The abs() Method in Java
- What Is the Absolute Value in Java
-
Use the
abs()
Method to Find the Absolute Value of a Number in Java
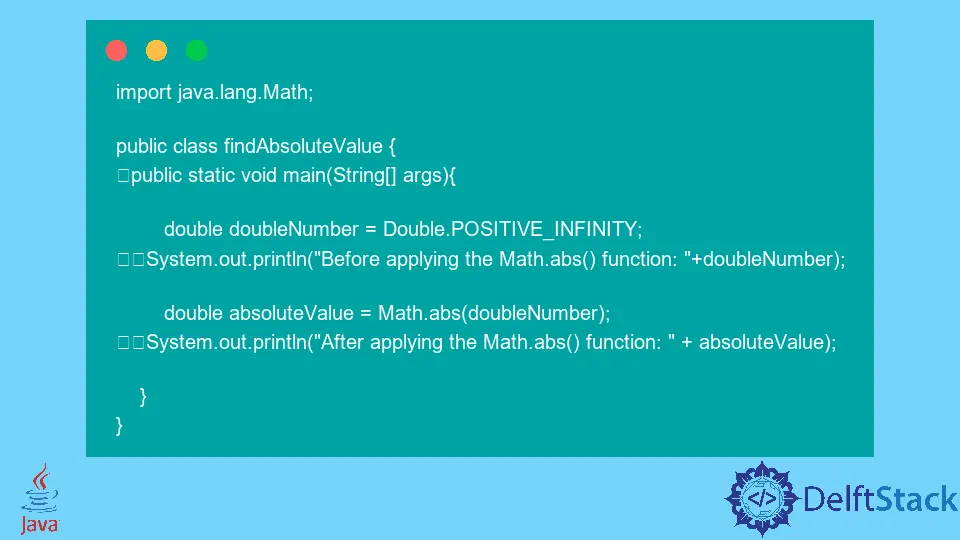
We will learn about the abs()
method in Java to find the absolute value of a specified number. We will be learning by writing and practicing various code examples.
What Is the Absolute Value in Java
Absolute value means a non-negative value of the specified number. For instance, the absolute value of -4 is 4.
We use the abs()
method of the java.lang.Math
package. It takes only one argument of type int
, double
, float
, or long
and returns its non-negative (absolute) value.
The following are some rules of thumb that you must remember to be an expert while finding an absolute value of a given number.
- If the argument’s data type is
float
ordouble
:
1.1 Theabs()
returns the positive value; it does not matter if the passed argument is positive or negative.
1.2 It results asPOSITIVE_INFINITY
if we passInfinity
as an argument.
1.3 It returnsNaN
if its argument isNaN
.
1.4 It returns a positive zero if theabs()
method gets a negative or positive zero. - If the argument’s data type is
long
orint
:
2.1 If the value ofLong.MIN_VALUE
orInteger.MIN_VALUE
is equal to theabs()
method’s argument, the output would be the same, a negative value.
Use the abs()
Method to Find the Absolute Value of a Number in Java
In this section, we will be writing different code examples to practice all the rules that are given above.
Example Code (when a negative number is passed as an argument):
import java.lang.Math;
public class findAbsoluteValue {
public static void main(String[] args) {
int intNumber = -8;
System.out.println("Before applying the Math.abs() function: " + intNumber);
int absoluteValue = Math.abs(intNumber);
System.out.println("After applying the Math.abs() function: " + absoluteValue);
}
}
Output:
Before applying the Math.abs() function: -8
After applying the Math.abs() function: 8
Inside the main
method, we declare and initialize a variable to hold a value further passed to the Math.abs()
function to calculate a given number’s absolute value (positive value).
We print the value of a number before and after applying the Math.abs()
function. This same process will be followed in the upcoming examples, but the variable name and type will be changed.
Example Code (when a positive number is passed as an argument):
import java.lang.Math;
public class findAbsoluteValue {
public static void main(String[] args) {
int intNumber = 8;
System.out.println("Before applying the Math.abs() function: " + intNumber);
int absoluteValue = Math.abs(intNumber);
System.out.println("After applying the Math.abs() function: " + absoluteValue);
}
}
Output:
Before applying the Math.abs() function: 8
After applying the Math.abs() function: 8
Example Code (when infinity
is passed as an argument):
import java.lang.Math;
public class findAbsoluteValue {
public static void main(String[] args) {
double doubleNumber = Double.POSITIVE_INFINITY;
System.out.println("Before applying the Math.abs() function: " + doubleNumber);
double absoluteValue = Math.abs(doubleNumber);
System.out.println("After applying the Math.abs() function: " + absoluteValue);
}
}
Output:
Before applying the Math.abs() function: Infinity
After applying the Math.abs() function: Infinity
Example Code (when NaN
is passed as an argument):
import java.lang.Math;
public class findAbsoluteValue {
public static void main(String[] args) {
double doubleNumber = Double.NaN;
System.out.println("Before applying the Math.abs() function: " + doubleNumber);
double absoluteValue = Math.abs(doubleNumber);
System.out.println("After applying the Math.abs() function: " + absoluteValue);
}
}
Output:
Before applying the Math.abs() function: NaN
After applying the Math.abs() function: NaN
Example Code (when positive zero or negative zero is passed as an argument):
import java.lang.Math;
public class findAbsoluteValue {
public static void main(String[] args) {
int number, absoluteValue;
number = -0;
System.out.println("Before applying the Math.abs() function: " + number);
absoluteValue = Math.abs(number);
System.out.println("After applying the Math.abs() function: " + absoluteValue);
number = 0;
System.out.println("Before applying the Math.abs() function: " + number);
absoluteValue = Math.abs(number);
System.out.println("After applying the Math.abs() function: " + absoluteValue);
}
}
Output:
Before applying the Math.abs() function: 0
After applying the Math.abs() function: 0
Before applying the Math.abs() function: 0
After applying the Math.abs() function: 0
Example Code (when Long.MIN_VALUE
or Integer.MIN_VALUE
is passed as an argument):
import java.lang.Math;
public class findAbsoluteValue {
public static void main(String[] args) {
long longNumber = Long.MIN_VALUE;
System.out.println("Before applying the Math.abs() function: " + longNumber);
long longAbsVal = Math.abs(longNumber);
System.out.println("After applying the Math.abs() function: " + longAbsVal);
int intNumber = Integer.MIN_VALUE;
System.out.println("Before applying the Math.abs() function: " + intNumber);
int intAbsVal = Math.abs(intNumber);
System.out.println("After applying the Math.abs() function: " + intAbsVal);
}
}
Output:
Before applying the Math.abs() function: -9223372036854775808
After applying the Math.abs() function: -9223372036854775808
Before applying the Math.abs() function: -2147483648
After applying the Math.abs() function: -2147483648