How to Initialise Boolean Variable in Java
- Initialising a Boolean Variable Directly
- Initialising a Boolean Variable Using Conditional Expressions
- Initialising a Boolean Variable with Method Return Values
- Initialising a Boolean Variable from User Input
- Conclusion
- FAQ
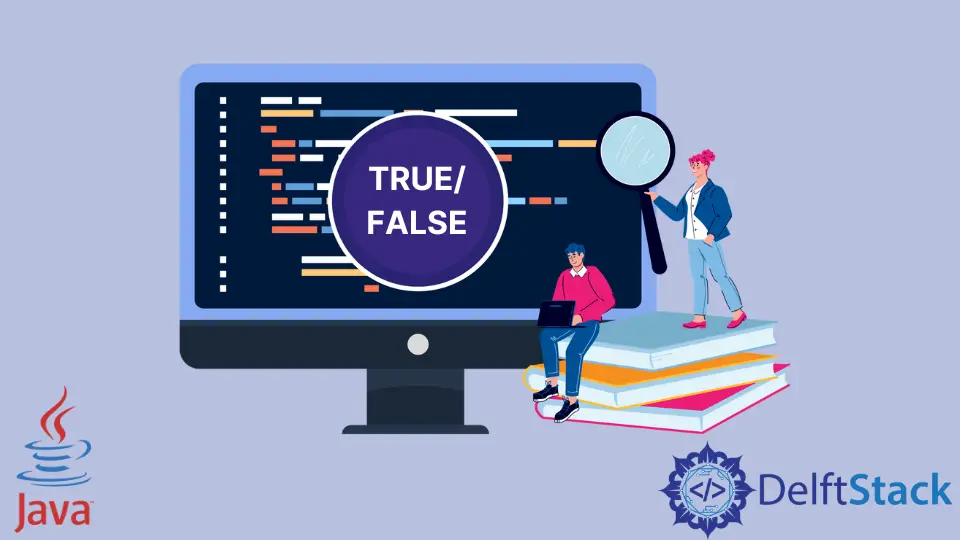
In the world of programming, understanding how to handle data types effectively is crucial. One of the most fundamental data types in Java is the boolean variable, which can hold only two values: true or false. Initialising boolean variables properly is essential for writing clean and efficient code. Whether you’re a beginner or an experienced developer, knowing how to initialise boolean variables in Java can help you avoid common pitfalls and enhance your programming skills.
In this article, we’ll explore various methods to initialise boolean variables, complete with code examples and detailed explanations. Let’s dive into the world of Java booleans!
Initialising a Boolean Variable Directly
The simplest way to initialise a boolean variable in Java is by assigning it a value directly. This method is straightforward and often the most commonly used approach. You can declare a boolean variable and set it to either true or false right at the moment of declaration.
boolean isJavaFun = true;
boolean isFishTasty = false;
In this example, we have declared two boolean variables: isJavaFun
and isFishTasty
. The first variable, isJavaFun
, is set to true, indicating that Java is indeed fun. The second variable, isFishTasty
, is assigned false, suggesting that fish may not be to everyone’s taste. This method is efficient for scenarios where you know the value of the boolean variable at the time of declaration.
Output:
isJavaFun: true
isFishTasty: false
By initialising boolean variables directly, you can quickly establish conditions that can be used later in your code. This approach is particularly useful in conditional statements where the boolean value determines the flow of execution.
Initialising a Boolean Variable Using Conditional Expressions
Another effective way to initialise a boolean variable in Java is by using conditional expressions. This method allows you to set the value of a boolean variable based on certain conditions, making your code more dynamic and responsive.
int a = 10;
int b = 20;
boolean isGreater = (a > b);
In this code snippet, we compare two integers, a
and b
. The boolean variable isGreater
is set to the result of the expression a > b
. Since 10 is not greater than 20, isGreater
will be assigned the value false. This technique is particularly useful when you need to evaluate conditions before setting a boolean value.
Output:
isGreater: false
Using conditional expressions to initialise boolean variables enhances the flexibility of your code. It allows you to create more complex logic based on the values of other variables, making your applications more interactive and intelligent.
Initialising a Boolean Variable with Method Return Values
You can also initialise a boolean variable with the return value of a method. This approach is beneficial when you need to encapsulate logic within a method and use its result to set the value of a boolean variable.
public class BooleanExample {
public static void main(String[] args) {
boolean isEven = isNumberEven(4);
}
public static boolean isNumberEven(int number) {
return number % 2 == 0;
}
}
In the example above, we have a method called isNumberEven
, which checks if a number is even. The boolean variable isEven
is initialised with the return value of this method when we pass the number 4. Since 4 is indeed even, isEven
will be set to true.
Output:
isEven: true
This method of initialising boolean variables is particularly useful in larger applications where you want to maintain clean and modular code. By using methods to encapsulate logic, you can improve code readability and reusability.
Initialising a Boolean Variable from User Input
Sometimes, you may want to initialise a boolean variable based on user input. This method allows your program to be interactive, responding to user decisions or preferences.
import java.util.Scanner;
public class UserInputExample {
public static void main(String[] args) {
Scanner scanner = new Scanner(System.in);
System.out.print("Do you like Java? (true/false): ");
boolean likesJava = scanner.nextBoolean();
System.out.println("User likes Java: " + likesJava);
}
}
In this code, we use the Scanner
class to capture user input. The user is prompted to enter either true or false, which is then stored in the boolean variable likesJava
. Depending on the user’s input, this variable will reflect their preference.
Output:
User likes Java: true (or false based on user input)
This method of initialising boolean variables is crucial for applications that require user interaction. It allows your program to adapt based on user preferences, enhancing the overall user experience.
Conclusion
Understanding how to initialise boolean variables in Java is a fundamental skill that every programmer should master. Whether you choose to assign values directly, use conditional expressions, capture method return values, or take user input, each method has its unique advantages. By employing these techniques, you can write cleaner, more efficient, and more interactive Java applications. So, go ahead and experiment with these methods in your projects, and watch your programming skills soar!
FAQ
-
What is a boolean variable in Java?
A boolean variable in Java is a data type that can hold one of two values: true or false. It is often used for conditional statements and logic. -
How do I declare a boolean variable in Java?
You can declare a boolean variable by using the keywordboolean
, followed by the variable name and an optional initial value, like this:boolean myVar = true;
. -
Can a boolean variable hold values other than true or false?
No, a boolean variable can only hold the values true or false. Any other value will result in a compilation error. -
How can I change the value of a boolean variable?
You can change the value of a boolean variable by simply reassigning it, like this:myVar = false;
. -
What are some common use cases for boolean variables in Java?
Boolean variables are commonly used in conditionals (if statements), loops (while and for loops), and flags to indicate the state of an object or process.
Rashmi is a professional Software Developer with hands on over varied tech stack. She has been working on Java, Springboot, Microservices, Typescript, MySQL, Graphql and more. She loves to spread knowledge via her writings. She is keen taking up new things and adopt in her career.
LinkedIn