How to Instance of a Class in Java
-
Using the
new
Keyword to Create an Instance of a Class in Java -
Using the
instanceof
Operator to Check the Given Type of a Class
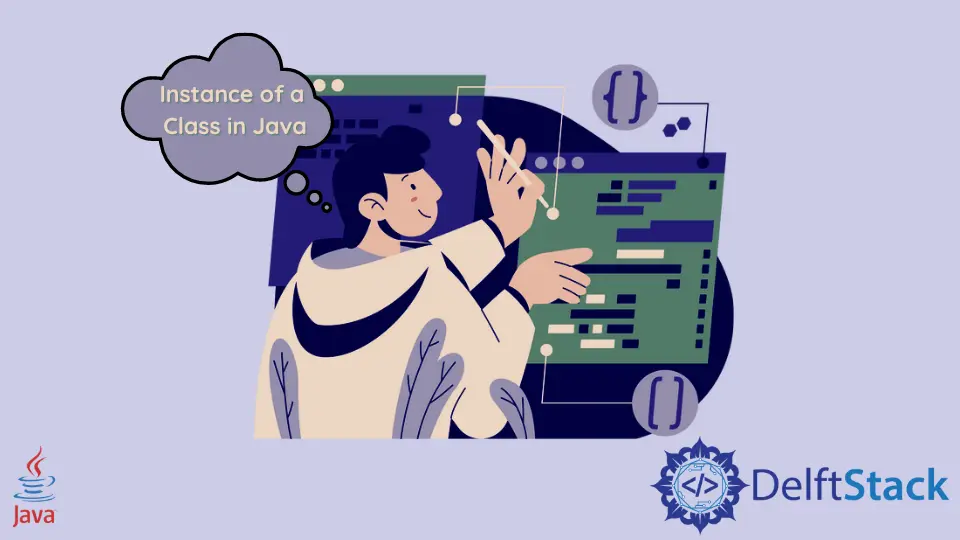
In Java, Class and Object are the basic concepts of Object-Oriented Programming.
Class is a blueprint from which objects are created. Instances in Java are known as Objects. An object is a real-life entity, whereas a Class is a group of similar objects.
Using the new
Keyword to Create an Instance of a Class in Java
An object is created from the class. Dog
is a class that is a real-life entity.
Basically, instance
and object
are the same thing. We create an instance
of the Dog
class using the new
keyword.
A call to the constructor after the new
keyword creates an object and initializes the new class. Hence instance
of the Dog
class.
public class Dog {
public Dog(String name) {
System.out.println("Dog,s name is : " + name);
}
public static void main(String[] args) {
Dog dog = new Dog("Scooby");
}
}
Output:
Dog,s name is : Scooby
Using the instanceof
Operator to Check the Given Type of a Class
In Java, instanceof
is a comparison operator is used to check whether an instance is of a specified type or not and returns boolean true or false. If we compare instance
with a null
type using the instaneof
operator, it returns false.
Here, we have Dog
as a child class which extends the Animal
class. The Dog
class has a constructor which takes three string
type variables name
, color
, and breed
, respectively.
We created an instance of the Dog
class using the new
keyword.
An object of a subtype is also a type of parent class. If we check if the instance
dog
is of type Animal, then the instanceof
operator returns true
as Dog extends Animal class. Hence dog
can be referred to as an instance of Dog
or Animal
class.
If we compare the dog1
instance that is null as a type of Dog
class, then instanceof
returns false.
class Animal {}
public class Dog extends Animal {
public Dog(String name, String color, String breed) {
System.out.println(name + " is a " + color + " colored " + breed + " Dog");
}
public static void main(String[] args) {
Dog dog = new Dog("Scooby", "brown", "Labrador");
Dog dog1 = null;
System.out.println(dog instanceof Dog);
System.out.println(dog instanceof Animal);
System.out.println(dog1 instanceof Dog);
}
}
Output:
Scooby is a brown colored Labrador Dog
true
true
false
Rupam Saini is an android developer, who also works sometimes as a web developer., He likes to read books and write about various things.
LinkedIn