How to Handle FileNotFoundException in Java
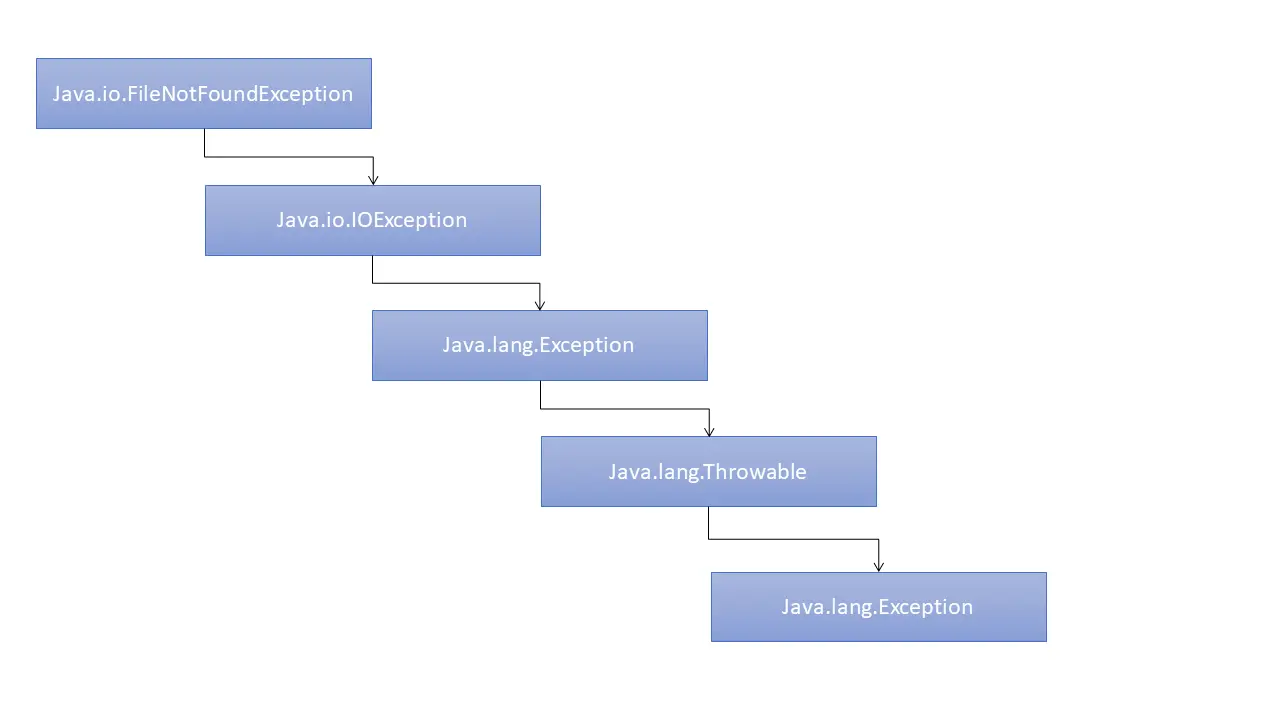
FileNotFoundException occurs when we are trying to access a file. It is a part of IO exceptions thrown by FileOutputStream, FileInputStream, and RandomAccessFile, and we can use try-catch
blocks to handle these exceptions.
This tutorial describes the FileNotFoundException and demonstrates how to handle FileNotFoundException.
the Java IO FileNotFoundException
The FileNotFoundException occurs when a file with a particular pathname does not exist, or the file with pathname exists, but we can access it for some reason. The FileNotFoundException extends the java.io.IOException, which extends java.lang.Exception, which is dealt with try-catch
blocks.
The picture below demonstrates the structure of FileNotFoundException
.
Let’s run an example to see the FileNotFoundException
:
package delftstack;
import java.io.File;
import java.util.*;
public class File_Not_Found_Exception {
public static void main(String[] args) throws Exception {
Scanner demo_file = new Scanner(new File("demo.txt"));
String demo_content = "";
demo_content = demo_file.nextLine();
}
}
The file demo.txt
doesn’t exist; the code will throw the FileNotFoundException.
Output:
Exception in thread "main" java.io.FileNotFoundException: demo.txt (The system cannot find the file specified)
at java.base/java.io.FileInputStream.open0(Native Method)
at java.base/java.io.FileInputStream.open(FileInputStream.java:216)
at java.base/java.io.FileInputStream.<init>(FileInputStream.java:157)
at java.base/java.util.Scanner.<init>(Scanner.java:639)
at delftstack.File_Not_Found_Exception.main(File_Not_Found_Exception.java:8)
Handle FileNotFoundException in Java
We can use try-catch
blocks to handle the FileNotFoundException in Java. The example below demonstrates the handling of FileNotFoundException:
package delftstack;
import java.io.BufferedReader;
import java.io.File;
import java.io.FileReader;
import java.io.IOException;
public class File_Not_Found_Exception {
private static final String demo_file = "demo.txt";
public static void main(String[] args) {
BufferedReader file_read = null;
try {
// Open the file to read the content
file_read = new BufferedReader(new FileReader(new File(demo_file)));
// Reading the file content
String file_line = null;
while ((file_line = file_read.readLine()) != null) System.out.println(file_line);
} catch (IOException e) {
System.err.println("FileNotFoundException was caught!");
e.printStackTrace();
} finally {
try {
file_read.close();
} catch (IOException e) {
System.err.println("FileNotFoundException was caught!");
e.printStackTrace();
}
}
}
}
The code above handles the exception. First, it tries to open and read the file, and if the file does not exist or is not permitted to open and read, it will throw the FileNotFoundException.
Output:
FileNotFoundException was caught!
java.io.FileNotFoundException: demo.txt (The system cannot find the file specified)
at java.base/java.io.FileInputStream.open0(Native Method)
at java.base/java.io.FileInputStream.open(FileInputStream.java:216)
at java.base/java.io.FileInputStream.<init>(FileInputStream.java:157)
at java.base/java.io.FileReader.<init>(FileReader.java:75)
at delftstack.File_Not_Found_Exception.main(File_Not_Found_Exception.java:16)
Exception in thread "main" java.lang.NullPointerException: Cannot invoke "java.io.BufferedReader.close()" because "file_read" is null
at delftstack.File_Not_Found_Exception.main(File_Not_Found_Exception.java:29)
Sheeraz is a Doctorate fellow in Computer Science at Northwestern Polytechnical University, Xian, China. He has 7 years of Software Development experience in AI, Web, Database, and Desktop technologies. He writes tutorials in Java, PHP, Python, GoLang, R, etc., to help beginners learn the field of Computer Science.
LinkedIn Facebook