Difference Between break and continue Statements in Java
-
Demonstrate the Difference Between Break and Continue Through
for
Loops in Java -
Demonstrate the Difference Between Break and Continue Through
foreach
Loops in Java - Demonstrate the Difference Between Break and Continue Through Nested Loops in Java
-
Demonstrate the Difference Between Labeled
break
and Labeledcontinue
Statements in Java -
Demonstrate the Use of
break
andcontinue
in theswitch
Condition in Java
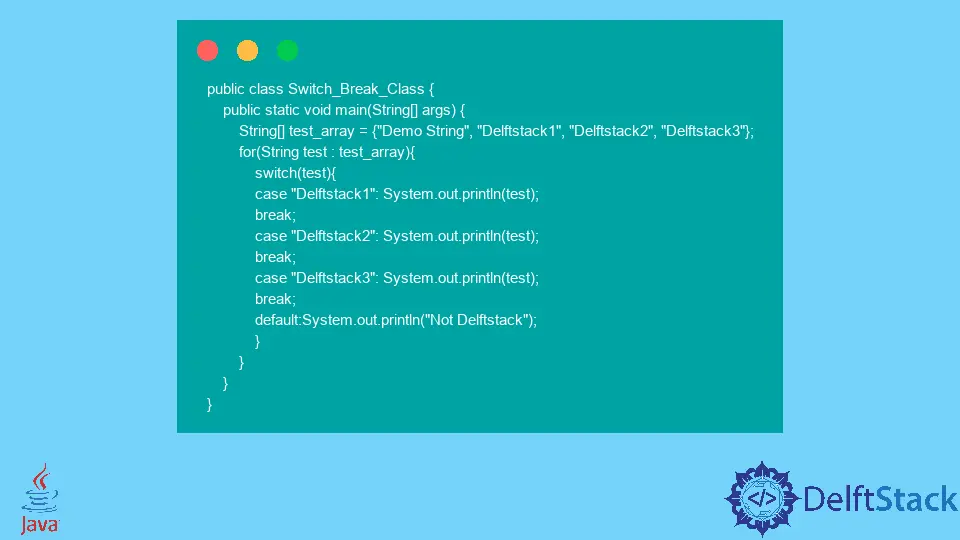
This tutorial will demonstrate the differences between Java’s break
and continue
statements.
The break
statement in Java is used to leave the loop at the given condition, whereas the continue
statement is used to jump an iteration of the loop on the given condition.
Demonstrate the Difference Between Break and Continue Through for
Loops in Java
See the example below to distinguish between break
and continue
using for
loops.
public class Break_Continue_Class {
public static void main(String args[]) {
System.out.println("The Break Statement: \n");
for (int x = 0; x < 15; x++) {
if (x == 8) {
break;
}
System.out.println(x);
}
System.out.println("The Continue Statement: \n");
for (int x = 0; x < 15; x++) {
if (x == 8) {
continue;
}
System.out.println(x);
}
}
}
Output:
The Break Statement:
0
1
2
3
4
5
6
7
The Continue Statement:
0
1
2
3
4
5
6
7
9
10
11
12
13
14
The loop with the break
statement terminates at 8, whereas the loop with continue
jumps the iteration at 8. It works similarly for while
and do-while
loops.
Demonstrate the Difference Between Break and Continue Through foreach
Loops in Java
See the example below to distinguish between break
and continue
statements for the foreach
loop.
public class Break_Continue_Class {
public static void main(String args[]) {
int[] test_array = {0, 1, 2, 3, 4, 5, 6, 7, 8, 9};
System.out.println("The Break statement works in this way: \n");
for (int test : test_array) {
if (test == 5) {
break;
}
System.out.print(test);
System.out.print("\n");
}
System.out.println("The Continue statement works in this way: \n");
for (int test : test_array) {
if (test == 5) {
continue;
}
System.out.print(test);
System.out.print("\n");
}
}
}
Output:
The Break statement works in this way:
0
1
2
3
4
The Continue statement works in this way:
0
1
2
3
4
6
7
8
9
As shown, the loop with the break
statement terminates at 5, and the loop with continue
jumps the iteration at 5.
Demonstrate the Difference Between Break and Continue Through Nested Loops in Java
The example below uses nested for
loops with the break
and continue
statements.
public class Break_Continue_Class {
public static void main(String[] args) {
System.out.println("The Break Statement: \n");
for (int x = 1; x <= 4; x++) {
for (int y = 1; y <= 4; y++) {
if (x == 3 && y == 3) {
break;
}
System.out.println(x + " " + y);
}
}
System.out.println("The Continue Statement: \n");
for (int x = 1; x <= 4; x++) {
for (int y = 1; y <= 4; y++) {
if (x == 3 && y == 3) {
continue;
}
System.out.println(x + " " + y);
}
}
}
}
Output:
The Break Statement:
1 1
1 2
1 3
1 4
2 1
2 2
2 3
2 4
3 1
3 2
4 1
4 2
4 3
4 4
The Continue Statement:
1 1
1 2
1 3
1 4
2 1
2 2
2 3
2 4
3 1
3 2
3 4
4 1
4 2
4 3
4 4
As we can see, the break
statement only breaks the inner loop where x and y both are 3, and the continue
statement just skipped one iteration where x and y both are 3.
Demonstrate the Difference Between Labeled break
and Labeled continue
Statements in Java
The unlabeled break
and continue
statements are only applied on the innermost loop in the nested loops. These labels are only used to apply the statement to our choice.
class Break_Continue_Labeled {
public static void main(String args[]) {
System.out.println("The Labeled Break Statement: ");
first_break: // First label
for (int x = 0; x < 4; x++) {
second_break: // Second label
for (int y = 0; y < 4; y++) {
if (x == 2 && y == 2) {
// Using break statement with label
break first_break;
}
System.out.println(x + " " + y);
}
}
System.out.println("The Labeled Continue Statement: ");
first_continue: // First label
for (int x = 0; x < 4; x++) {
second_continue: // Second label
for (int y = 0; y < 4; y++) {
if (x == 2 && y == 2) {
// Using break statement with label
continue first_continue;
}
System.out.println(x + " " + y);
}
}
}
}
Output:
The Labeled Break Statement:
0 0
0 1
0 2
0 3
1 0
1 1
1 2
1 3
2 0
2 1
The Labeled Continue Statement:
0 0
0 1
0 2
0 3
1 0
1 1
1 2
1 3
2 0
2 1
3 0
3 1
3 2
3 3
The code above uses labels as first and second. If we pass first as a parameter to the statement, it will be applied on the first statement, and if we pass second, it will be used on second.
Demonstrate the Use of break
and continue
in the switch
Condition in Java
Only the break
statement is used for the switch
condition; there is no use for the continue
statement.
public class Switch_Break_Class {
public static void main(String[] args) {
String[] test_array = {"Demo String", "Delftstack1", "Delftstack2", "Delftstack3"};
for (String test : test_array) {
switch (test) {
case "Delftstack1":
System.out.println(test);
break;
case "Delftstack2":
System.out.println(test);
break;
case "Delftstack3":
System.out.println(test);
break;
default:
System.out.println("Not Delftstack");
}
}
}
}
Output:
Not Delftstack
Delftstack1
Delftstack2
Delftstack3
The code above contains three cases and one default case, which shows the break
statement in switch conditions.
Sheeraz is a Doctorate fellow in Computer Science at Northwestern Polytechnical University, Xian, China. He has 7 years of Software Development experience in AI, Web, Database, and Desktop technologies. He writes tutorials in Java, PHP, Python, GoLang, R, etc., to help beginners learn the field of Computer Science.
LinkedIn Facebook