How to Get Day of Week in Java
- Java Get Day of Week
-
Get the Day of the Week Using Java 7
Calendar
Class - Get the Day of the Week Using Java 8 Date API
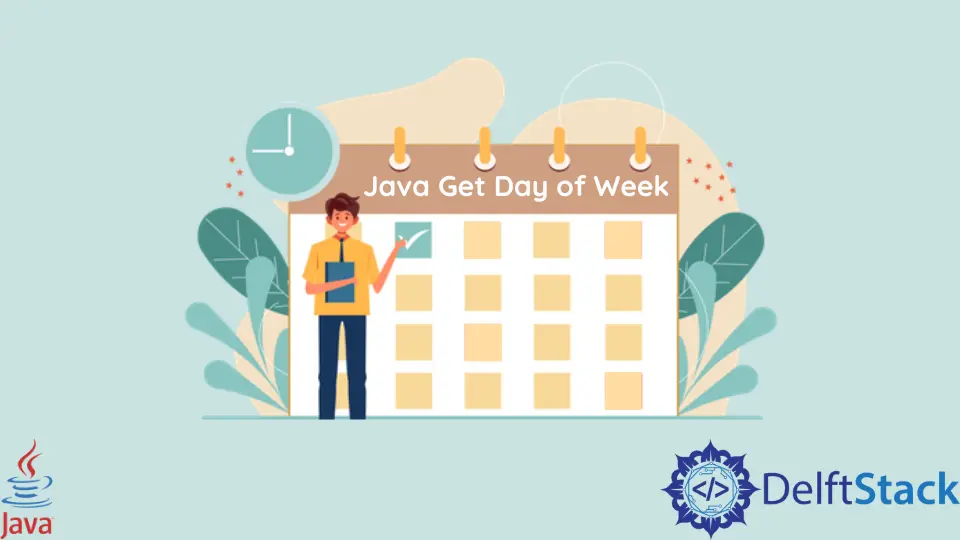
This tutorial demonstrates how to get the day of the Week in Java.
Java Get Day of Week
Sometimes there is a requirement to get the day of a week while working with UI. This can be achieved in Java with the following two methods:
- From Java 7, the legacy
Calendar
class can be used, which defines the constants from SUNDAY (1) to SATURDAY (7). We can get the day using the methodcalendar.get(Calendar.DAY_OF_WEEK)
on the instance of the calendar. - From Java 8, the legacy API Date can be used to get the day of a week from Monday (1) to SUNDAY (7). The method
LocalDate.getDayOfWeek()
can be used to get the day of the week.
Get the Day of the Week Using Java 7 Calendar
Class
The Java 7 Calendar
class is used to get the day of the week. For that, we need to use java.util.Date
and java.util.Calendar
.
Follow the steps below:
-
Create an instance of the
Calendar
class. -
Set the date in calendar using the
setTime(new Date())
method, which will set the current date. -
Now use
get(Calendar.DAY_OF_WEEK)
on the calendar instance to get the day’s number of the week. -
We need to use the
SimpleDateFormat()
Class to get the name of the day.
Let’s implement the above example in Java:
package delftstack;
import java.text.DateFormat;
import java.text.SimpleDateFormat;
import java.util.Calendar;
import java.util.Date;
public class Example {
public static void main(String[] args) {
Calendar Demo_Calendar = Calendar.getInstance();
Demo_Calendar.setTime(new Date());
int Day_Number = Demo_Calendar.get(Calendar.DAY_OF_WEEK);
DateFormat Date_Formatter = new SimpleDateFormat("EEEE");
String Day_Name = Date_Formatter.format(Demo_Calendar.getTime());
System.out.println("The current day of the Week in number is :: " + Day_Number);
System.out.println("The current day of the Week in Text is :: " + Day_Name);
}
}
The code above will show the day of the week for the current date in number and text. See output:
The current day of the Week in number is :: 5
The current day of the Week in Text is :: Thursday
Get the Day of the Week Using Java 8 Date API
To get the day of the week using Java 8 API, we need to use the LocalDate
class and DayofWeek
enum from the Time
package of Java. Follow the steps to get the day of the week using Java 8:
-
Create an instance of
LocalDate
with the methodnow()
, which will be the current date. -
Use the
DayofWeek
enum and thegetDayOfWeek()
method on the instance ofLocalDate
to get the current day of the week. -
Use the
getValue()
method onDayofWeek
to get the day in number. -
Use the
getDisplayName()
method onDayofWeek
to show the name of the day in full and short formats.
Let’s try to implement the above example in Java:
package delftstack;
import java.text.DateFormat;
import java.text.SimpleDateFormat;
import java.time.DayOfWeek;
import java.time.LocalDate;
import java.time.format.TextStyle;
import java.util.Locale;
public class Example {
public static void main(String[] args) {
LocalDate Current_Date = LocalDate.now();
DayOfWeek Week_Day = Current_Date.getDayOfWeek();
System.out.println("The current day of the Week is :: " + Week_Day);
System.out.println("The current day of the Week in Number is :: " + Week_Day.getValue());
System.out.println("The current day of the Week in full format is :: "
+ Week_Day.getDisplayName(TextStyle.FULL, Locale.getDefault()));
System.out.println("The current day of the Week in short format is :: "
+ Week_Day.getDisplayName(TextStyle.SHORT, Locale.getDefault()));
}
}
The code above will get the day of the current date and convert it to number, full, and short formats. See output:
The current day of the Week is :: THURSDAY
The current day of the Week in Number is :: 4
The current day of the Week in full format is :: Thursday
The current day of the Week in short format is :: Thu
Sheeraz is a Doctorate fellow in Computer Science at Northwestern Polytechnical University, Xian, China. He has 7 years of Software Development experience in AI, Web, Database, and Desktop technologies. He writes tutorials in Java, PHP, Python, GoLang, R, etc., to help beginners learn the field of Computer Science.
LinkedIn Facebook