How to Use Custom Serializer With Jackson in Java
- Serialization in Java
- Custom Serializer With Jackson on the Class
-
Use the
serialize()
Method in Java
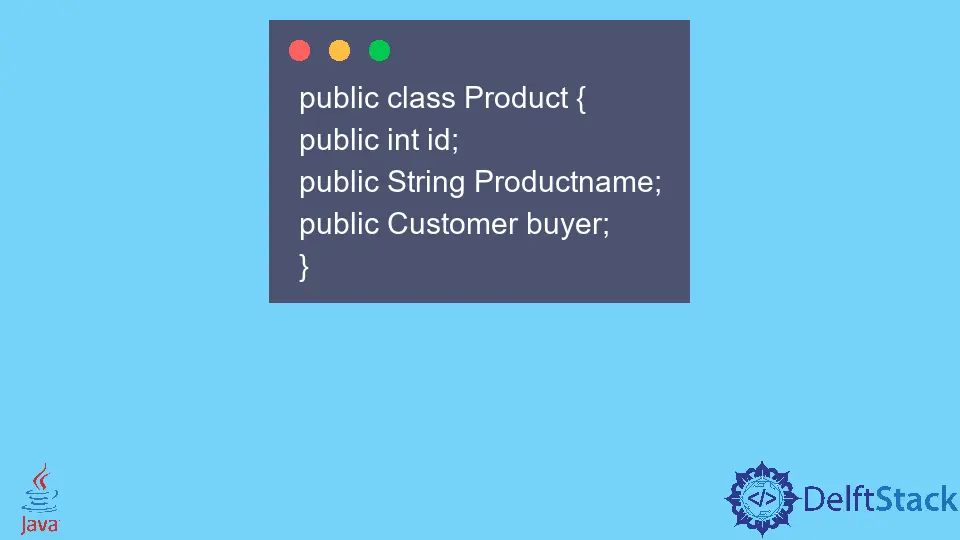
This tutorial will look at how to utilize a custom Serializer with Jackson in Java. First, let’s have a look at the serialization concept.
Serialization in Java
The serialization process in Java is a technique to convert an object to and from a stream of bytes. This not only makes it possible to store an object in a file, but it also makes it possible to send an object over a network.
The serialization process begins with creating an implementation of the Serializable interface, followed by the use of Java.io
.
Custom Serializer With Jackson on the Class
Let’s create two simple classes, ’ Customerand
Product`.
Code - Customer
class:
public class Customer {
public int id;
public String Cname;
}
Code - Produtc
class:
public class Product {
public int id;
public String Productname;
public Customer buyer;
}
Apply JsonSerialize
in the Class
Now, We’ll register the serializer in the class like in the following.
@JsonSerialize(using = ProductSerializer.class)
public class Product {
public int id;
public String Productname;
public Customer buyer;
}
Then apply standard serialization.
Product p = new Product(10, "drinks", new Customer(12, "Peter"));
String serialized = new ObjectMapper().writeValueAsString(p);
Lastly, We will obtain the individualized JSON
output generated by the serializer and defined using the @JsonSerialize
.
Use the serialize()
Method in Java
We can use the StdSerializer
class to implement a custom serializer. In this case, we will need to override the serialize()
method.
Syntax:
public abstract void serialize(Product val, JsonGenerator jg, SerializerProvider p)
throws IOException
If you don’t mind having a custom serializer, you don’t need to develop one for the Product
class. Instead, you should write one for the Customer
class.
public void serialize(Product value, JsonGenerator jg, SerializerProvider p)
throws IOException, JsonProcessingException {
jg.writeNumber(id);
}
I have been working as a Flutter app developer for a year now. Firebase and SQLite have been crucial in the development of my android apps. I have experience with C#, Windows Form Based C#, C, Java, PHP on WampServer, and HTML/CSS on MYSQL, and I have authored articles on their theory and issue solving. I'm a senior in an undergraduate program for a bachelor's degree in Information Technology.
LinkedIn