How to Import Custom Class in Java
- Syntax to import class in Java
- Import custom Class in Java
- Import custom Class in Java
- Static Import Class in Java
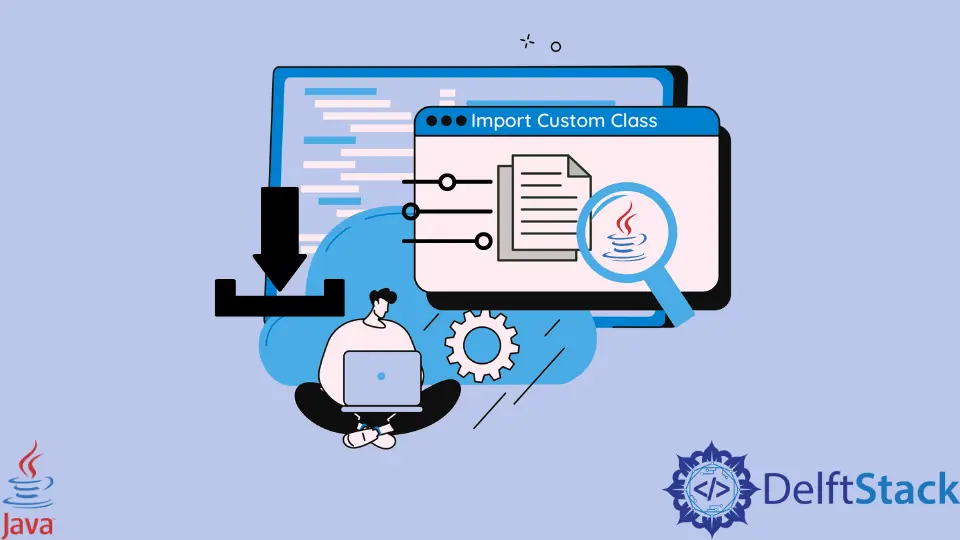
This tutorial introduces how to import the custom class in Java.
If the class is present in the same package, then use that by creating its object, and if the class is present in another package, then we should first import the package the use its methods and variables. Let’s take a close look at the examples.
Syntax to import class in Java
Below is the syntax to import a class and static members of the class in Java.
import static package.myclass; // static import
import package.myclass;
Import custom Class in Java
Let’s create a custom class (Student
) containing two instance variables and getter
and setter
methods. After that, import this class into the SimpleTesting
class by using the import statement. See the example below.
Student.java
package myjavaproject;
class Student {
String name;
String email;
public String getName() {
return name;
}
public void setName(String name) {
this.name = name;
}
public String getEmail() {
return email;
}
public void setEmail(String email) {
this.email = email;
}
}
SimpleTesting.java
package xyz;
import myjavaproject.Student;
public class SimpleTesting {
public static void main(String[] args) {
Student student = new Student();
student.setName("Rohna");
student.setEmail("rohna@xyz.com");
System.out.println(student.getName());
System.out.println(student.getEmail());
}
}
Output:
Rohna
rohna@xyz.com
Import custom Class in Java
If the class is in the same package or in the same file, then we don’t need to import that but simply use it by creating an object of this class. See the example below.
package myjavaproject;
public class SimpleTesting extends Thread {
public static void main(String[] args) {
Student student = new Student();
student.setName("Rohna");
student.setEmail("rohna@xyz.com");
System.out.println(student.getName());
System.out.println(student.getEmail());
}
}
Output:
Rohna
rohna@xyz.com
Static Import Class in Java
In case we only want to import static members of the class, we can use the static import concept. We need to use the static
keyword with the import
statement. See the example below.
import static java.lang.Math.*;
public class SimpleTesting {
public static void main(String[] args) {
int a = 10;
double b = ceil(a);
System.out.println(b);
}
}
Output:
10.0