Java Conditional Compilation
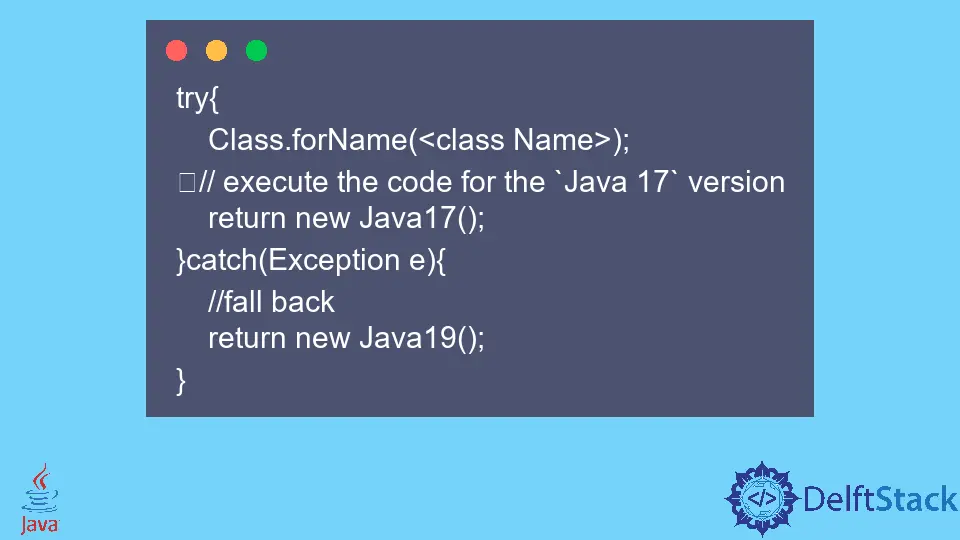
In this article, we will learn to compile the Java code conditionally. The conditional compile removes the chunk of code while compiling the code based on certain conditions, which can be Java version-specific or OS-specific.
Conditional Compile in Java
The simplest way to set the conditional compilation is to create a global Boolean variable and compile the code according to its values. Let’s understand the conditional compile using the below example code.
Use the Boolean Variable for Conditional Compilation in Java
In the example below, we have created the file name called javaversion.java
and created the Javaversion
class inside the file, which contains the public static
variable name Version
.
In the test.java
file, we are accessing the Version
variable and compiling the Java code according to the version.
javaversion.java
:
class Javaversion {
//
public static final String Version = "Java 19";
}
test.java
:
class Test {
public static void main(String[] args) {
System.out.println("Hello, World!");
if (Javaversion.Version == "Java 19") {
System.out.println("Code block executed for java 19");
} else {
System.out.println("Code block executed for < java 19");
}
}
}
Output:
Hello, World!
Code block executed for java 19
Also, users can create different files with different codes of Java and compile the different files according to certain conditions.
try {
Class.forName(<class Name>);
// execute the code for the `Java 17` version
return new Java17();
} catch (Exception e) {
// fall back
return new Java19();
}
In the above pseudo-code, we are detecting the class using the Class.name()
method and returning the object of the Java17
or Java19
class. Here, users must create the Java17
and Java19
classes in different files.