How to Change the JLabel Text in Java Swing
Sheeraz Gul
Feb 02, 2024
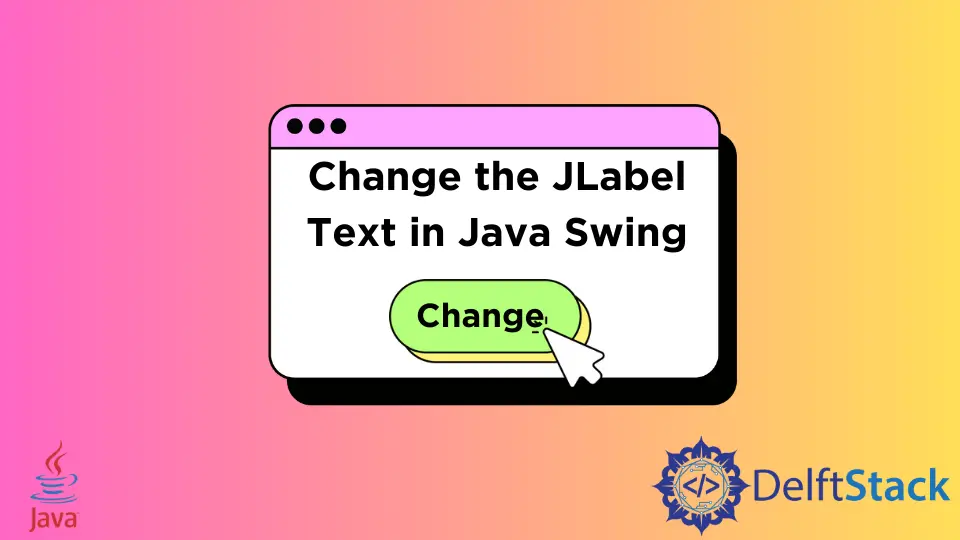
This tutorial demonstrates how to change the JLabel
text in Java swing.
Change the JLabel
Text in Java Swing
The method setText()
can be used to update the text of JLabel
in Swing. In Java, we create a frame where the label will be changed if we press the button.
Follow the steps below:
-
First of all, create a
JFrame
and set the size. -
Now, create the first label with the original text label.
-
Create a button to change the label.
-
Add an action listener to the button.
-
Add the setText method to the action listener and change the text for
JLabel
. -
Finally, run the program, and the
JLabel
text will be changed when the button is clicked.
Let’s implement the program in Java based on the steps above:
package delftstack;
import java.awt.BorderLayout;
import java.awt.event.ActionEvent;
import java.awt.event.ActionListener;
import javax.swing.*;
public class Change_Jlabel {
public static void main(String args[]) {
JFrame Demo_Frame = new JFrame("Demo Frame");
Demo_Frame.setLayout(new BorderLayout());
Demo_Frame.setDefaultCloseOperation(WindowConstants.EXIT_ON_CLOSE);
Demo_Frame.setSize(250, 100);
final JLabel J_Label = new JLabel("Original Label");
JButton J_Button = new JButton("Change Label");
J_Button.addActionListener(new ActionListener() {
@Override
public void actionPerformed(ActionEvent arg0) {
J_Label.setText("New Label");
}
});
Demo_Frame.add(J_Label, BorderLayout.NORTH);
Demo_Frame.add(J_Button, BorderLayout.CENTER);
Demo_Frame.setVisible(true);
}
}
See the output for the code above:
Author: Sheeraz Gul
Sheeraz is a Doctorate fellow in Computer Science at Northwestern Polytechnical University, Xian, China. He has 7 years of Software Development experience in AI, Web, Database, and Desktop technologies. He writes tutorials in Java, PHP, Python, GoLang, R, etc., to help beginners learn the field of Computer Science.
LinkedIn FacebookRelated Article - Java Swing
- How to Clear Text Field in Java
- How to Create Canvas Using Java Swing
- How to Use of SwingUtilities.invokeLater() in Java
- How to Center a JLabel in Swing
- Java Swing Date