How to Break Out of a for Loop in Java
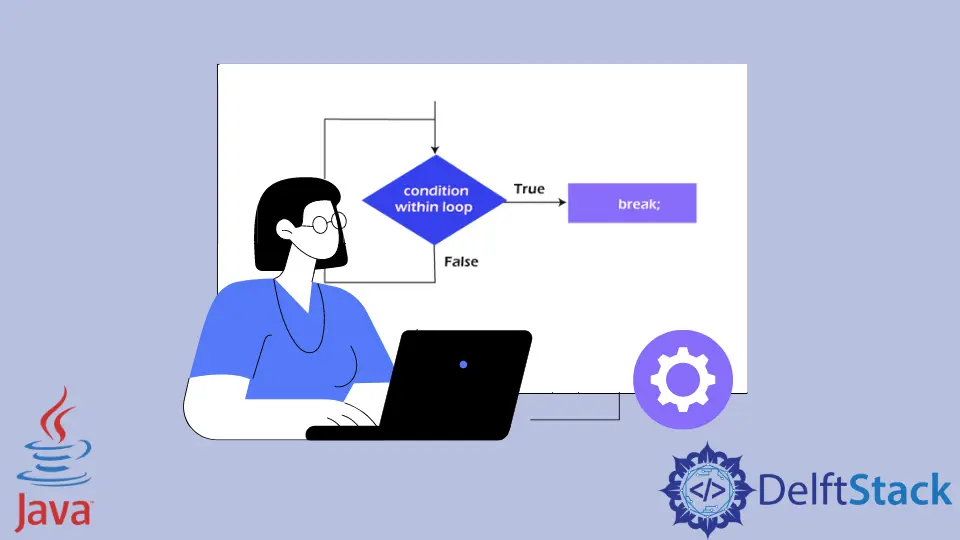
This guide will teach us how to break out of the for
loop in Java. In programming, certain conditions require breaking the for loop or any other loop for that matter. Let’s take a look.
Break Out of for
Loop in Java
The way to break the current iteration of the loop could not be more simple. You just need to use break
, and the program will jump out of that loop. The code example down below is self-explanatory.
public class Main {
public static void main(String[] args) {
// break statement is use to break loop at any point of iteration.
for (int i = 0; i < 10; i++) {
if (i == 5) {
break; // breaking 5th iteration
}
System.out.println(i);
}
}
}
Output:
0
1
2
3
4
As you can see, by simply writing the command break;
, we have stopped the iteration and jumped out of the loop.
Haider specializes in technical writing. He has a solid background in computer science that allows him to create engaging, original, and compelling technical tutorials. In his free time, he enjoys adding new skills to his repertoire and watching Netflix.
LinkedIn