How to Covert Array to Stream in Java
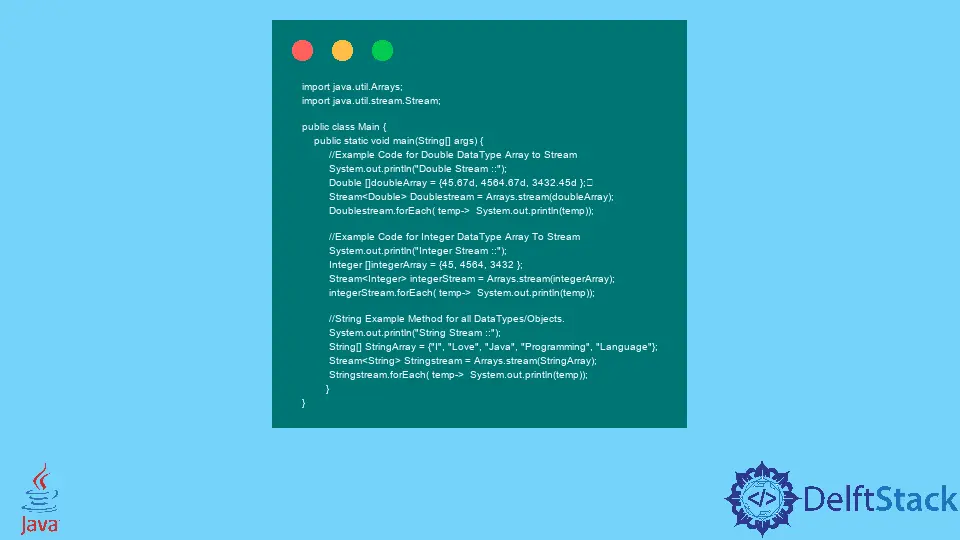
There are some static methods that allow you to convert an array to a stream in the Java programming language. The methods are implemented by the public class array. So these are the default methods that you can use to convert Arrays into streams. Let’s take a look.
Convert an Array to Stream in Java
The arrays could be of several types, for instance, double
, int
, long
, and so on. So, how do you convert them to stream? Well, there are separate methods for each data type of array. Let’s learn about them.
import java.util.Arrays;
import java.util.stream.Stream;
public class Main {
public static void main(String[] args) {
// Example Code for Double DataType Array to Stream
System.out.println("Double Stream ::");
Double[] doubleArray = {45.67d, 4564.67d, 3432.45d};
Stream<Double> Doublestream = Arrays.stream(doubleArray);
Doublestream.forEach(temp -> System.out.println(temp));
// Example Code for Integer DataType Array To Stream
System.out.println("Integer Stream ::");
Integer[] integerArray = {45, 4564, 3432};
Stream<Integer> integerStream = Arrays.stream(integerArray);
integerStream.forEach(temp -> System.out.println(temp));
// String Example Method for all DataTypes/Objects.
System.out.println("String Stream ::");
String[] StringArray = {"I", "Love", "Java", "Programming", "Language"};
Stream<String> Stringstream = Arrays.stream(StringArray);
Stringstream.forEach(temp -> System.out.println(temp));
}
}
Output:
Double Stream ::
45.67
4564.67
3432.45
Integer Stream ::
454564
3432
String Stream ::
I
LoveJava
Programming
Language
The first method is to convert a double type of array into a stream. Next up, we have an integer data type array, and in the end, we used the stream method for the string data type. As you can see, all the methods are implemented from the public class array.
Haider specializes in technical writing. He has a solid background in computer science that allows him to create engaging, original, and compelling technical tutorials. In his free time, he enjoys adding new skills to his repertoire and watching Netflix.
LinkedInRelated Article - Java Array
- How to Concatenate Two Arrays in Java
- How to Convert Byte Array in Hex String in Java
- How to Remove Duplicates From Array in Java
- How to Count Repeated Elements in an Array in Java
- Natural Ordering in Java
- How to Slice an Array in Java