How to Escape Characters in Java
- Escape Sequences and Their Descriptions in Tabular Format
- Escape Sequences in Java and How to Use Them
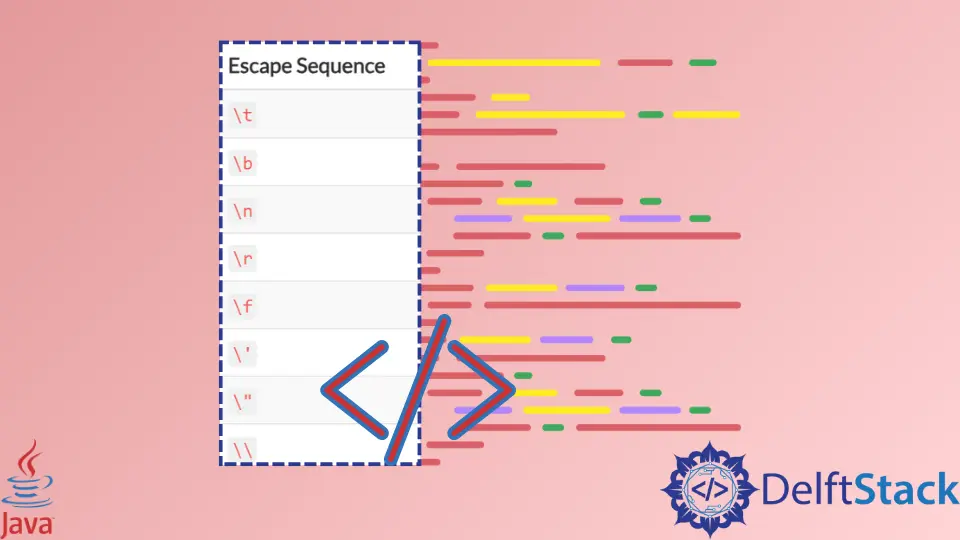
This article introduces all the commonly used escape characters or sequence in Java and their usage with an example.
Escape Sequences and Their Descriptions in Tabular Format
Escape Sequence | Description |
---|---|
\t |
A tab |
\b |
A backspace |
\n |
A new line character |
\r |
A carriage return |
\f |
A form feed |
\' |
A single quote |
\" |
A double quote |
\\ |
A backslash character |
Escape Sequences in Java and How to Use Them
In the above section, we saw the short descriptions of the various escape sequences; now we will discuss these escape characters with an example.
Note that some compilers may give different or unusual results.
\t
inserts a tab or a large space in the text at the point where it is used. It can be used when we want to show something in a different section. In the following example, tabExample
has a string with \t
escape sequence between two words. The output shows the result.
\b
inserts a backspace or can say that it removes the character behind it. backspaceExample
uses \b
, and the extra space between the words has been removed as seen in the output.
\n
inserts a new line at the point in the text where it is being used. newLineExample
is a complete string, but when we use \n
, the output shows that a part of the string goes into the new line.
\r
inserts a carriage return at the point where it is being used. It just goes to the beginning of the line and then prints the remaining part of the string. carriageReturnExample
uses \r
and the output shows that the part after the \r
character goes to the new line and starts from the beginning.
\f
inserts a formfeed at the point of usage in the text. It is rarely used nowadays. The new compilers give different outputs that make it difficult to work with.
\'
inserts or escapes a single quote. 'singleQuoteExample'
contains a single quote character, but we cannot use a single quote directly in a char
as it will behave differently. Thus, to escape the single quote, we use \'
.
\"
inserts or escapes a double quote. It works exactly like escaping a single quote.
\\
inserts or escapes a backslash in a text. backslashExample
has a string with \\
that outputs the string with a backslash.
public class EscapeCharacters {
public static void main(String[] args) {
String tabExample = "This is just an \t example";
String backspaceExample = "This is just an \bexample";
String newLineExample = "This is just an \n example";
String carriageReturnExample = "This is just an example \r We got into a new line ";
String formFeedExample = "This is just an example \f We got into a new line ";
char singleQuoteExample = '\'';
String doubleQuotesExample = "\"This string is surrounded with double quotes\"";
String backslashExample = "This string is surrounded with a \\ backslash ";
System.out.println("1.: " + tabExample);
System.out.println("2.: " + backspaceExample);
System.out.println("3.: " + newLineExample);
System.out.println("4.: " + carriageReturnExample);
System.out.println("5.: " + formFeedExample);
System.out.println("6.: " + singleQuoteExample);
System.out.println("7.: " + doubleQuotesExample);
System.out.println("8.: " + backslashExample);
}
}
Output:
1.: This is just an example
2.: This is just anexample
3.: This is just an
example
4.: This is just an example
We got into a new line
5.: This is just an example
We got into a new line
6.: '
7.: "This string is surrounded with double quotes"
8.: This string is surrounded with a \ backslash
Rupam Saini is an android developer, who also works sometimes as a web developer., He likes to read books and write about various things.
LinkedIn