How to Assign Custom Values to Enums in Java
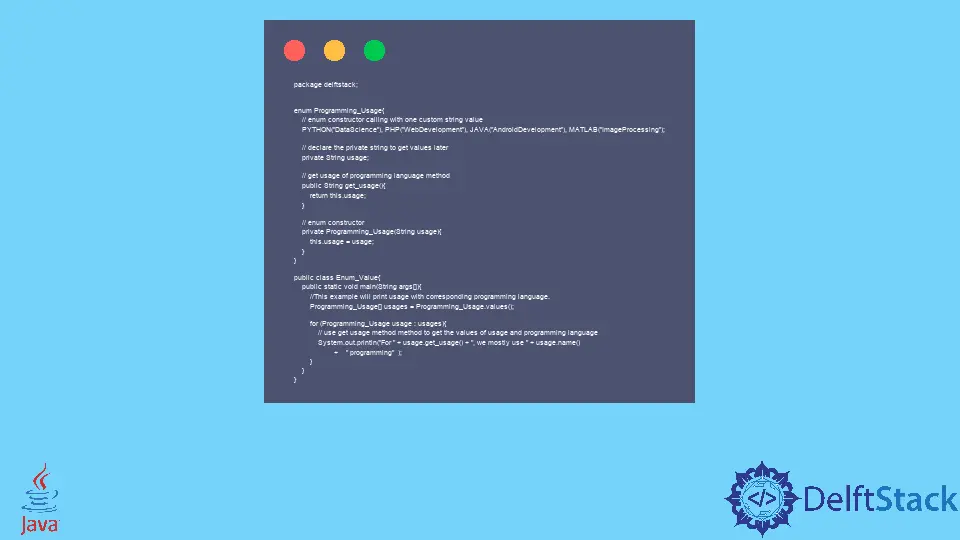
The Java enum
data type creates and uses constant values in a language-supported way. The enum
becomes more type-safe than constant variables like int or string by defining a finite set of values.
This tutorial demonstrates how to create enum
with custom variables in Java.
Assign Custom Values to Enums in Java
Let’s attach the customized values to enums and try to get and print them. The example below creates an enum
of different programming languages with the corresponding usage.
package delftstack;
enum Programming_Usage {
// enum constructor calling with one custom string value
PYTHON("DataScience"),
PHP("WebDevelopment"),
JAVA("AndroidDevelopment"),
MATLAB("ImageProcessing");
// declare the private string to get values later
private String usage;
// get usage of programming language method
public String get_usage() {
return this.usage;
}
// enum constructor
private Programming_Usage(String usage) {
this.usage = usage;
}
}
public class Enum_Value {
public static void main(String args[]) {
// This example will print usage with corresponding programming language.
Programming_Usage[] usages = Programming_Usage.values();
for (Programming_Usage usage : usages) {
// use get usage method method to get the values of usage and programming language
System.out.println(
"For " + usage.get_usage() + ", we mostly use " + usage.name() + " programming");
}
}
}
The code above will get the customized values of the enum and print the programming languages with the corresponding usage where name()
is the built-in method for enum and get_usage()
is the method we defined.
Output:
For DataScience, we mostly use PYTHON programming
For WebDevelopment, we mostly use PHP programming
For AndroidDevelopment, we mostly use JAVA programming
For ImageProcessing, we mostly use MATLAB programming
Sheeraz is a Doctorate fellow in Computer Science at Northwestern Polytechnical University, Xian, China. He has 7 years of Software Development experience in AI, Web, Database, and Desktop technologies. He writes tutorials in Java, PHP, Python, GoLang, R, etc., to help beginners learn the field of Computer Science.
LinkedIn Facebook