Minimum and Maximum Value of Integer in Java
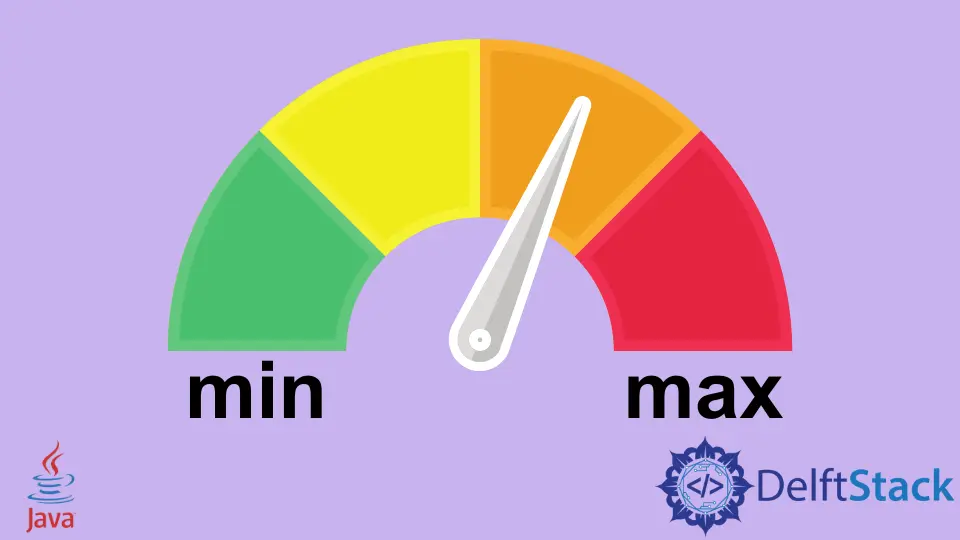
This tutorial introduces the minimum and maximum values of Integers in Java.
The Integer is a wrapper class in Java that is used to create integer objects in Java.
Since Java works with objects only, so we can use this class for creating integer objects. Java allows primitive int that holds the numeric values, and we need to convert it into an object where an object is required.
This article will teach us to get the minimum and maximum value of Integer supported by Java JVM. However, Integer uses 4 bytes in the memory. Let’s understand with the examples.
Min and Max Value of Integer in Java
To get the minimum and maximum value, the Java Integer class provides MIN_VALUE
and MAX_VALUE
constants. These constants hold the values that an Integer object can hold as min and max values.
See the example below, where we used these constants and printed the values.
public class SimpleTesting {
public static void main(String[] args) {
int a = Integer.MAX_VALUE;
System.out.println("max value " + a);
int b = Integer.MIN_VALUE;
System.out.println("min value " + b);
}
}
Output:
max value 2147483647
min value -2147483648
Overflow and Underflow in Java Integer
The range of Java Integer can be fetched by using the constants, and Java is pretty smart to handle the overflow and underflow conditions.
For example, what will happen if we store an integer value more than the max value? In this case, Java automatically assigns a negative value based on the value.
The integer values assignment circularly takes place. If we pass any value less than the min value, the value will convert to a positive value.
See the example below.
public class SimpleTesting {
public static void main(String[] args) {
int a = Integer.MAX_VALUE;
System.out.println("max value " + a);
a += 1;
System.out.println("max value incremented by 1 " + a);
int b = Integer.MIN_VALUE;
System.out.println("min value " + b);
b -= 1;
System.out.println("min value incremented by 1 " + b);
}
}
Output:
max value 2147483647
max value incremented by 1 -2147483648
min value -2147483648
min value incremented by 1 2147483647