Java Labels
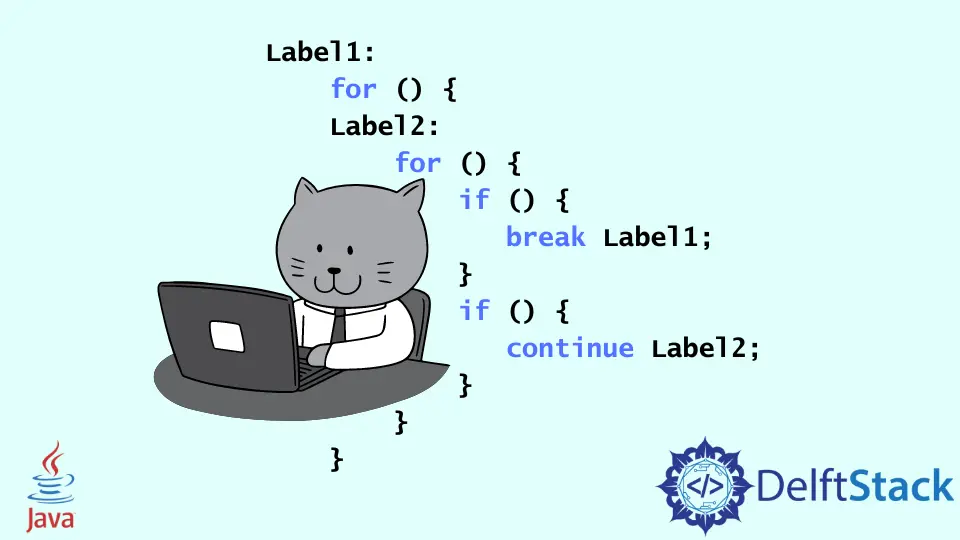
The concept of labels comes from the assembly language, but in Java, the labels are mainly used with break
and continue
statements used to control the flow of a program. This tutorial demonstrates how to use labels in Java.
Use Labels in a Single Loop in Java
The labels are used with break
and continue
statements to control the flow of a loop; let’s try an example for a single for
loop to see how labeled break
and continue
statements work.
See example:
package delftstack;
class Java_Label {
public static void main(String[] args) {
Demo_Label1:
for (int x = 0; x < 10; x++) {
if (x == 7) {
break Demo_Label1;
}
System.out.print(x + " ");
}
System.out.print("\n");
Demo_Label2:
for (int x = 0; x < 10; x++) {
if (x == 7) {
continue Demo_Label2;
}
System.out.print(x + " ");
}
}
}
We create the label outside the loop in which we want to use the label and then use it with break
or continue
statements.
Output:
0 1 2 3 4 5 6
0 1 2 3 4 5 6 8 9
Use Labels in a Nested Loops in Java
The actual use of labels is best for the nested loops because we can apply the break
or continue
statement to the loop of our wish; otherwise, by default, these statements are only applied to the loop where the statement is written.
But with labels, we can apply the continue
and break
statements on the loop of our choice.
See example:
package delftstack;
class Java_Label {
public static void main(String[] args) {
First_Loop:
for (int x = 0; x < 5; x++) {
Second_Loop:
for (int y = 0; y < 5; y++) {
if (x == 3) {
System.out.println("The outer Loop breaks from inside of inner loop at " + x);
break First_Loop;
}
if (y == 3) {
System.out.println("The inner loop is continued at " + y);
continue Second_Loop;
}
}
}
}
}
The code above shows the use of labels in Java. We can apply the break
or continue
statement on the first loop from the second loop.
Output:
The inner loop is continued at 3
The inner loop is continued at 3
The inner loop is continued at 3
The outer Loop breaks from inside of inner loop at 3
Sheeraz is a Doctorate fellow in Computer Science at Northwestern Polytechnical University, Xian, China. He has 7 years of Software Development experience in AI, Web, Database, and Desktop technologies. He writes tutorials in Java, PHP, Python, GoLang, R, etc., to help beginners learn the field of Computer Science.
LinkedIn Facebook