How to Use assertTrue in Java
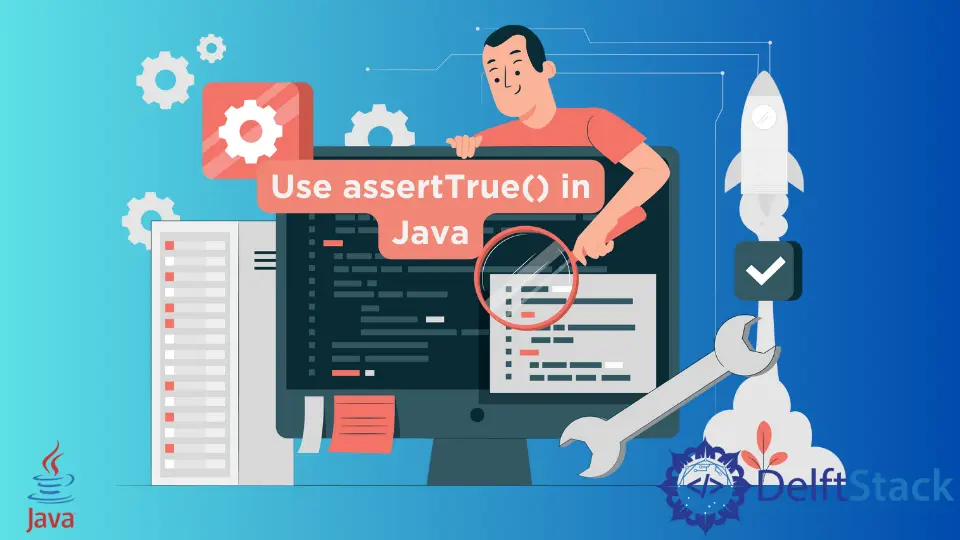
Java assertTrue()
is a function in the JUnit library used for testing purposes. JUnit minimizes the risk of negativity on our system.
The assertTrue()
function can evaluate a condition that runs on our system.
This tutorial will demonstrate how to use assetTrue()
in Java.
Use assertTrue()
to Evaluate a Condition in Java
First, we must import the JUnit library to use assertTrue()
. Download the JUnit jar file and add it to your libraries from Project Properties > Java Build Path > Libraries > Add External JAR
.
Below is example on how to use assertTrue()
.
package delftstack;
import static org.junit.Assert.*;
import org.junit.Test;
public class Assert_True {
public boolean ODD_Number(int number) {
boolean test = false;
if (number % 2 != 0) {
test = true;
}
return test;
}
@Test
public void ODD_Number_Test() {
Assert_True assert_test = new Assert_True();
assertTrue(assert_test.ODD_Number(2)); // 4
}
}
Output:
The code above checked if the number is odd or not. The assertTrue()
method ran a test in the IDE, in our case, Eclipse.
In the output for number 3, the test is passed, but for number 4, it failed. The failure trace will be something like this:
java.lang.AssertionError
at junit@4.10/org.junit.Assert.fail(Assert.java:92)
at junit@4.10/org.junit.Assert.assertTrue(Assert.java:43)
at junit@4.10/org.junit.Assert.assertTrue(Assert.java:54)
at Delftstack/delftstack.Assert_True.ODD_Number_Test(Assert_True.java:19)
Sheeraz is a Doctorate fellow in Computer Science at Northwestern Polytechnical University, Xian, China. He has 7 years of Software Development experience in AI, Web, Database, and Desktop technologies. He writes tutorials in Java, PHP, Python, GoLang, R, etc., to help beginners learn the field of Computer Science.
LinkedIn Facebook