Invalid Character Constant in Java
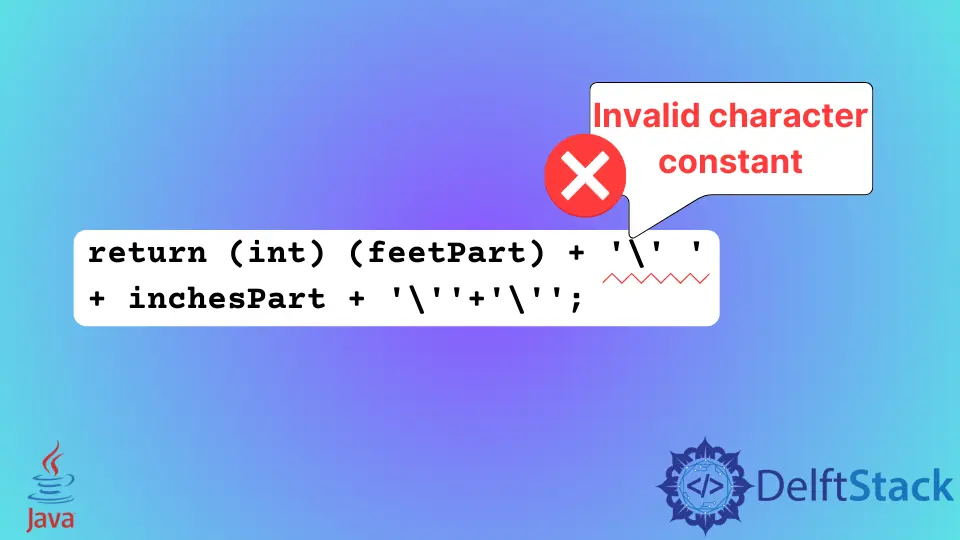
This Java tutorial will discuss invalid character constants. But before we can do that, we need to understand the character constant.
Java Character Constant
A single character constant, also known as a character constant, is a single character encapsulated in a pair of ''
or single quotes.
For instance, the following are examples of single-character constants:
'a'
,'b'
, and'c'
'1'
,'4'
, and'7'
''
a null string
One thing that should be brought to your attention is that the character constant 'a'
is not the same as a
.
Solve the Invalid character constant
Error in Java
Now that we have that out of the way, let us discuss the invalid character constant with the help of an example to understand it better.
Let’s say we want to display height in feet and inches and use the client side using the same server side. However, when we execute the following line of code, we get the error message: Invalid character constant
.
return (int) (feetPart) + '\' ' + inchesPart + '\'' + '\'';
Take a look at the potential solution to this problem now.
This particular character '\' '
is the source of the error. Because this is an attempt to specify a character literal, which is comprised of two characters, including an apostrophe and a space thus, we cannot accept it.
A character literal can only contain a single character at a time. If we want to define an apostrophe space, we should use a string literal instead when the apostrophe doesn’t need to be escaped because it will already be in the string.
"' "
The entire statement would work more effectively as:
return (int) (feetPart) + "' " + inchesPart + "''";
Alternatively, to indicate inches use the "
(one double quotation mark) instead of the ''
(two single quotation marks).
return (int) feetPart + "' " + inchesPart + "\"";
Therefore, now that we know that single quotation marks indicate a single character, using single quotation marks outside of multiple characters will undoubtedly result in an error.
On the other hand, double quotation marks indicate a string, meaning numerous character strings should be used whenever possible. It is also possible to wrap a single character in double quotes, changing its significance to that of a string.
I have been working as a Flutter app developer for a year now. Firebase and SQLite have been crucial in the development of my android apps. I have experience with C#, Windows Form Based C#, C, Java, PHP on WampServer, and HTML/CSS on MYSQL, and I have authored articles on their theory and issue solving. I'm a senior in an undergraduate program for a bachelor's degree in Information Technology.
LinkedInRelated Article - Java Error
- How to Fix the Error: Failed to Create the Java Virtual Machine
- How to Fix the Missing Server JVM Error in Java
- How to Fix the 'No Java Virtual Machine Was Found' Error in Eclipse
- How to Fix Javax.Net.SSL.SSLHandShakeException: Remote Host Closed Connection During Handshake
- How to Fix the Error: Failed to Create the Java Virtual Machine
- How to Fix Java.Lang.VerifyError: Bad Type on Operand Stack