How to Create File if Not Exists in Java
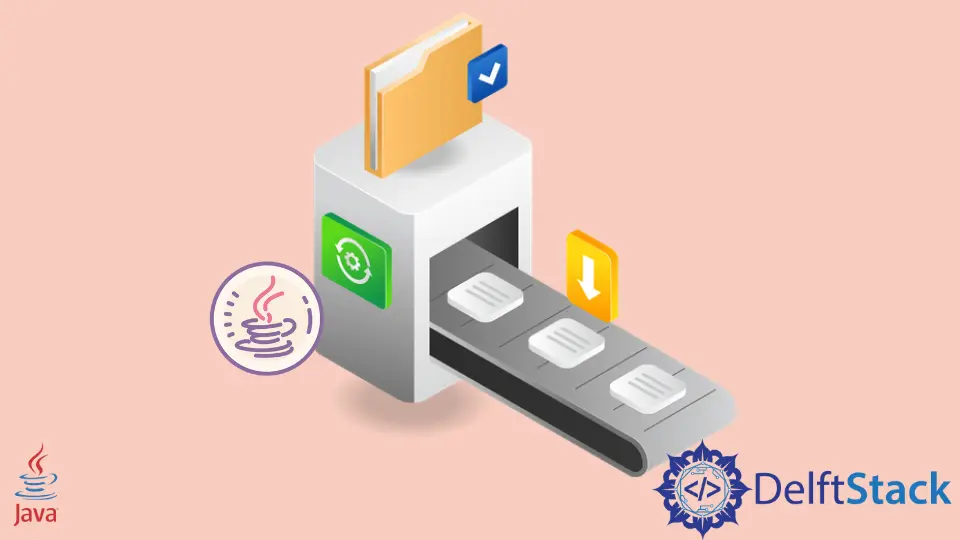
Creating a file in Java is an easy operation. This tutorial demonstrates how to create a file in Java if it does not exists.
Create File if It Does Not Exist in Java
java.io.File
class in Java has a method createNewFile()
that will create a new empty file with the given name only if the file does not exists. It will return true
if the file is created and false
if it already exists.
Let’s try an example.
package delftstack;
import java.io.File;
import java.io.IOException;
public class Create_File {
public static void main(String[] args) {
try {
File New_File = new File("NewDelftstack.txt");
if (New_File.createNewFile()) {
System.out.println("The file is created successfully!");
} else {
System.out.println("The file already exists.");
}
} catch (IOException e) {
e.printStackTrace();
}
}
}
The code above will create a file with the given name if it is not already existing. See output:
The file is created successfully!
Sheeraz is a Doctorate fellow in Computer Science at Northwestern Polytechnical University, Xian, China. He has 7 years of Software Development experience in AI, Web, Database, and Desktop technologies. He writes tutorials in Java, PHP, Python, GoLang, R, etc., to help beginners learn the field of Computer Science.
LinkedIn Facebook