How to Compare Content of Two Files in Java
- Byte by Byte Comparison of Two Files in Java
- Line by Line Comparison of Two Files in Java
-
Compare Two Files With
Files.mismatch()
in Java - Compare Two Files With Apache Commons IO in Java
-
Compare Two Files With
Arrays.equals()
in Java
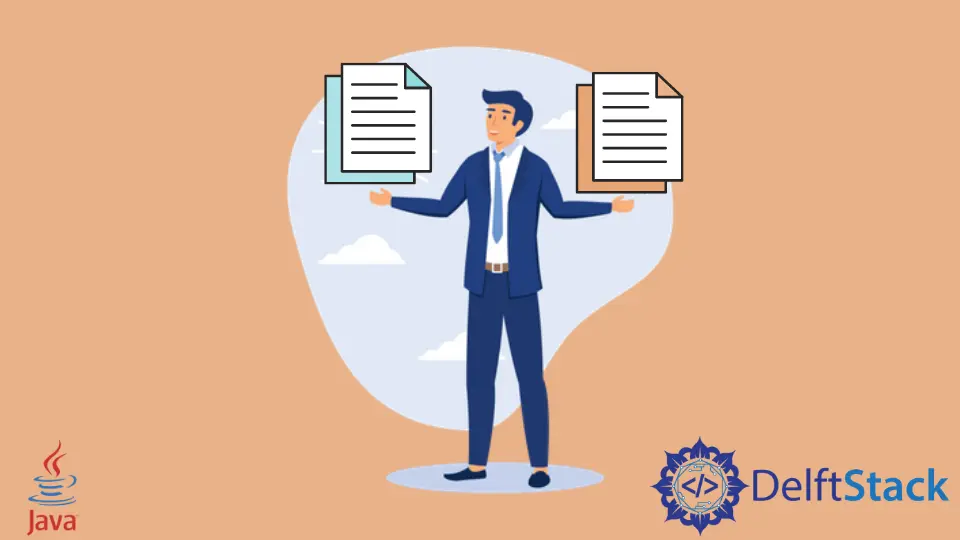
There are quite a few ways to compare the content of two files. This tutorial demonstrates different ways of comparing two files in Java.
Byte by Byte Comparison of Two Files in Java
The BufferedInputStream
is used to read the file and compare each byte of one file to the other file. We use the read()
method from BufferedReader
to read each byte and compare it.
We created two similar text files with different names, delftstack1
and delftstack2
, with the content:
Hello This is delftstack.com
The best online platform for learning different programming languages.
Let’s try an example to compare both files byte by byte.
package delftstack;
import java.io.BufferedReader;
import java.io.File;
import java.io.IOException;
import java.nio.file.Files;
import java.nio.file.Path;
import java.util.Arrays;
public class File_Compare {
private static boolean CompareFilesbyByte(Path File_One, Path File_Two) {
try {
long file_size = Files.size(File_One);
if (file_size != Files.size(File_Two)) {
return false;
}
if (file_size < 2048) {
return Arrays.equals(Files.readAllBytes(File_One), Files.readAllBytes(File_Two));
}
// Compare byte-by-byte
try (BufferedReader Content1 = Files.newBufferedReader(File_One);
BufferedReader Content2 = Files.newBufferedReader(File_Two)) {
int byt;
while ((byt = Content1.read()) != -1) {
if (byt != Content2.read()) {
return false;
}
}
}
return true;
} catch (IOException e) {
e.printStackTrace();
}
return false;
}
public static void main(String[] args) {
File File_One = new File("delftstack1.txt"); // Path to file one
File File_Two = new File("delftstack2.txt"); // Path to file two
boolean Comparison = CompareFilesbyByte(File_One.toPath(), File_Two.toPath());
if (Comparison) {
System.out.println("The Input files are equal.");
} else {
System.out.println("The Input files are not equal");
}
}
}
The code compares the files byte-by-byte. The output is:
The Input files are equal.
Line by Line Comparison of Two Files in Java
Similarly, the BufferedInputStream
is also used to read the file and compare each line of one file to each line of the other file. We use the readline()
method from BufferedReader
to read each line and compare it.
Let’s see an example.
package delftstack;
import java.io.BufferedReader;
import java.io.File;
import java.io.IOException;
import java.nio.file.Files;
import java.nio.file.Path;
import java.util.Arrays;
public class File_Compare {
private static boolean CompareFilesbyByte(Path File_One, Path File_Two) {
try {
long file_size = Files.size(File_One);
if (file_size != Files.size(File_Two)) {
return false;
}
if (file_size < 2048) {
return Arrays.equals(Files.readAllBytes(File_One), Files.readAllBytes(File_Two));
}
// Compare byte-by-byte
try (BufferedReader Content1 = Files.newBufferedReader(File_One);
BufferedReader Content2 = Files.newBufferedReader(File_Two)) {
String line;
while ((line = Content1.readLine()) != null) {
if (line != Content2.readLine()) {
return false;
}
}
}
return true;
} catch (IOException e) {
e.printStackTrace();
}
return false;
}
public static void main(String[] args) {
File File_One = new File("delftstack1.txt"); // Path to file one
File File_Two = new File("delftstack2.txt"); // Path to file two
boolean Comparison = CompareFilesbyByte(File_One.toPath(), File_Two.toPath());
if (Comparison) {
System.out.println("The Input files are equal.");
} else {
System.out.println("The Input files are not equal");
}
}
}
The code above compares two files line by line. As the content in the files is equal, the output is:
The Input files are equal.
Compare Two Files With Files.mismatch()
in Java
Recently Java 12 added the method Files.mismatch
in the java.nio.file.Files
class to compare two files.
This method takes files as inputs and compares the content of given files; it returns -1
if the two files are equals, and if not, it returns the position of the first mismatched byte.
We created a third file with different content. Let’s try an example to compare the different files.
package delftstack;
import java.io.File;
import java.io.IOException;
import java.nio.file.Files;
import java.nio.file.Path;
public class File_Compare {
public static void main(String[] args) throws IOException {
File File_One = new File("delftstack1.txt");
File File_Two = new File("delftstack2.txt");
File File_Three = new File("delftstack3.txt");
long Compare1 = Files.mismatch(File_One.toPath(), File_Two.toPath());
System.out.println(Compare1);
long Compare2 = Files.mismatch(File_Two.toPath(), File_Three.toPath());
System.out.println(Compare2);
}
}
The code above first compares two similar files which should return -1
and then compares two different files which should return the position of the first mismatched byte. See output:
-1
34
Compare Two Files With Apache Commons IO in Java
Apache Commons IO’s FileUtils
class is used to work with files. The contentEquals()
method can be used to compare two files which will return true
if the content of two files is equal or both files don’t exist.
Let’s try an example.
package delftstack;
import java.io.File;
import java.io.IOException;
import org.apache.commons.io.FileUtils;
public class File_Compare {
public static void main(String[] args) throws IOException {
File File_One = new File("delftstack1.txt");
File File_Two = new File("delftstack2.txt");
boolean Comapre_Result = FileUtils.contentEquals(File_One, File_Two);
if (Comapre_Result) {
System.out.println("The input files are equal.");
} else {
System.out.println("The input files are not equal.");
}
}
}
The code compares two files and checks if they are equal or not using the Apache FileUtils
class. See output:
The input files are equal.
Compare Two Files With Arrays.equals()
in Java
We can read the entire files into byte arrays and then compare these arrays for equality. We can use Files.readAllBytes()
to read the file into byte arrays and Arrays.equals()
to check their equality.
This method is not recommended for large files. See example:
package delftstack;
import java.io.File;
import java.io.IOException;
import java.nio.file.Files;
import java.nio.file.Path;
import java.util.Arrays;
public class File_Compare {
private static boolean CompareFileArrays(Path File_One, Path File_Two) {
try {
if (Files.size(File_One) != Files.size(File_Two)) {
return false;
}
byte[] First_File = Files.readAllBytes(File_One);
byte[] Second_File = Files.readAllBytes(File_Two);
return Arrays.equals(First_File, Second_File);
} catch (IOException e) {
e.printStackTrace();
}
return false;
}
public static void main(String[] args) {
File File_One = new File("delftstack1.txt");
File File_Two = new File("delftstack2.txt");
boolean Compare_Result = CompareFileArrays(File_One.toPath(), File_Two.toPath());
if (Compare_Result) {
System.out.println("The Input files are equal.");
} else {
System.out.println("The Input files are not equal.");
}
}
}
The code above reads the files into byte arrays and then compares those arrays. See output:
The Input files are equal.
Sheeraz is a Doctorate fellow in Computer Science at Northwestern Polytechnical University, Xian, China. He has 7 years of Software Development experience in AI, Web, Database, and Desktop technologies. He writes tutorials in Java, PHP, Python, GoLang, R, etc., to help beginners learn the field of Computer Science.
LinkedIn Facebook