How to Create Animation in Java
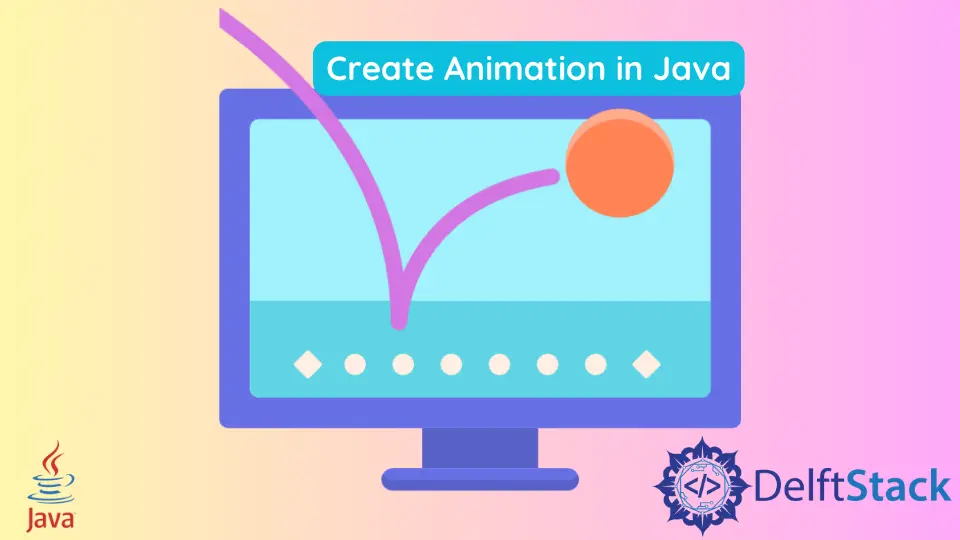
The JFrame class from the Java Swing library can be used to create different graphics and animations in Java. Until JDK 8 applet was used to create animations, but later it was deleted, and JAVA swing was added.
This tutorial will discuss and demonstrate creating an animation using Java.
Use the JFrame Class to Create Animation in Java
Using JFrame, we can decide colors, shapes, and timings for the animations. Let’s create a 2D rotating pie chart with three parts but six colors.
package delftstack;
import java.awt.Color;
import java.awt.Graphics;
import java.awt.Insets;
import java.util.Timer;
import java.util.TimerTask;
import javax.swing.JFrame;
public class Animation extends JFrame {
private static int DELAY = 500; // The speed on which the animation will run
Insets animate_insets;
Color colors[] = {Color.PINK, Color.GREEN, Color.ORANGE, Color.BLACK, Color.WHITE, Color.MAGENTA};
public void paint(Graphics demo) {
super.paint(demo);
if (animate_insets == null) {
animate_insets = getInsets();
}
int a = animate_insets.left;
int b = animate_insets.top;
int animation_width = getWidth() - animate_insets.left - animate_insets.right;
int animation_height = getHeight() - animate_insets.top - animate_insets.bottom;
int animation_start = 0;
int animation_steps = colors.length;
int animation_stepSize = 720 / animation_steps;
synchronized (colors) {
for (int i = 0; i < animation_steps; i++) {
demo.setColor(colors[i]);
demo.fillArc(a, b, animation_width, animation_height, animation_start, animation_stepSize);
animation_start += animation_stepSize;
}
}
}
public void go() {
TimerTask animation_task = new TimerTask() {
public void run() {
Color animation_color = colors[0];
synchronized (colors) {
System.arraycopy(colors, 1, colors, 0, colors.length - 1);
colors[colors.length - 1] = animation_color;
}
repaint();
}
};
Timer animation_timer = new Timer();
animation_timer.schedule(animation_task, 0, DELAY);
}
public static void main(String args[]) {
Animation Demo_Animation = new Animation();
Demo_Animation.setSize(400, 400);
Demo_Animation.show();
Demo_Animation.go();
}
}
The code above will generate a rotating pie chart with three parts and six different colors.
Output:
We can create animations with different shapes and colors using the Java swing library.
Sheeraz is a Doctorate fellow in Computer Science at Northwestern Polytechnical University, Xian, China. He has 7 years of Software Development experience in AI, Web, Database, and Desktop technologies. He writes tutorials in Java, PHP, Python, GoLang, R, etc., to help beginners learn the field of Computer Science.
LinkedIn Facebook