How to Get Length of Array in Java
-
Use the Array
length
Attribute to Get the Length of Array in Java -
Use the
for-each
Loop to Get the Length of Array in Java
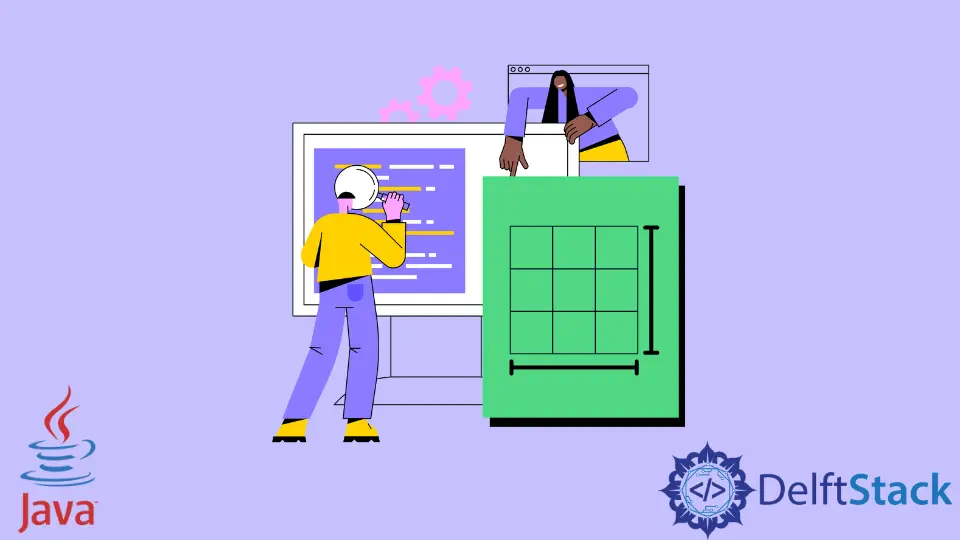
This tutorial article elaborates on how to get the array length in Java.
There are two ways to get the length of the array in Java as follows.
- Array
length
attribute - The
for-each
loop
Use the Array length
Attribute to Get the Length of Array in Java
In Java, the length of an array is the number of array elements. There is no predetermined method to get the length of an array in Java. We can get the array length in Java using the array’s length
attribute.
Java offers the length
attribute in the array to get the length of the array. The length
attribute can be accessed using the dot operators (.
) along with the array name.
The example code of getting the length using the length
attribute is as follows.
public class ArrayLength {
public static void main(String args[]) {
int Array[] = {1, 2, 3, 4, 5};
int ArrLength = 0;
ArrLength = Array.length;
System.out.println("The Length of Array is : " + ArrLength);
}
}
Output:
The Length of Array is : 5
Use the for-each
Loop to Get the Length of Array in Java
We can also get the length of an array using the for-each
loop in Java by iterating the array and counting the array elements. We get the length of an array after the last iteration.
The example code of getting the length using the for-each
loop is as follows.
public class ArrayLength {
public static void main(String args[]) {
int Array[] = {1, 2, 3, 4, 5};
int ArrLength = 0;
for (int Elements : Array) ArrLength++;
System.out.println("The Length of Array is : " + ArrLength);
}
}
Output:
The Length of Array is : 5
We increment the variable ArrLength
upon every iteration in the for-each
loop. After completing all the iterations, we get the length of an array stored in the variable ArrLength
.