How to Generate Random Doubles in an Array in Java
-
Generate Random
double
in a Java Array Using thejava.util.Random
Class -
Generate Random
double
in a Java Array UsingThreadLocalRandom
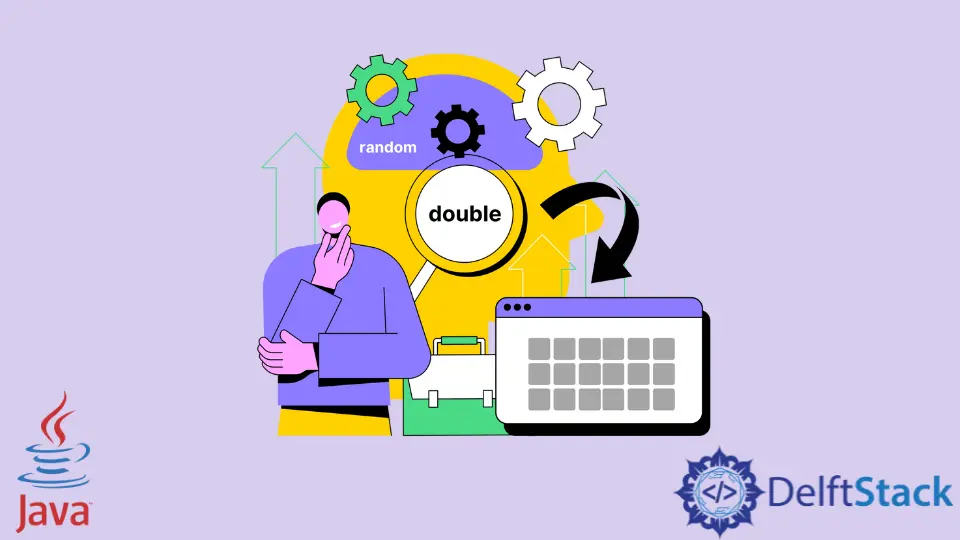
This article will introduce how to generate random doubles in a Java array.
Generate Random double
in a Java Array Using the java.util.Random
Class
In Java, we utilize the nextDouble()
method of the java.util.Random
class to generate a random array of doubles. This function returns the next random double value generated by this random number generator.
Example:
import java.util.Random;
public class RandomDoubles {
public static void main(String[] args) {
Random rd = new Random();
double[] array = new double[3];
for (int i = 0; i < array.length; i++) {
array[i] = rd.nextDouble();
System.out.println(array[i]);
}
}
}
Here, rd.nextDouble()
returns a number between 0 and 1.0.
Output:
0.6281807590035252
0.34456583017282394
0.23684352236085848
Shorter Version
There is a shorter version of doing the same thing as the following.
Example:
import java.util.Random;
public class RandomDoubles {
public static void main(String[] args) {
Random rd = new Random();
double[] array = rd.doubles(5, 10, 100).toArray();
}
}
Th first argument of rd.doubles
method takes the number of random doubles you want in the array. And, the second and third argument takes a minimum and maximum value of range for generating random numbers.
Output:
78.21950288465801 80.12875947698258 94.95442635507693 88.63415507060164 31.283712117527568
Generate Random double
in a Java Array Using ThreadLocalRandom
We can also generate random doubles by using ThreadLocalRandom
.
Example:
import java.util.concurrent.ThreadLocalRandom;
public class RandomDoubles {
public static void main(String[] args) {
double[] randoms = ThreadLocalRandom.current().doubles(5).toArray();
for (double num : randoms) {
System.out.println(num);
}
}
}
Output:
0.5417255613845797
0.2522145088132569
0.42238415650855565
0.8432309480218088
0.13192651019478996
Shiv is a self-driven and passionate Machine learning Learner who is innovative in application design, development, testing, and deployment and provides program requirements into sustainable advanced technical solutions through JavaScript, Python, and other programs for continuous improvement of AI technologies.
LinkedInRelated Article - Java Array
- How to Concatenate Two Arrays in Java
- How to Convert Byte Array in Hex String in Java
- How to Remove Duplicates From Array in Java
- How to Count Repeated Elements in an Array in Java
- Natural Ordering in Java
- How to Slice an Array in Java