How to Make a BMI Calculator in Java
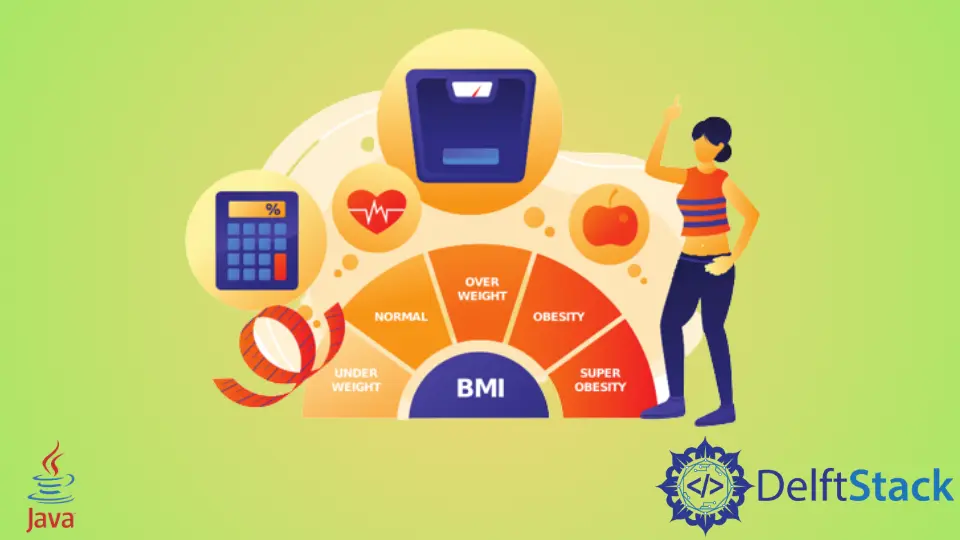
BMI stands for body mass index. This tutorial demonstrates how to create a BMI calculator in Java.
Make a BMI Calculator in Java
The Body Mass Index BMI is a measurement of health based on height and weight. The BMI is calculated by taking the weight in kilograms and dividing it by the square of height in meters.
The formula to take the BMI is:
BMI = (Weight in Kilograms) / (Height in Meters * Height in Meters)
The BMI has ranges which can be seen in the table below:
BMI Range | Category |
---|---|
> 30 | Obese |
25 – 30 | Overweight |
18.5 – 25 | Normal |
< 18.5 | Underweight |
Let’s implement the BMI index calculator in Java:
package delftstack;
import java.util.Scanner;
public class Calculate_BMI {
// method to check BMI
public static String BMIChecker(double Weight, double Height) {
// calculate the BMI
double BMI = Weight / (Height * Height);
// check the range of BMI
if (BMI < 18.5)
return "Underweight";
else if (BMI < 25)
return "Normal";
else if (BMI < 30)
return "Overweight";
else
return "Obese";
}
public static void main(String[] args) {
double Weight = 0.0f;
double Height = 0.0f;
String BMI_Result = null;
Scanner scan_input = new Scanner(System.in);
System.out.print("Please enter the weight in Kgs: ");
Weight = scan_input.nextDouble();
System.out.print("Pleae enter the height in meters: ");
Height = scan_input.nextDouble();
BMI_Result = BMIChecker(Weight, Height);
System.out.println(BMI_Result);
scan_input.close();
}
}
The code above will take inputs for the weight and height and then check the category of BMI. See output:
Please enter the weight in Kgs: 79
Please enter the height in meters: 1.86
Normal
Sheeraz is a Doctorate fellow in Computer Science at Northwestern Polytechnical University, Xian, China. He has 7 years of Software Development experience in AI, Web, Database, and Desktop technologies. He writes tutorials in Java, PHP, Python, GoLang, R, etc., to help beginners learn the field of Computer Science.
LinkedIn Facebook