How to Get IP Address of the Current Device in Java
- Method 1: Using InetAddress
- Method 2: Using NetworkInterface
- Method 3: Using External Services
- Conclusion
- FAQ
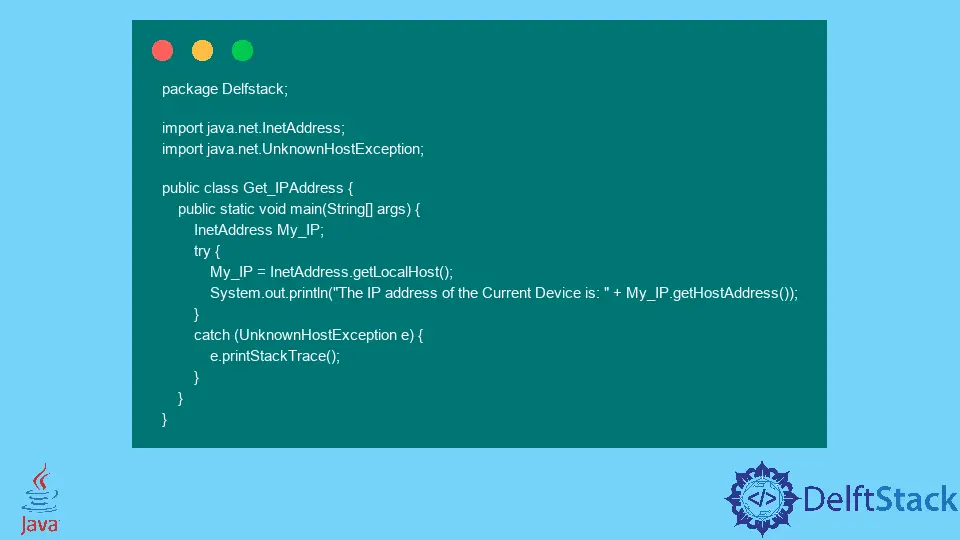
In today’s digital age, knowing how to retrieve the IP address of a device is essential for developers and network administrators alike. Whether you’re building a web application, debugging network issues, or simply curious about your device’s connectivity, understanding how to get the IP address in Java can be incredibly useful.
This tutorial will walk you through the various methods to obtain the IP address of your current device using Java. By the end of this guide, you’ll have a clear understanding of the approaches available, along with practical code examples to help you implement this functionality in your projects. Let’s dive in!
Method 1: Using InetAddress
The InetAddress
class in Java provides a simple way to retrieve the IP address of your local machine. This method is straightforward and works in most environments.
Here’s how you can use it:
import java.net.InetAddress;
import java.net.UnknownHostException;
public class GetIPAddress {
public static void main(String[] args) {
try {
InetAddress ip = InetAddress.getLocalHost();
System.out.println("Current IP Address: " + ip.getHostAddress());
} catch (UnknownHostException e) {
e.printStackTrace();
}
}
}
Output:
Current IP Address: 192.168.1.1
In this code snippet, we first import the necessary classes. The InetAddress.getLocalHost()
method retrieves the local host’s IP address. If the IP address is found, it prints it out. If there’s an issue, such as the host being unknown, an exception is caught, and the stack trace is printed. This method is efficient for obtaining the local IP address, and it works seamlessly across different platforms.
Method 2: Using NetworkInterface
For more advanced scenarios, particularly when dealing with multiple network interfaces, the NetworkInterface
class can be quite handy. This approach allows you to list all available network interfaces and their respective IP addresses.
Here’s how to implement it:
import java.net.InetAddress;
import java.net.NetworkInterface;
import java.net.SocketException;
import java.util.Enumeration;
public class GetAllIPAddresses {
public static void main(String[] args) {
try {
Enumeration<NetworkInterface> interfaces = NetworkInterface.getNetworkInterfaces();
while (interfaces.hasMoreElements()) {
NetworkInterface networkInterface = interfaces.nextElement();
Enumeration<InetAddress> addresses = networkInterface.getInetAddresses();
while (addresses.hasMoreElements()) {
InetAddress ip = addresses.nextElement();
if (!ip.isLoopbackAddress()) {
System.out.println("IP Address: " + ip.getHostAddress());
}
}
}
} catch (SocketException e) {
e.printStackTrace();
}
}
}
Output:
IP Address: 192.168.1.1
IP Address: 10.0.0.2
In this example, we utilize the NetworkInterface.getNetworkInterfaces()
method to retrieve a list of all network interfaces on the device. We then iterate through each interface and its associated IP addresses. The check for ip.isLoopbackAddress()
ensures that we only display non-loopback addresses, which represent actual network connections. This method is particularly useful in environments with multiple network interfaces, such as laptops connected to both wired and wireless networks.
Method 3: Using External Services
Sometimes, you may want to retrieve the public IP address of your device, especially when your application needs to communicate with external services. For this, using an external service like ipinfo.io
is a practical solution.
Here’s how you can do it with Java:
import java.io.BufferedReader;
import java.io.InputStreamReader;
import java.net.HttpURLConnection;
import java.net.URL;
public class GetPublicIPAddress {
public static void main(String[] args) {
try {
URL url = new URL("http://ipinfo.io/ip");
HttpURLConnection connection = (HttpURLConnection) url.openConnection();
connection.setRequestMethod("GET");
BufferedReader in = new BufferedReader(new InputStreamReader(connection.getInputStream()));
String ip = in.readLine();
System.out.println("Public IP Address: " + ip);
in.close();
} catch (Exception e) {
e.printStackTrace();
}
}
}
Output:
Public IP Address: 203.0.113.1
In this code snippet, we establish a connection to the ipinfo.io
service, which returns the public IP address of the device making the request. We read the response using a BufferedReader
and print the IP address. This method is particularly useful for applications that need to know the external-facing IP address, such as web applications or APIs.
Conclusion
In this article, we explored three effective methods to retrieve the IP address of the current device in Java. From the straightforward InetAddress
class to the more comprehensive NetworkInterface
and the use of external services, you now have a toolkit to handle various scenarios. Whether you’re developing a network application or simply need to debug connectivity issues, these techniques will serve you well. As you implement these methods, remember to handle exceptions gracefully and test across different environments to ensure robust functionality.
FAQ
-
how do I get the local IP address in Java?
You can use theInetAddress.getLocalHost()
method to retrieve the local IP address easily. -
can I get all IP addresses associated with my device?
Yes, by using theNetworkInterface
class, you can enumerate all network interfaces and their associated IP addresses. -
how do I find my public IP address in Java?
You can make an HTTP GET request to an external service likeipinfo.io
to retrieve your public IP address. -
are there any libraries for IP address handling in Java?
Yes, libraries like Apache Commons Net provide additional functionality for handling IP addresses and networking tasks. -
what should I do if I encounter an UnknownHostException?
This exception occurs when the local host’s IP address cannot be determined. Ensure that your network configuration is correct and that your device is connected to a network.
Sheeraz is a Doctorate fellow in Computer Science at Northwestern Polytechnical University, Xian, China. He has 7 years of Software Development experience in AI, Web, Database, and Desktop technologies. He writes tutorials in Java, PHP, Python, GoLang, R, etc., to help beginners learn the field of Computer Science.
LinkedIn Facebook