How to Get Thread Id in Java
-
Get Thread Id Using
Thread.getId()
in Java -
Get Current Thread Pool Id Using
Thread.currentThread().getId()
in Java
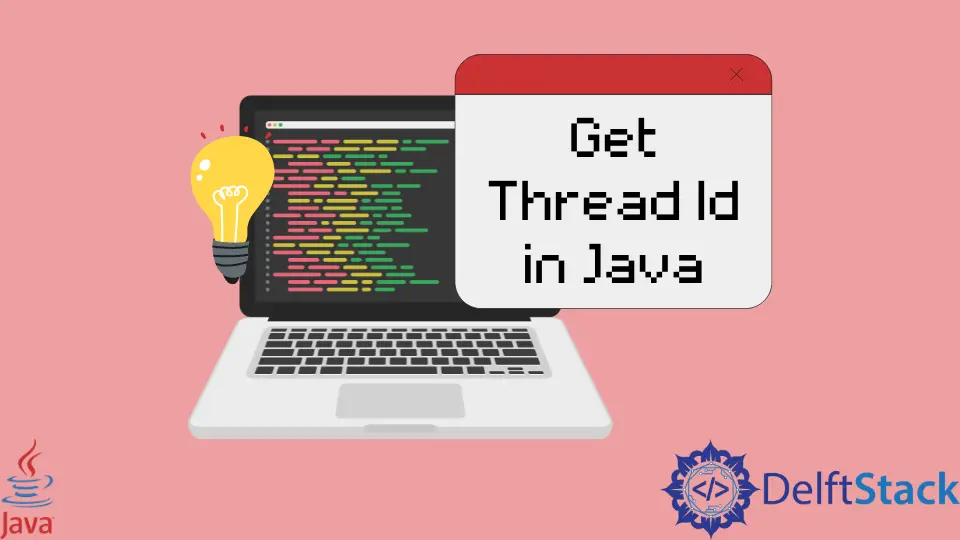
In this tutorial, we will introduce methods to get thread id in Java. We will also see how we can get the current thread’s id from a thread pool.
Get Thread Id Using Thread.getId()
in Java
In this example, we have created a class Task
that implements the Runnable
class because we need its run()
method to execute the thread. The Task
class takes a thread name from its constructor, and the run()
method prints it on the console when it is executed.
In the main()
method, we create two Task
objects in the constructor and then two threads objects in which we pass task1
and task2
to assign the tasks.
We will call the start()
method using thread1
and thread2
to execute the threads. At last, once the threads have been executed, we can get each thread’s id using thread.getId()
, which returns the id as a long
.
public class GetThreadID {
public static void main(String[] args) {
Task task1 = new Task("Task 1");
Task task2 = new Task("Task 2");
Thread thread1 = new Thread(task1);
Thread thread2 = new Thread(task2);
thread1.start();
thread2.start();
System.out.println("Thread1's ID is: " + thread1.getId());
System.out.println("Thread2's ID is: " + thread2.getId());
}
}
class Task implements Runnable {
private String name;
Task(String name) {
this.name = name;
}
@Override
public void run() {
System.out.println("Executing " + name);
}
}
Output:
Thread1's ID is: 13
Thread2's ID is: 14
Executing Task 2
Executing Task 1
Get Current Thread Pool Id Using Thread.currentThread().getId()
in Java
Thread pools are beneficial when it comes to the heavy execution of tasks. In the example below, we create a thread pool using Executors.newFixedThreadPool(numberOfThreads)
. We can specify the number of threads we want in the pool.
The Task
class is responsible for executing the thread in the run()
method. It is a simple class that sets and gets the thread’s name passed in the constructor. To create multiple tasks, we use a for
loop in which five task
objects are created, and five threads are executed in the pool.
Our goal is to get the id of every thread that is being executed currently. To do that, we will use Thread.currentThread().getId()
that returns the current thread’s id. In the output, we can see the ids of all the threads that execute the individual tasks.
Once the tasks are completed, we should stop executing the thread pool using threadExecutor.shutdown()
. !threadExecutor.isTerminated()
is used to wait until the threadExecutor
has been terminated.
package com.company;
import java.util.concurrent.ExecutorService;
import java.util.concurrent.Executors;
public class GetThreadID {
public static void main(String[] args) {
int numberOfThreads = 5;
ExecutorService threadExecutor = Executors.newFixedThreadPool(numberOfThreads);
for (int i = 0; i < 5; i++) {
Task task = new Task("Task " + i);
System.out.println("Created Task: " + task.getName());
threadExecutor.execute(task);
}
threadExecutor.shutdown();
while (!threadExecutor.isTerminated()) {
}
System.out.println("All threads have completed their tasks");
}
}
class Task implements Runnable {
private String name;
Task(String name) {
this.name = name;
}
public String getName() {
return name;
}
@Override
public void run() {
System.out.println("Executing: " + name);
System.out.println(name + " is on thread id #" + Thread.currentThread().getId());
}
}
Output:
Created Task: Task 0
Created Task: Task 1
Created Task: Task 2
Created Task: Task 3
Created Task: Task 4
Executing: Task 0
Executing: Task 2
Executing: Task 1
Executing: Task 4
Executing: Task 3
Task 0 is on thread id #13
Task 1 is on thread id #14
Task 4 is on thread id #17
Task 2 is on thread id #15
Task 3 is on thread id #16
All threads have completed their tasks
Rupam Saini is an android developer, who also works sometimes as a web developer., He likes to read books and write about various things.
LinkedIn