How to Set Time Zone of a java.util.Date
-
Use
ZonedDateTime
to Set Time Zone of ajava.util.Date
-
Use
UTC Instant
to Set Time Zone of ajava.util.Date
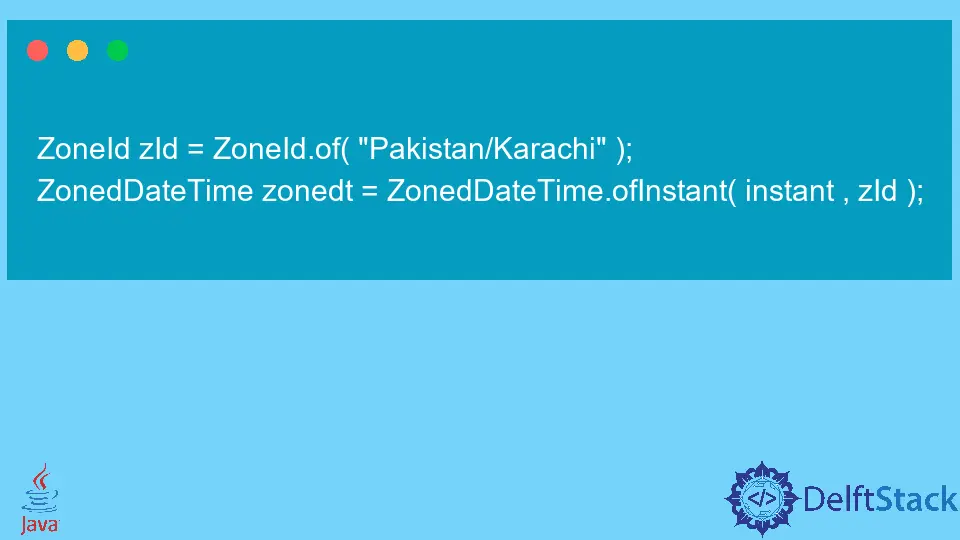
This quick tutorial will look at how to use Java to set the time zone associated with a date. The java.util.Date
has no time zone.
It denotes the UTC/GMT standard with no time zone offset. Because when creating a string representation, its toString
function uses the JVM’s default time zone as the basis for its application.
The built-in java.util.Date
, .Calendar
, and java.text.SimpleDateFormat
classes are generally difficult to work with and should be avoided. We’ll use the java.time
package that comes standard with Java 8 instead.
Below are the ways the java.time
classes can represent a time zone.
Use ZonedDateTime
to Set Time Zone of a java.util.Date
Applying a time zone, an offset, and the guidelines for dealing with irregularities like Daylight Saving Time
or DST
is a better option than the alternative.
When a ZoneId
is used with an Instant
, a ZonedDateTime
is produced. Always be sure to use the correct name for the time zone.
Abbreviations of three to four letters, such as EST
that are neither specific nor standardized should never be used.
ZoneId zId = ZoneId.of("Pakistan/Karachi");
ZonedDateTime zonedt = ZonedDateTime.ofInstant(instant, zId);
Use UTC Instant
to Set Time Zone of a java.util.Date
Instant
is the fundamental building component of java.time
. It represents an instant
on the timeline that is measured in UTC
.
Implement the majority of your application logic using Instant
objects.
Instant instant = Instant.now();
LocalDateTime
When any text being parsed does not include any indicators of offset or zone, then it should be interpreted as a LocalDateTime
.
If you are definite about the time zone that should be used, you may generate a ZonedDateTime
by assigning a ZoneId
. See the following sample of code.
LocalDateTime.parse("2022-09-10T01:13:15.123456789").atZone(ZoneId.of("Asia/Pakistan"))
I have been working as a Flutter app developer for a year now. Firebase and SQLite have been crucial in the development of my android apps. I have experience with C#, Windows Form Based C#, C, Java, PHP on WampServer, and HTML/CSS on MYSQL, and I have authored articles on their theory and issue solving. I'm a senior in an undergraduate program for a bachelor's degree in Information Technology.
LinkedIn