How to Use Regex in the String.contains() Method in Java
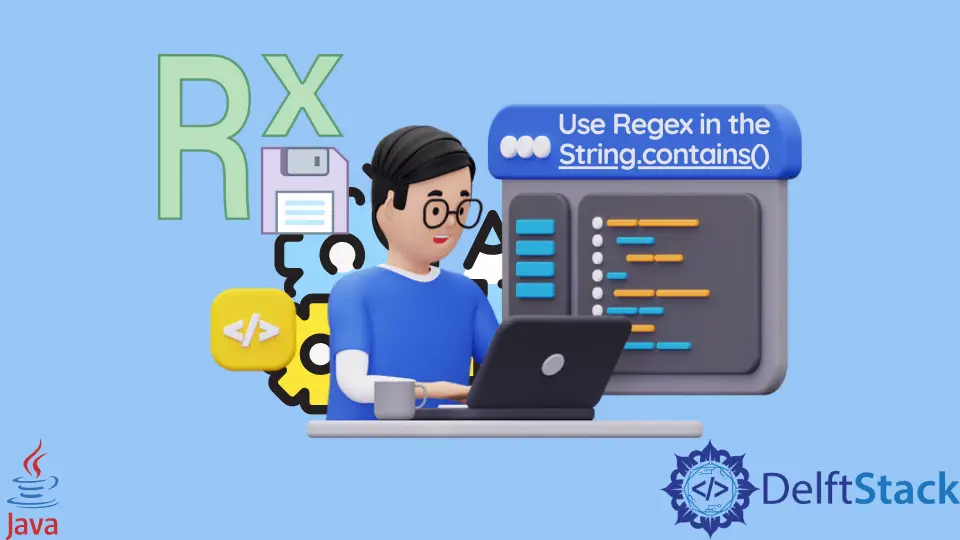
This article will demonstrate how to utilize regex in Java’s String.contains()
function.
The String.contains()
function scans for a certain character sequence in a string. If a series of char values are discovered in this string, it returns true
; otherwise, it returns false
.
Use Regex in the String.contains()
Method in Java
It transforms a CharSequence
to a String before calling the indexOf
function. If indexOf
finds the string, it returns 0
or a greater number; otherwise, it returns -1
.
So, if a series of char values exists, the contains()
function returns true
; otherwise, it returns false
.
Syntax:
public boolean contains(CharSequence seq) {
return indexOf(seq.toString()) > -1;
}
Here’s an example of seeing if the charSequence
is present or not.
To begin with, create a string type variable named st
in the class, and fill it with a string value.
String st = " Hey folks Delft Stack here ";
Now, you can use the .contains()
method here:
System.out.println(st.contains("Test"));
class shanii {
public static void main(String args[]) {
String st = " Hey folks Delft Stack here ";
System.out.println(st.contains("Test"));
System.out.println(st.contains("Delft"));
System.out.println(st.contains("DS"));
System.out.println(st.contains("D"));
}
}
Output:
false
true
false
true
Because it was not contained in the string we named st
, the first parameter supplied, "Test"
, would output false
. And since "Delft"
was found in the string st
, it will return true
and vice versa.
I have been working as a Flutter app developer for a year now. Firebase and SQLite have been crucial in the development of my android apps. I have experience with C#, Windows Form Based C#, C, Java, PHP on WampServer, and HTML/CSS on MYSQL, and I have authored articles on their theory and issue solving. I'm a senior in an undergraduate program for a bachelor's degree in Information Technology.
LinkedIn