How to Get a Char From the Input in Java
-
Get a Char From the Input Using
Scanner.next().charAt(0)
in Java -
Get a Char From the Input Using
System.in.read()
in Java -
Get a Char From the Input Using
InputStreamReader()
in Java
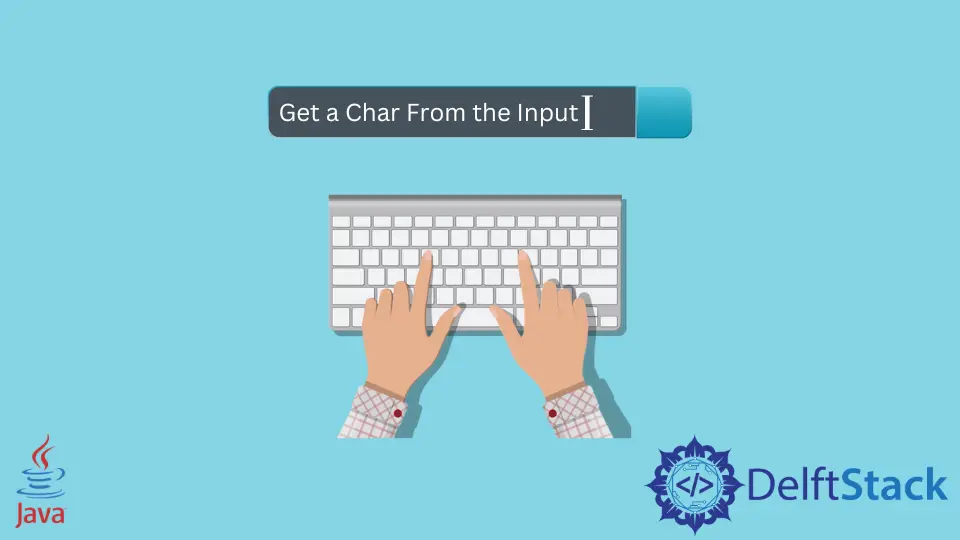
This article will introduce several methods that we can use to input a character in Java. We can input and read a whole sentence in Java, but there are very few ways to read a single character. The following examples show a few ways and how to use them.
Get a Char From the Input Using Scanner.next().charAt(0)
in Java
In the first example, we will use the Scanner
class to take the input. We use scanner.next().charAt(0)
to read the input as char
. charAt(0)
reads read the first character from the scanner.
import java.util.Scanner;
public class InputChar {
public static void main(String[] args) d {
Scanner scanner = new Scanner(System.in);
System.out.println("Please input a character: ");
char value = scanner.next().charAt(0);
System.out.println("Character: " + value);
}
}
Output:
Please input a character:
h
Character: h
Get a Char From the Input Using System.in.read()
in Java
The next example uses the System.in
directly to call the read()
method. System.in.read()
reads one byte and returns an int
. As every character represents a number we can convert the int
to a character and vice versa.
Below, we read the input using System.in.read()
and then cast it to a char
to convert it into a character type.
import java.io.IOException;
public class InputChar {
public static void main(String[] args) throws IOException {
System.out.println("Please input a character: ");
char value = (char) System.in.read();
System.out.println("Character: " + value);
}
}
Output:
Please input a character:
j
Character: j
Get a Char From the Input Using InputStreamReader()
in Java
Another method similar to the previous one uses an InputStreamRead()
that provides the read()
method just like System.in
. We can use this read()
method to input the character that will return an int
and then cast it to a char
as we have done in the example below.
import java.io.IOException;
import java.io.InputStreamReader;
import java.io.Reader;
public class InputChar {
public static void main(String[] args) throws IOException {
System.out.println("Please input a character: ");
Reader reader = new InputStreamReader(System.in);
int characterAsInt = reader.read();
char character = (char) characterAsInt;
System.out.println("Character: " + character);
}
}
Output:
Please input a character:
/
Character: /
Rupam Saini is an android developer, who also works sometimes as a web developer., He likes to read books and write about various things.
LinkedInRelated Article - Java Scanner
- How to Fix Java Error: Scanner NextLine Skips
- Difference Between next() and nextLine() Methods From Java Scanner Class
- How to Get a Keyboard Input in Java
- Press Enter to Continue in Java
- How to Clear Scanner in Java