Difference Between Integer and Int in Java
- What is Int in Java?
- What is Integer in Java?
- Key Differences Between Int and Integer
- When to Use Int and When to Use Integer
- Conclusion
- FAQ
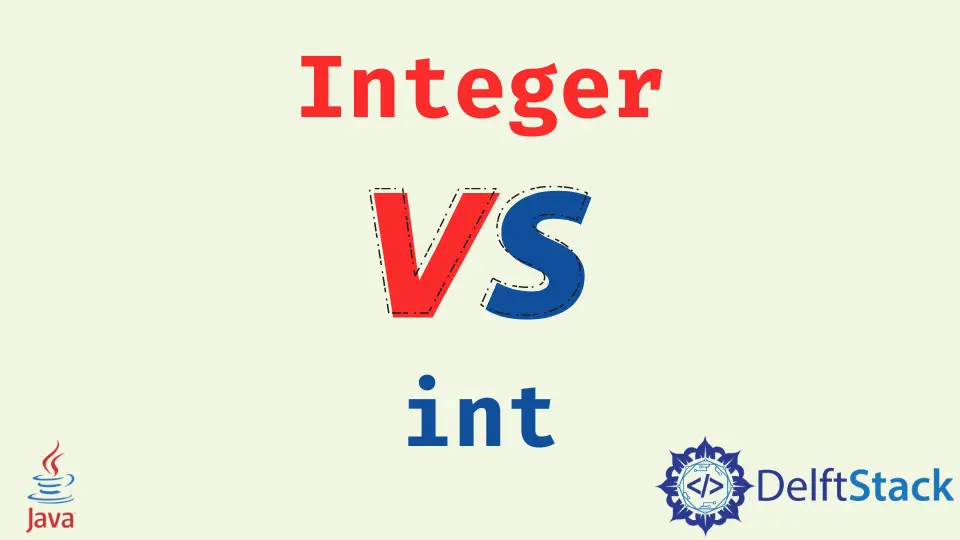
Java is a powerful programming language that offers a rich set of features for developers. Among these features are two commonly used data types: int
and Integer
. While they may seem similar at first glance, understanding the differences between them is crucial for effective programming in Java.
This article will explore the key distinctions between int
and Integer
, including their characteristics, uses, and implications for memory management. Whether you’re a beginner or an experienced developer, grasping these differences will enhance your coding skills and help you make better decisions in your Java projects.
What is Int in Java?
The int
data type in Java is a primitive data type that represents a 32-bit signed integer. It is one of the eight primitive types available in Java, making it efficient for storing numerical values. Being a primitive type, int
is stored directly in memory, which allows for faster access and manipulation.
Here’s a simple example of how to use int
in Java:
public class IntExample {
public static void main(String[] args) {
int number = 10;
System.out.println("The value of number is: " + number);
}
}
Output:
The value of number is: 10
In this snippet, we declare an int
variable named number
and assign it a value of 10. The System.out.println
statement then outputs the value. Since int
is a primitive type, it is stored in a straightforward manner in memory, which leads to efficient performance, especially in mathematical computations.
What is Integer in Java?
On the other hand, Integer
is a wrapper class in Java, part of the Java Collections Framework. It is used to encapsulate an int
value in an object. This is particularly useful when you need to store integer values in data structures that require objects, such as ArrayList
or HashMap
.
Here’s a simple example of how to use Integer
in Java:
import java.util.ArrayList;
public class IntegerExample {
public static void main(String[] args) {
Integer number = 10;
ArrayList<Integer> list = new ArrayList<>();
list.add(number);
System.out.println("The value in the list is: " + list.get(0));
}
}
Output:
The value in the list is: 10
In this code, we create an Integer
object and add it to an ArrayList
. The key point here is that Integer
can be used in contexts where objects are required, making it versatile for data manipulation. However, because Integer
is an object, it comes with some overhead in terms of memory usage compared to the primitive int
.
Key Differences Between Int and Integer
Understanding the differences between int
and Integer
is essential for effective programming in Java. Here are the primary distinctions:
- Type:
int
is a primitive data type, whileInteger
is a wrapper class. - Memory Usage:
int
uses less memory thanInteger
. Anint
takes up 4 bytes, whereas anInteger
object requires more memory due to the overhead associated with objects. - Nullability:
int
cannot be null, as it is a primitive type. In contrast,Integer
can be null, which can be useful in certain programming scenarios. - Performance: Operations with
int
are generally faster than those withInteger
because of the overhead associated with object manipulation. - Use Cases: Use
int
for simple calculations and performance-sensitive applications. UseInteger
when you need to work with collections or require the ability to represent a null value.
When to Use Int and When to Use Integer
Choosing between int
and Integer
depends on the specific needs of your application. If you are performing arithmetic operations or require high performance, int
is the better choice. It is efficient and straightforward, making it ideal for loops and mathematical computations.
On the other hand, if you are working with data structures that require objects, such as lists or maps, Integer
becomes necessary. It allows you to take advantage of Java’s rich API for collections, enabling you to store, sort, and manipulate integer values easily.
Here’s a practical example of using both int
and Integer
in a Java application:
import java.util.HashMap;
public class MixedExample {
public static void main(String[] args) {
int primitiveInt = 5;
Integer wrapperInt = Integer.valueOf(primitiveInt);
HashMap<Integer, String> map = new HashMap<>();
map.put(wrapperInt, "Five");
System.out.println("The value for key 5 is: " + map.get(wrapperInt));
}
}
Output:
The value for key 5 is: Five
In this example, we declare a primitive int
and then convert it into an Integer
using Integer.valueOf()
. This allows us to store the integer in a HashMap
, demonstrating how both types can coexist in a program.
Conclusion
In summary, the difference between int
and Integer
in Java is significant. While int
is a primitive data type that offers efficiency and speed, Integer
is a wrapper class that provides additional functionality, such as nullability and compatibility with collections. Understanding when to use each type can greatly enhance your programming skills and improve the performance of your applications. By choosing the right type for your needs, you can write cleaner, more efficient Java code.
FAQ
-
What is the main difference between int and Integer in Java?
The main difference is thatint
is a primitive data type, whileInteger
is a wrapper class that encapsulates anint
value as an object. -
Can an int be null in Java?
No, anint
cannot be null since it is a primitive type. However, anInteger
can be null. -
Which is more memory efficient, int or Integer?
int
is more memory efficient thanInteger
because it uses less memory due to being a primitive type. -
When should I use Integer instead of int?
UseInteger
when you need to work with collections or require the ability to represent a null value. Useint
for performance-sensitive applications. -
How can I convert an int to an Integer in Java?
You can convert anint
to anInteger
using theInteger.valueOf(int)
method or by simply assigning anint
value to anInteger
variable, which will automatically box the primitive.
Haider specializes in technical writing. He has a solid background in computer science that allows him to create engaging, original, and compelling technical tutorials. In his free time, he enjoys adding new skills to his repertoire and watching Netflix.
LinkedInRelated Article - Java Int
- How to Convert Int to Char in Java
- How to Convert Int to Double in Java
- List of Ints in Java
- How to Convert Integer to Int in Java
- How to Check if Int Is Null in Java
- How to Convert Int to Byte in Java