How to Show Animated GIF in Java
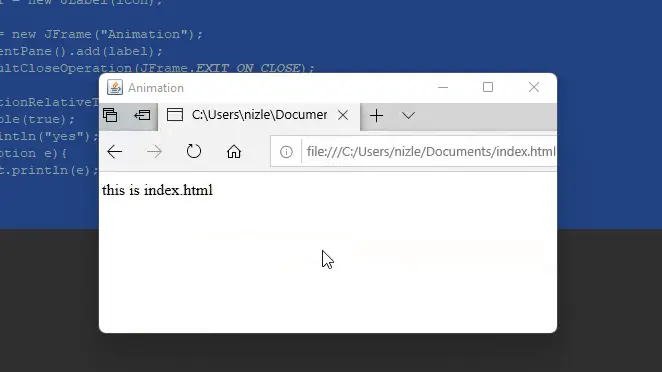
We can use the Swing
library methods of the javax
package to show the animated GIFs in Java. This article introduces how users can show animated GIFs in Java applications or separate windows.
Use the Javax.swing
Library to Show Animated GIFs in Java
In the below example code, we have imported the required libraries. We use the jLabel
and jFrame
classes of the javax.swing
library.
Also, we are using the URL
class from the Java.net
library. To read the GIF from the URL, we created the object of the URL
class and passed the location URL of the GIF as an argument.
From that URL, we have created the image icon using the object of the ImageIcon
class.
Next, we created the jLabel
from the Imageicon
. Now, we will create a frame to display the jLabel
.
After that, we added the label
to the frame to show the GIF. At last, we have set the visible
to true using the frame.setVisible(true)
.
Example Code:
// import required libraries
import java.net.*;
import javax.swing.*;
public class TestClass {
public static void main(String[] args) {
try {
// create a new URL from the image URL
URL ImageUrl =
new URL("https://www.delftstack.com/img/HTML/page%20redirects%20to%20index.gif");
// Create image icon from URL
Icon imageIcon = new ImageIcon(ImageUrl);
// Create a new JLabel from the icon
JLabel label = new JLabel(imageIcon);
// Create a new JFrame to append the icon
JFrame frame = new JFrame("Animation");
// add a label to JFrame
frame.getContentPane().add(label);
frame.setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);
frame.pack();
frame.setLocationRelativeTo(null);
// Show the JFrame
frame.setVisible(true);
} catch (Exception e) {
System.out.println(e);
}
}
}
Output:
In the above output, users can see a new window pop-up showing the animated GIF.
Also, if users want to show the GIF from the local computer, they can give the path of the GIF while initializing the object of ImageIcon
as shown in the code below.
Icon imageIcon = new ImageIcon("<path of GIF from local computer");
We don’t need to create the URL
class object if we show the GIF from the local computer.
Furthermore, users can customize the size of the window frame of a GIF and set custom labels for the frame according to their needs.