How to Get First Element From List in Java
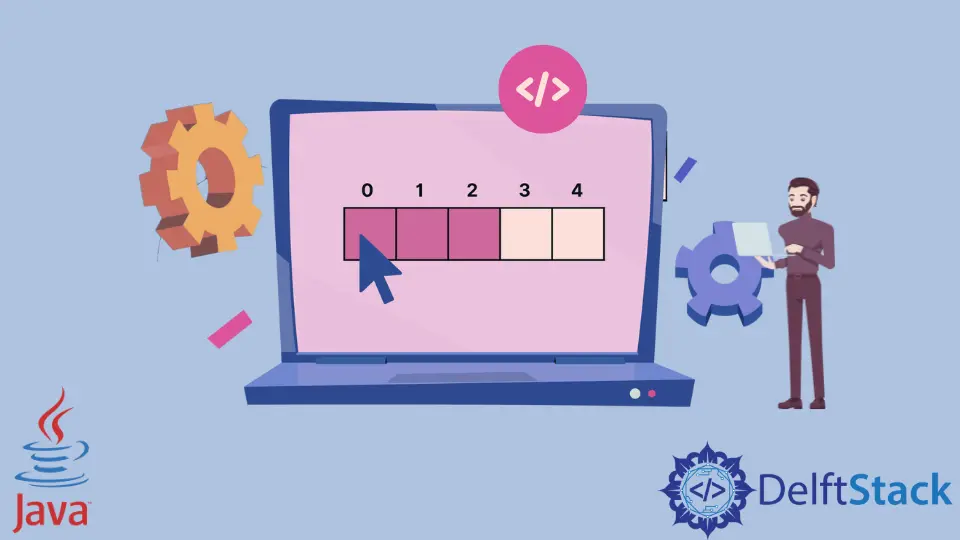
In this article, we’ll see a simple implementation of the list and get the first element from it.
Create List Element in Java
In our example below, we will see how we can create a list element in Java. The code for our example will be something like the below,
// Importing necessary packages
import java.util.ArrayList;
import java.util.Arrays;
import java.util.List;
public class CollectionsDemo {
public static void main(String[] args) {
List<Integer> MyList =
new ArrayList<>(Arrays.asList(8, 9, 10)); // Declaring a List with some element
System.out.println("List elements are: " + MyList); // Printing the list
}
}
In our above example, we first created a List
name, MyList
. After running the above example, we will get the following.
Output:
List elements are: [8, 9, 10]
Get First Element From the List in Java
We can use the method get()
to get a specific element from a list. In this method, we need to provide the index of the specific element.
Let’s have an example. We will extract the first element from the list, and to get it, we need to follow the syntax below.
Syntax:
MyList.get(IndexOfElement)
Remember, the counting starts from 0
. So the index of our first element will be 0
.
Code Example:
// Importing necessary packages
import java.util.ArrayList;
import java.util.Arrays;
import java.util.List;
public class CollectionsDemo {
public static void main(String[] args) {
List<Integer> MyList = new ArrayList<>(Arrays.asList(8, 9, 10));
System.out.println("List elements are: " + MyList);
System.out.println("\nThe first element of the list is: " + MyList.get(0));
}
}
In our above example, we first created a list name MyList
. After that, we extracted the first element by MyList.get(0)
.
Output:
List elements are: [8, 9, 10]
The first element of the list is: 8
Please note that the code examples shared here are written in Java, and you must install Java on your environment if your system doesn’t contain Java.
Aminul Is an Expert Technical Writer and Full-Stack Developer. He has hands-on working experience on numerous Developer Platforms and SAAS startups. He is highly skilled in numerous Programming languages and Frameworks. He can write professional technical articles like Reviews, Programming, Documentation, SOP, User manual, Whitepaper, etc.
LinkedIn