How to Solve the Int Cannot Be Dereferenced Error in Java
- Dereferencing in Java
-
Cause of
int cannot be dereferenced
in Java -
Solve the
int cannot be dereferenced
Error in Java
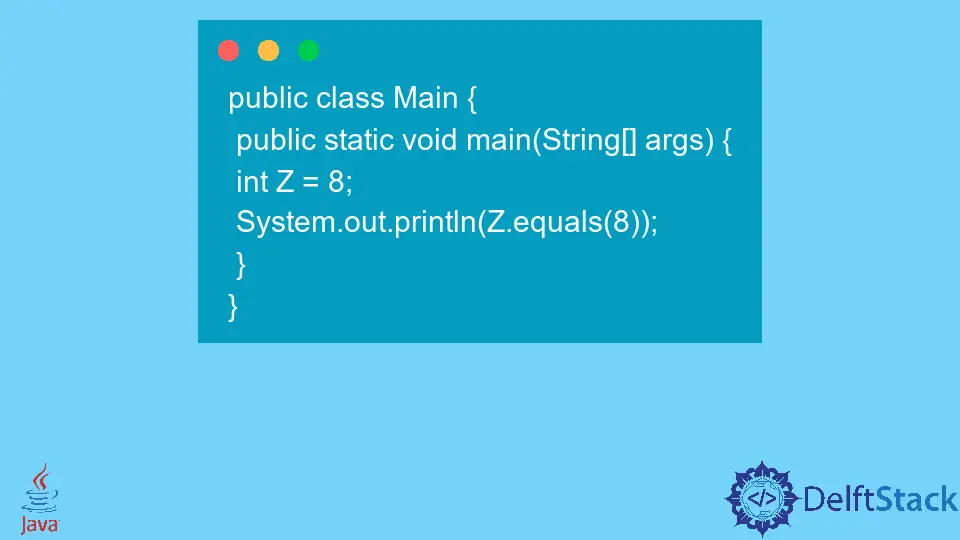
In this article, we’ll discuss the cause of the Java int cannot be dereferenced
exception and how to fix it. First, have a look at what dereferencing in Java is.
Dereferencing in Java
Primitive and object variables are the two types of variables that can be used in Java. However, only object variables can be reference types. The int data type is a primitive rather than an object.
Accessing the value that is pointed to by a reference is what’s known as dereferencing. It is impossible to dereference int because it is already a value and is not a reference to anything else.
Cause of int cannot be dereferenced
in Java
- A reference in Java is an address that points to a specific object or variable. The term dereferencing refers to obtaining an object’s properties through a reference.
- If you try to perform any dereferencing on a primitive, like the below example, you will receive the error
Z
cannot be dereferenced, whereZ
is a type considered primitive. - The cause for this is that primitives are not the same thing as objects; instead, they are raw value representations.
Solve the int cannot be dereferenced
Error in Java
Let’s understand int cannot be dereferenced
and figure out how to fix it with the help of the example below:
Example:
public class Main {
public static void main(String[] args) {
int Z = 8;
System.out.println(Z.equals(8));
}
}
Firstly, we created the Main
class and compared an int to a value chosen at random. Z
is one of Java’s eight fundamental data types, including int, byte, short, etc.
We will see the following error when we attempt to compile the code:
Main.java:5: error: int cannot be dereferenced
System.out.println(Z.equals(8));
^
1 error
In our case, we need to determine whether or not the two values are equal. Solving our issue is to utilize the notation ==
rather than the equals()
function for primitive types:
public class Main {
public static void main(String[] args) {
int Z = 8;
System.out.println(Z == 8);
}
}
The following output will be printed when we compile the code:
true
I have been working as a Flutter app developer for a year now. Firebase and SQLite have been crucial in the development of my android apps. I have experience with C#, Windows Form Based C#, C, Java, PHP on WampServer, and HTML/CSS on MYSQL, and I have authored articles on their theory and issue solving. I'm a senior in an undergraduate program for a bachelor's degree in Information Technology.
LinkedInRelated Article - Java Error
- How to Fix the Error: Failed to Create the Java Virtual Machine
- How to Fix the Missing Server JVM Error in Java
- How to Fix the 'No Java Virtual Machine Was Found' Error in Eclipse
- How to Fix Javax.Net.SSL.SSLHandShakeException: Remote Host Closed Connection During Handshake
- How to Fix the Error: Failed to Create the Java Virtual Machine
- How to Fix Java.Lang.VerifyError: Bad Type on Operand Stack