java.lang.ClassCastException in Java
- What is java.lang.ClassCastException?
- Common Causes of ClassCastException
- How to Avoid ClassCastException
- Handling ClassCastException in Java
- Conclusion
- FAQ
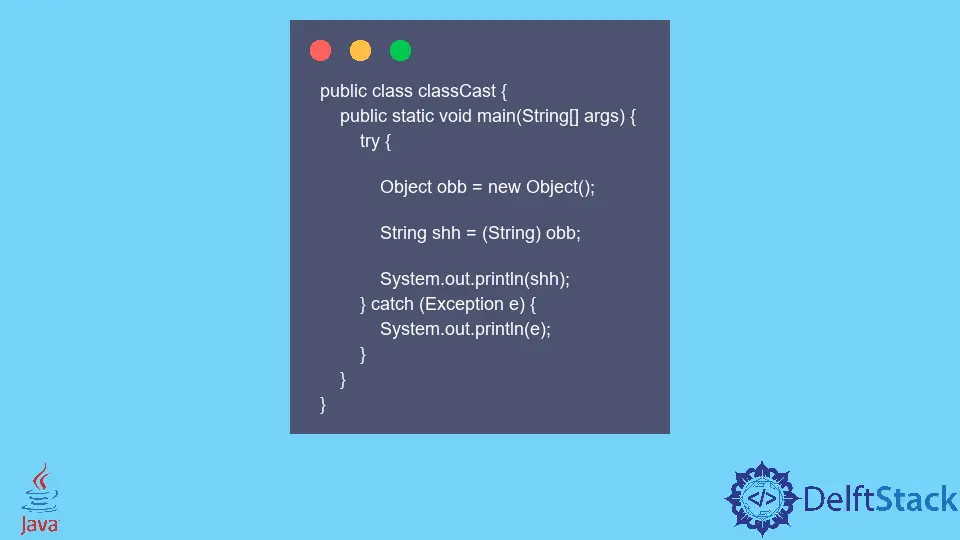
Java is a powerful programming language widely used for building applications, but it can be tricky at times. One common pitfall developers encounter is the java.lang.ClassCastException
. This exception occurs when you try to cast an object to a class of which it is not an instance. Understanding how to handle this exception is crucial for writing robust Java code.
In this article, we will explore the causes of ClassCastException
, how to avoid it, and best practices for handling it effectively. By the end, you’ll be better equipped to deal with this common issue and ensure smoother application performance.
What is java.lang.ClassCastException?
The java.lang.ClassCastException
is a runtime exception in Java that indicates an invalid cast operation. It occurs when you attempt to cast an object to a subclass of which it is not an instance. For example, if you have a class hierarchy where Animal
is a superclass and Dog
and Cat
are subclasses, trying to cast a Cat
object to a Dog
type will throw this exception.
This exception serves as a reminder of the importance of type safety in Java. Java’s strong typing system helps catch many errors at compile time, but ClassCastException
can still slip through during runtime. Understanding how to identify and fix these issues can save you from unexpected crashes and bugs in your applications.
Common Causes of ClassCastException
Several scenarios can lead to a ClassCastException
. Here are some common causes:
- Incorrect Type Casting: Attempting to cast an object to a type that it does not belong to.
- Using Generics Incorrectly: When working with collections, failing to use generics properly can lead to casting issues.
- Polymorphism Misunderstandings: Misunderstanding how polymorphism works can lead to incorrect assumptions about object types.
Recognizing these causes can help you avoid situations that lead to ClassCastException
.
How to Avoid ClassCastException
The best way to avoid ClassCastException
is to ensure that you are casting objects correctly. Here are some strategies to consider:
- Use
instanceof
Operator: Before casting an object, use theinstanceof
operator to check its type. - Generics: Utilize Java generics to enforce type checks at compile time.
- Code Reviews: Regular code reviews can help catch potential casting issues early in the development process.
By following these strategies, you can significantly reduce the chances of encountering a ClassCastException
.
Handling ClassCastException in Java
Even with the best practices in place, you may still encounter a ClassCastException
. Here are some methods to handle it effectively:
Method 1: Using try-catch Blocks
One straightforward way to handle ClassCastException
is by using a try-catch block. This allows your program to continue running even if a casting error occurs.
try {
Object obj = new Cat();
Dog dog = (Dog) obj; // This will throw ClassCastException
} catch (ClassCastException e) {
System.out.println("Caught a ClassCastException: " + e.getMessage());
}
In this example, we attempt to cast an object of type Cat
to Dog
, which results in a ClassCastException
. The catch block captures the exception and prints a message instead of crashing the program.
Output:
Caught a ClassCastException: Cat cannot be cast to Dog
Using try-catch blocks is a common practice for handling exceptions in Java. It allows developers to gracefully manage errors and provide feedback to users or logs without terminating the application unexpectedly.
Method 2: Validating Types with instanceof
Another effective way to handle potential ClassCastException
is to use the instanceof
operator. This operator checks whether an object is an instance of a specific class before performing a cast.
Object obj = new Cat();
if (obj instanceof Dog) {
Dog dog = (Dog) obj;
} else {
System.out.println("The object is not an instance of Dog.");
}
In this code snippet, we first check if obj
is an instance of Dog
. If it is, we proceed with the cast. If not, we print a message indicating that the cast cannot be performed.
Output:
The object is not an instance of Dog.
Using instanceof
is a proactive approach to prevent ClassCastException
. It allows you to validate the type before attempting the cast, ensuring that your code runs smoothly without unexpected crashes.
Method 3: Leveraging Generics
Generics can help you avoid ClassCastException
by enforcing type checks at compile time. When you use generics in collections, you can specify the type of objects they contain, reducing the chances of a casting error.
List<Dog> dogs = new ArrayList<>();
dogs.add(new Dog());
Object obj = dogs.get(0);
if (obj instanceof Dog) {
Dog dog = (Dog) obj;
} else {
System.out.println("The object is not an instance of Dog.");
}
In this example, we create a list of Dog
objects. When retrieving an object from the list, we check its type using instanceof
before casting. This ensures that we only cast objects of the correct type.
Output:
The object is not an instance of Dog.
By using generics, you can enforce type safety at compile time, which greatly reduces the likelihood of encountering ClassCastException
in your code.
Conclusion
In summary, java.lang.ClassCastException
is a common exception in Java that occurs when you attempt to cast an object to an incompatible type. By understanding its causes and implementing strategies to avoid it, such as using instanceof
, try-catch blocks, and generics, you can write more robust and error-free Java applications. Always remember that while exceptions are a part of programming, how you handle them can make a significant difference in the user experience and the reliability of your software.
FAQ
-
What is java.lang.ClassCastException?
java.lang.ClassCastException is a runtime exception that occurs when you try to cast an object to a type it does not belong to. -
How can I avoid ClassCastException in Java?
You can avoid ClassCastException by using the instanceof operator, utilizing generics, and performing regular code reviews. -
What happens if I do not handle ClassCastException?
If you do not handle ClassCastException, your application may crash, leading to poor user experience and potential data loss. -
Can ClassCastException be caught using try-catch?
Yes, ClassCastException can be caught using try-catch blocks, allowing your application to handle the error gracefully.
- Is using instanceof a good practice?
Yes, using instanceof before casting is a good practice as it helps ensure type safety and prevents runtime exceptions.
Shiv is a self-driven and passionate Machine learning Learner who is innovative in application design, development, testing, and deployment and provides program requirements into sustainable advanced technical solutions through JavaScript, Python, and other programs for continuous improvement of AI technologies.
LinkedInRelated Article - Java Error
- How to Fix the Error: Failed to Create the Java Virtual Machine
- How to Fix the Missing Server JVM Error in Java
- How to Fix the 'No Java Virtual Machine Was Found' Error in Eclipse
- How to Fix Javax.Net.SSL.SSLHandShakeException: Remote Host Closed Connection During Handshake
- How to Fix the Error: Failed to Create the Java Virtual Machine
- How to Fix Java.Lang.VerifyError: Bad Type on Operand Stack