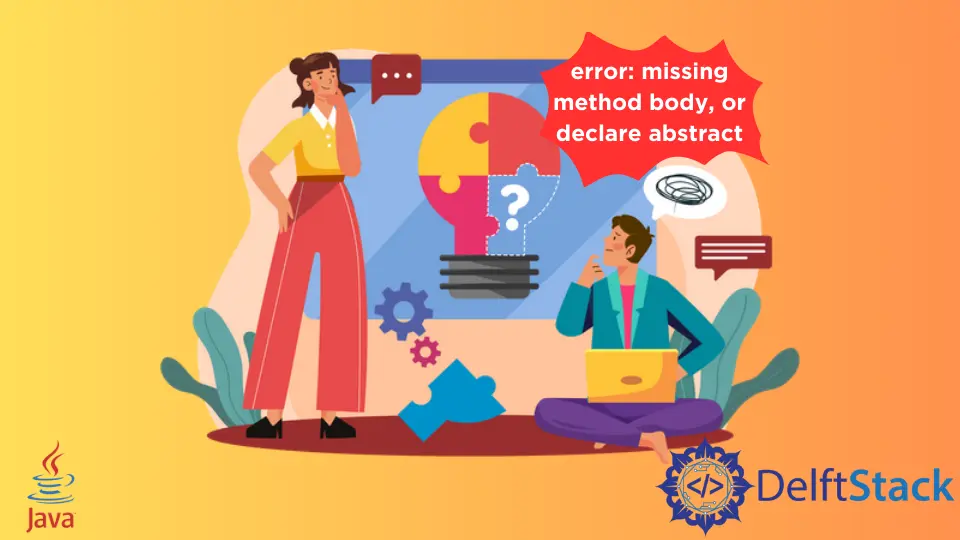
This tutorial discusses a compile-time error, missing method body, or declare abstract
. Here, we will go through three different steps.
First, we will understand a Java program to learn about the error. Second, highlight the possible causes of this error and finally have a solution to eradicate this compile time error.
Resolve the Missing Method Body or Declare Abstract Error in Java
Let’s start with the first step and understand the program causing this error.
Example Code (Main.java
class):
class Main {
public static void main(String[] param) {
HiWorld();
System.exit(0);
}
public static void HiWorld();
{ System.out.println("Hi World"); }
}
Here, we have a Main.java
class that contains a main()
method. Inside the main()
method, we call a method HiWorld()
and exit from the program.
Then, the HiWorld
method has a simple println
statement.
Everything seems fine, but this is causing the error saying missing method body, or declare abstract
. Why? It is because the compiler assumes that we have missed writing the function body or we want to declare this function as abstract
, but we forgot to write the abstract
keyword while defining the function.
Here, we are at the second step to learn about possible reasons causing this error. All possible reasons are listed below:
- First, re-check the code. We might have put the semi-colon (
;
) at the end of the function definition or somewhere else where it should not be. If so, remove that. - Another possibility is to forget to write the
abstract
keyword if we were intended to write anabstract
method. - Sometimes, we get this error if we have forgotten the
{
after themain()
method. - If you have
setters
in your program, then make sure thesetters
are not returning any value because usually, they do not have thereturn
type.
In our case, we have written the semi-colon (;
) at the end of the HiWorld()
method’s definition. So, removing it leads to the final step, which is a solution for this error.
Example Code (Main.java
):
class Main {
public static void main(String[] param) {
HiWorld();
System.exit(0);
}
public static void HiWorld() {
System.out.println("Hi World");
}
}
OUTPUT:
Hi World
Related Article - Java Error
- How to Fix the Error: Failed to Create the Java Virtual Machine
- How to Fix the Missing Server JVM Error in Java
- How to Fix the 'No Java Virtual Machine Was Found' Error in Eclipse
- How to Fix Javax.Net.SSL.SSLHandShakeException: Remote Host Closed Connection During Handshake
- How to Fix the Error: Failed to Create the Java Virtual Machine
- How to Fix Java.Lang.VerifyError: Bad Type on Operand Stack