Introduction to Interceptors in Java EE
- Introduction to the Interceptors in Java EE
-
the
javax.interceptor
Package in Java - the Interceptor Method in Java
- Use of Interceptor Methods and Interceptor Classes in Java
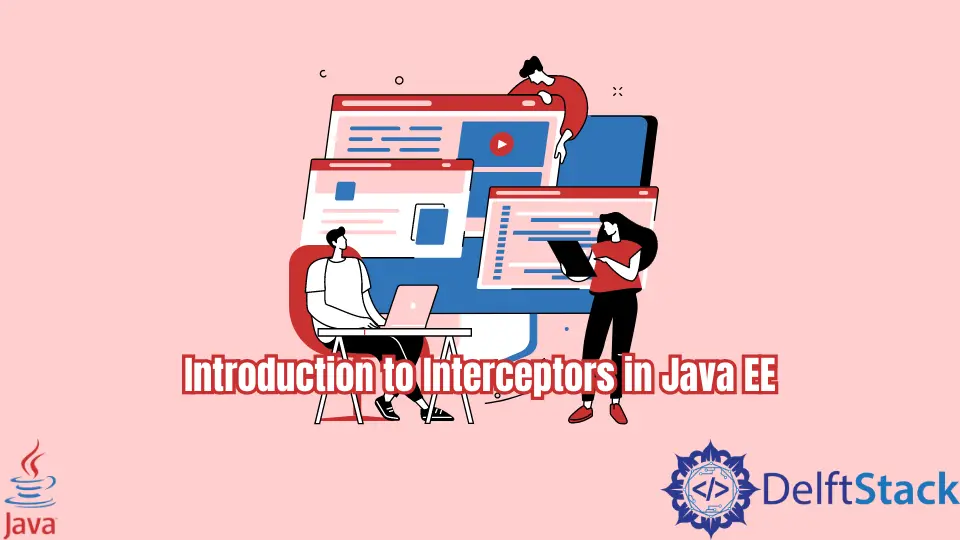
In this article, we’ll go through an introduction to the interceptors in Java EE. It includes interceptor methods, interceptor classes, and lifecycle callback interceptors.
Introduction to the Interceptors in Java EE
In Java EE version 5, we could use interceptors only on Enterprise JavaBeans (EJBs
). While the Java EE version 6 brought new specifications, we could apply that more generically because it was abstracted at a higher level.
The Java EE version 7 introduced the @Transactional
annotation, allowing container-managed transactions out of the Enterprise JavaBeans. It is defined as the interceptor binding & implemented by the Java EE runtime.
The interceptor is the class whose methods are invoked when a business method is invoked on the target. For instance, lifecycle events such as those methods that destroy or create a bean occur.
We use interceptors to implement the cross-cutting concerns. For instance, security, auditing, and logging from business logic.
We can define interceptors as class interceptors or method interceptors, and the preferred approach is to use metadata annotations to define an interceptor. We can also define them in an application descriptor, but they would not be portable across Java EE servers.
the javax.interceptor
Package in Java
The metadata annotations resides in javax.interceptor
package. Some examples of metadata annotations are @AroundTimeout
and @AroundInvoke
.
The javax.interceptor
package has all the interfaces and annotations we use to define the interceptor classes and methods and is also used for binding an interceptor class to the target class. The functionality of an interceptor is defined in Java Interceptor Specifications.
The interceptors’ specifications define two kinds of interception points: lifecycle callback interception and business method interception. The Context and Dependency Injection (CDI
) improves this functionality and improves with a semantic & annotation-based approach for binding the interceptors to beans.
the Interceptor Method in Java
It is a method of the target class or an interceptor class. Invoked for interposing on the invocation of the target class’ method, and also, on the target class’ constructor and lifecycle event etc.
For a target class, we can declare the interceptor method in the target class, interceptor class, or in superclass of the interceptor class or target class. The AroundConstruct
is the only method we can define in the interceptor class or its superclass.
The AroundInvoke
, AroundTimeout
, PostConstruct
, and PreDestroy
are also the interceptor methods. While PostConstruct
, AroundConstruct
, & PreDestroy
are collectively referred to as lifecycle callback interceptor methods.
Interceptor Classes
The interceptor class is different from the target class. The methods of the interceptor class invoke in response to invocations on a target class, and a target class may associate with any number of the interceptor classes.
Remember, the interceptor class must contain a public constructor with no arguments. For a class, we use metadata annotations to define interceptor classes and methods, and we can also use deployment descriptors to define interceptor classes/methods.
The interceptor classes are invoked in the order they have been defined in the javax.interceptor.Interceptors
annotation. Remember, this order is not fixed, and the deployment descriptor can override it.
Interceptor Lifecycle
The lifecycle of interceptor classes is the same as their associated target classes. When we instantiate the target class, the interceptor class is also instantiated, and we do it for every interceptor class that we declare in a target class.
We instantiate all the interceptor and target classes before invoking the @PostConstruct
callbacks. Further, destroy the instances of the interceptor target class before invoking @PreDestroy
callbacks.
Use of Interceptor Methods and Interceptor Classes in Java
We only need to put them around our method as follows to use interceptor methods. We call it the intercept method interceptor.
@AroundTimeout public void timeoutInterceptorMethod(InvocationContext ctx){...}...
For interceptor classes, we add interceptor annotations. Put them in our interceptor class.
See the following example.
Example:
@Stateless
public class OrderBean {
... @Interceptors(OrderInterceptor.class) public void placeOrder(Order order){...}...
}
You may check this to explore interceptors in detail.