Class Constant in Java
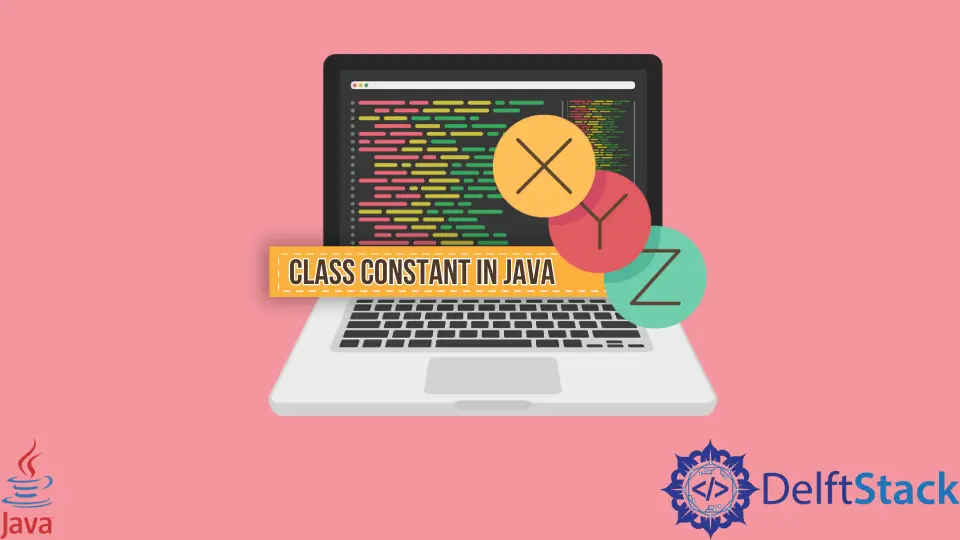
Constant means anything which we cannot change. In Java, static final
variables are considered class constants.
This tutorial demonstrates how to declare a class constant in Java.
Class Constant in Java
The final
keyword declares anything which cannot be modified later in Java. The class constants are static
variables in the class, declared as final.
The instance variables cannot be class constants because they are not static. The class constant is declared as static and final.
A static
keyword means that the field is available without first constructing an instance of the class. The example below demonstrates how to declare a class constant in Java.
Example:
package delftstack;
public class Delftstack {
// The Class constant
public static final String DEMO = "This is delftstack.com";
public static void main(String[] args) {
String Print = Delftstack.DEMO;
System.out.println(Print);
System.out.println(DEMO);
}
}
Output:
This is delftstack.com
This is delftstack.com
The code above created a class constant string, DEMO
, which can be used anywhere in the class.
Class constants are written in uppercase letters because when all letters are uppercase, camel notation is not possible. So, we used uppercase letters with underscores to separate the words.
Sheeraz is a Doctorate fellow in Computer Science at Northwestern Polytechnical University, Xian, China. He has 7 years of Software Development experience in AI, Web, Database, and Desktop technologies. He writes tutorials in Java, PHP, Python, GoLang, R, etc., to help beginners learn the field of Computer Science.
LinkedIn Facebook