How to Convert Iterable to Stream in Java
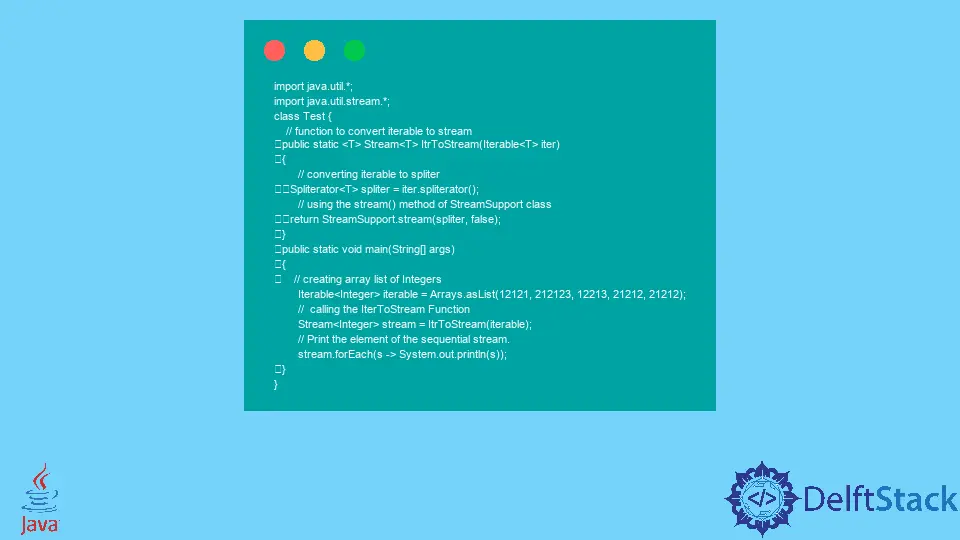
In Java, we can use the Iterator
to iterate through every collection element. The stream is a pipeline of objects from collections.
There can be two types of streams. One is parallel, and another is a sequential stream.
In this article, we will learn a method to convert the Iterable
to Stream
.
Use the StreamSupport.stream()
Method to Convert Iterable
to Stream
in Java
No method in the iterable
interface can directly convert iterable
to stream
. So, we must use the stream()
method of the StreamSupport
class.
Users can follow the syntax below to use the stream()
method.
Spliterator<T> spliter = iter.spliterator();
return StreamSupport.stream(spliter, isParallel);
Parameters:
spliter |
It is a spliterator of the iterator, which we have converted using the Iterable.spliterator() method. |
isParallel |
A Boolean value defines whether the stream is parallel or sequential. Users should pass the true Boolean value to get the parallel stream. |
In the example below, we have created the iterable of integers. We called the IterToStream()
function and passed the iterable as an argument that returns the stream of a particular iterable.
In the ItrToStream()
function, we are converting the iterable to spliterator
. After that, we used the StreamSupport.stream()
method to convert the spliterator
to stream.
At last, we are printing the stream.
import java.util.*;
import java.util.stream.*;
class Test {
// function to convert iterable to stream
public static <T> Stream<T> ItrToStream(Iterable<T> iter) {
// converting iterable to spliter
Spliterator<T> spliter = iter.spliterator();
// using the stream() method of StreamSupport class
return StreamSupport.stream(spliter, false);
}
public static void main(String[] args) {
// creating array list of Integers
Iterable<Integer> iterable = Arrays.asList(12121, 212123, 12213, 21212, 21212);
// calling the IterToStream Function
Stream<Integer> stream = ItrToStream(iterable);
// Print the element of the sequential stream.
stream.forEach(s -> System.out.println(s));
}
}
Output:
12121
212123
12213
21212
21212
We have successfully learned to convert iterable
to stream
in Java using the StreamSupport.stream()
method.