How to Resolve the Java.Lang.NoClassDefFoundError: Org/Apache/Commons/Logging/LogFactory Error
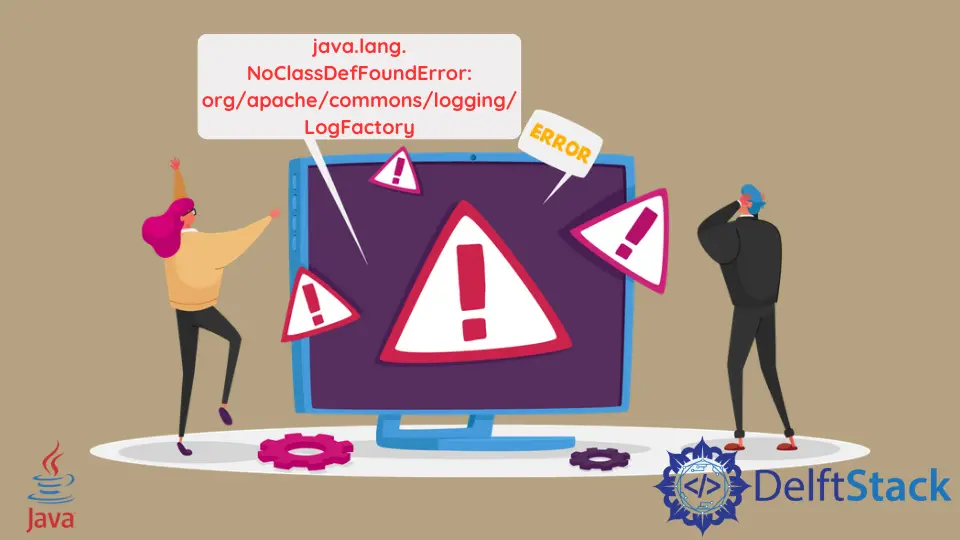
This tutorial demonstrates the java.lang.NoClassDefFoundError: org/apache/commons/logging/LogFactory
error in Java.
Resolve the java.lang.NoClassDefFoundError: org/apache/commons/logging/LogFactory
When using the Apache Commons and Spring, the error java.lang.NoClassDefFoundError: org/apache/commons/logging/LogFactory
can occur while injecting the dependencies. The error usually looks like this:
Exception in thread "main" java.lang.NoClassDefFoundError: org/apache/commons/logging/LogFactory
at org.springframework.context.support.AbstractApplicationContext.<init>(AbstractApplicationContext.java:119)
at org.springframework.context.support.AbstractXmlApplicationContext.<init>(AbstractXmlApplicationContext.java:55)
at org.springframework.context.support.ClassPathXmlApplicationContext.<init>(ClassPathXmlApplicationContext.java:77)
at org.springframework.context.support.ClassPathXmlApplicationContext.<init>(ClassPathXmlApplicationContext.java:65)
at org.springframework.context.support.ClassPathXmlApplicationContext.<init>(ClassPathXmlApplicationContext.java:56)
at com.client.StoryReader.main(StoryReader.java:15)
The reasons for this error are:
- The Apache Commons Logging jar files are not correct or not present.
- Commons Logging is not added to the dependencies.
Here are the solutions for the reasons above to solve this issue:
Add the Correct Apache Commons Logging Jars
If the Apache Commons Logging jars are not added to the build path of your project or the added files are not correct, then it will throw the java.lang.NoClassDefFoundError: org/apache/commons/logging/LogFactory
error.
Follow the steps below to solve this issue:
-
Download the Apache Commons Logging jars from here.
-
Add the jars to your project’s build path.
The above two steps should solve the error java.lang.NoClassDefFoundError: org/apache/commons/logging/LogFactory
. If you are using Maven or Spring, you need to add dependencies.
Add Commons Logging to Dependencies
If you are using Maven and Apache Commons-Logging is missing from the dependencies, it will throw the java.lang.NoClassDefFoundError: org/apache/commons/logging/LogFactory
error. Add the following dependency to pom.xml
:
<dependency>
<groupId>commons-logging</groupId>
<artifactId>commons-logging</artifactId>
<version>1.2</version>
</dependency>
Adding the above dependency will solve the problem for Maven.
In the case of using the Spring application, the developers sometimes exclude the Commons Logging but forget to insert another logging frame as the application requires the logging framework:
<dependency>
<groupId>org.springframework</groupId>
<artifactId>spring-webmvc</artifactId>
<version>${spring.version}</version>
<exclusions>
<exclusion>
<groupId>commons-logging</groupId>
<artifactId>commons-logging</artifactId>
</exclusion>
</exclusions>
</dependency>
The above pom.xml
is for the Spring application, and as we can see, the Commons-Logging is excluded; that is why it throws the java.lang.NoClassDefFoundError: org/apache/commons/logging/LogFactory
error.
To solve this issue, we must add another logging framework; most of the time, the developers use the SLF4j
logging. We also need to redirect the Spring’s logging using a bridge.
Add the following to pom.xml
of the Spring application:
<dependency>
<groupId>org.springframework</groupId>
<artifactId>spring-webmvc</artifactId>
<version>${spring.version}</version>
<exclusions>
<exclusion>
<groupId>commons-logging</groupId>
<artifactId>commons-logging</artifactId>
</exclusion>
</exclusions>
</dependency>
<!-- Redirect the Spring logging -->
<dependency>
<groupId>org.slf4j</groupId>
<artifactId>jcl-over-slf4j</artifactId>
<version>${jcl.slf4j.version}</version>
</dependency>
<dependency>
<groupId>ch.qos.logback</groupId>
<artifactId>logback-classic</artifactId>
<version>${logback.version}</version>
</dependency>
Following the above process for Maven and Spring will fix the java.lang.NoClassDefFoundError: org/apache/commons/logging/LogFactory
error.
Sheeraz is a Doctorate fellow in Computer Science at Northwestern Polytechnical University, Xian, China. He has 7 years of Software Development experience in AI, Web, Database, and Desktop technologies. He writes tutorials in Java, PHP, Python, GoLang, R, etc., to help beginners learn the field of Computer Science.
LinkedIn FacebookRelated Article - Java Error
- How to Fix the Error: Failed to Create the Java Virtual Machine
- How to Fix the Missing Server JVM Error in Java
- How to Fix the 'No Java Virtual Machine Was Found' Error in Eclipse
- How to Fix Javax.Net.SSL.SSLHandShakeException: Remote Host Closed Connection During Handshake
- How to Fix the Error: Failed to Create the Java Virtual Machine
- How to Fix Java.Lang.VerifyError: Bad Type on Operand Stack