How to Instantiate an Object in Java
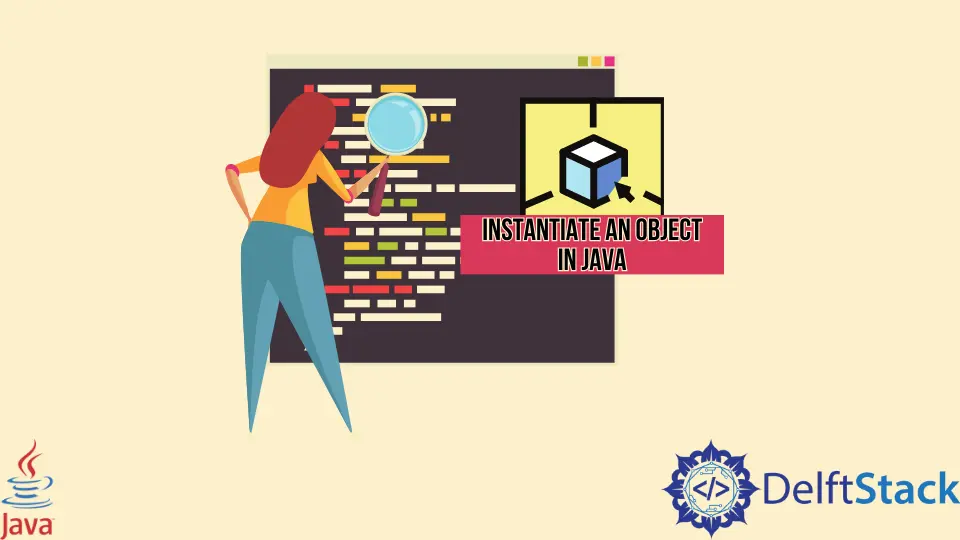
In Java, an object is referred to as an instance of a class. For example, let us assume a class named car
, then SportsCar
, SedanCar
, StationWagon
, etc., can be considered the objects of this class.
In this tutorial, we will discuss how to instantiate objects in Java.
Using the new
keyword, we can create instances of a class in Java. Remember that we do not instantiate methods in Java as objects are instances of classes and not methods. A method is just a behavior that the class possesses.
By creating an object of one class, we can access its public methods through another class. Like in the code below, we create an instance of a second class in the first class and then use the second class methods in the first class.
// creating a class named first
public class First {
public static void main(String[] args) {
Second myTest = new Second(1, 2); // instantiating an object of class second
int sum = myTest.sum(); // using the method sum from class second
System.out.println(sum);
}
}
// creating a class named second
class Second {
int a;
int b;
Second(int a, int b) {
this.a = a;
this.b = b;
}
public int sum() {
return a + b;
}
}
Output:
3
If we wish to access the methods from one class in another method of the same class, it is unnecessary to instantiate an object if the method is declared static
.
For example,
public class Testing {
private static int sample(int a, int b) {
return a + b;
}
public static void main(String[] args) {
int c = sample(1, 2); // method called
System.out.println(c);
}
}
Output:
3
In the above example, we can call for the method sample()
as both the methods are of the same class, and sample()
is declared static
, so no object is required.
But we can still carry out the object instantiation if both methods are of the same class, as illustrated below. It is done if the method is not declared static
.
See the code below.
public class Testing {
private int Sample() {
int a = 1;
int b = 2;
int c = a + b;
return c;
}
public static void main(String[] args) {
Testing myTest = new Testing();
int result = myTest.Sample();
System.out.println(result);
}
}
Output:
3