How to Delegate in Java
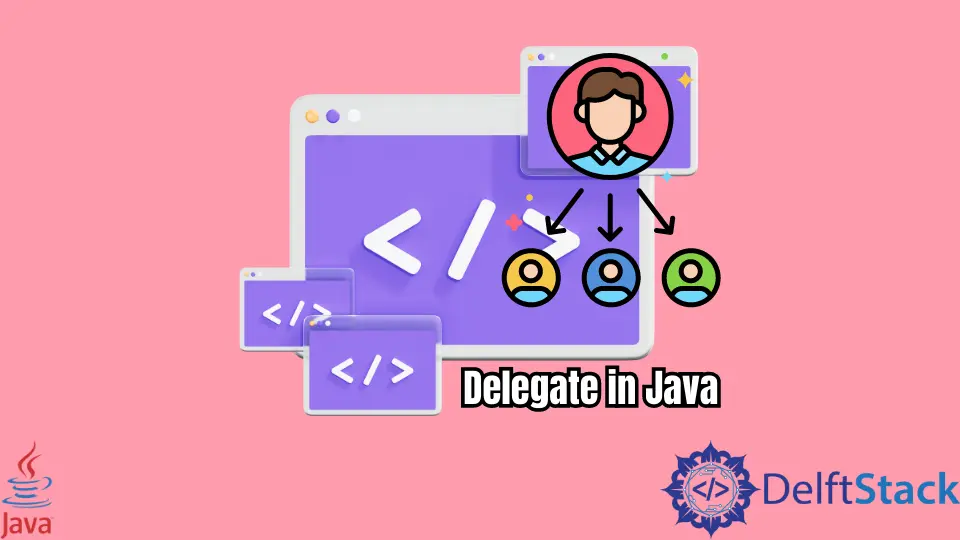
If you are working with programming languages like Java, you may be familiar with inheritance. But there is an alternative to inheritance, the Delegation
.
You can use an object from another class as an instance variable through the Delegation
. In some ways, it is better than inheritance as it does not force you to accept unnecessary methods from the superclass.
Besides, the instance is of a known class. We call the Delegation
a relationship maker between the objects where one object can forward a method call to another object.
The Delegation
can provide you flexibility on run-time. But the problem is that most popular programming languages do not support the Delegation
.
In this article, we will look at the use of Delegation
in Java. Also, we will discuss the topic by using an example with proper explanations to make the topic easier.
Delegation in Java
Below we will look at a simple example of Delegation
and describe it part by part.
class MainPrinter { // The class that hold the actual PrintData() method
void PrintData() {
System.out.println("This is the Delegate.");
}
}
class MyPrinter { // The class that calls PrintData() method from the object of class MainPrinter
MainPrinter p = new MainPrinter(); // Creating an object for MainPrinter class.
void PrintData() {
p.PrintData(); // Calling the method from MainPrinter class object.
}
}
class TestDelegate {
public static void main(String[] args) {
MyPrinter printer = new MyPrinter(); // Creating an object for MyPrinter class.
printer.PrintData(); // Calling the method from MyPrinter class object.
}
}
Above, we shared an example that illustrates Delegation
. We already commanded the purpose of each line on the code.
In the example above, we created three different classes named MainPrinter
, MyPrinter
, and the TestDelegate
, where the class MainPrinter
contains the actual method that will print the data.
And the class MyPrinter
also contains the printData()
method that mainly calls another method from another class named MainPrinter
.
But if you look at the handler class named TestDelegate
, you can see that we created an object from the MyPrinter
class where the MyPrinter
class does not have the actual printData()
method.
After you run the above example code, you will get an output like the one below.
This is the Delegate.
Please note that the code examples shared here are in Java, and you must install Java on your environment if your system doesn’t contain Java.
Aminul Is an Expert Technical Writer and Full-Stack Developer. He has hands-on working experience on numerous Developer Platforms and SAAS startups. He is highly skilled in numerous Programming languages and Frameworks. He can write professional technical articles like Reviews, Programming, Documentation, SOP, User manual, Whitepaper, etc.
LinkedIn